Assert updated with the returned status code instead of URL
Dependencies: ublox-at-cellular-interface
UbloxATCellularInterfaceExt.h
00001 /* Copyright (c) 2017 ARM Limited 00002 * 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 */ 00015 00016 #ifndef _UBLOX_AT_CELLULAR_INTERFACE_EXT_ 00017 #define _UBLOX_AT_CELLULAR_INTERFACE_EXT_ 00018 00019 #include "UbloxATCellularInterface.h" 00020 #include "UbloxCellularDriverGen.h" 00021 00022 /**UbloxATCellularInterfaceExt class. 00023 * 00024 * This interface extends the UbloxATCellularInterface to 00025 * include other features that use the IP stack on board the 00026 * cellular modem: HTTP, FTP and Cell Locate. 00027 * 00028 * Note: the UbloxCellularGeneric class is required because 00029 * reading a large HTTP response is performed via a modem 00030 * file system call and the UbloxCellularGeneric class is 00031 * where modem file system support is provided. 00032 */ 00033 class UbloxATCellularInterfaceExt : public UbloxATCellularInterface, public UbloxCellularDriverGen { 00034 00035 public: 00036 /** Constructor. 00037 * 00038 * @param tx the UART TX data pin to which the modem is attached. 00039 * @param rx the UART RX data pin to which the modem is attached. 00040 * @param baud the UART baud rate. 00041 * @param debugOn true to switch AT interface debug on, otherwise false. 00042 */ 00043 UbloxATCellularInterfaceExt(PinName tx = MDMTXD, 00044 PinName rx = MDMRXD, 00045 int baud = MBED_CONF_UBLOX_CELL_BAUD_RATE, 00046 bool debugOn = false, 00047 osPriority priority = osPriorityNormal); 00048 00049 /* Destructor. 00050 */ 00051 virtual ~UbloxATCellularInterfaceExt(); 00052 00053 /********************************************************************** 00054 * PUBLIC: General 00055 **********************************************************************/ 00056 00057 /** Infinite timeout. 00058 */ 00059 #define TIMEOUT_BLOCKING -1 00060 00061 /** A struct containing an HTTP or FTP error class and code 00062 */ 00063 typedef struct { 00064 int eClass; 00065 int eCode; 00066 } Error; 00067 00068 /********************************************************************** 00069 * PUBLIC: HTTP 00070 **********************************************************************/ 00071 00072 /** HTTP profile unused marker. 00073 */ 00074 #define HTTP_PROF_UNUSED -1 00075 00076 /** HTTP configuration parameters (reference to HTTP control +UHTTP). 00077 */ 00078 typedef enum { 00079 HTTP_IP_ADDRESS = 0, 00080 HTTP_SERVER_NAME = 1, 00081 HTTP_USER_NAME = 2, 00082 HTTP_PASSWORD = 3, 00083 HTTP_AUTH_TYPE = 4, 00084 HTTP_SERVER_PORT = 5, 00085 HTTP_SECURE = 6 00086 } HttpOpCode; 00087 00088 /** Type of HTTP Command. 00089 */ 00090 typedef enum { 00091 HTTP_HEAD = 0, 00092 HTTP_GET = 1, 00093 HTTP_DELETE = 2, 00094 HTTP_PUT = 3, 00095 HTTP_POST_FILE = 4, 00096 HTTP_POST_DATA = 5 00097 } HttpCmd; 00098 00099 /** HTTP content types. 00100 */ 00101 typedef enum { 00102 HTTP_CONTENT_URLENCODED = 0, 00103 HTTP_CONTENT_TEXT = 1, 00104 HTTP_CONTENT_OCTET_STREAM = 2, 00105 HTTP_CONTENT_FORM_DATA = 3, 00106 HTTP_CONTENT_JSON = 4, 00107 HTTP_CONTENT_XML = 5, 00108 HTTP_CONTENT_USER_DEFINED = 6 00109 } HttpContentType; 00110 00111 /** Find a free HTTP profile. 00112 * 00113 * A profile will be blocking when first allocated. 00114 * 00115 * @return the profile or negative if none are available. 00116 */ 00117 int httpAllocProfile(); 00118 00119 /** Free the HTTP profile. 00120 * 00121 * @param profile the HTTP profile handle. 00122 * @return true if successful, otherwise false. 00123 */ 00124 bool httpFreeProfile(int profile); 00125 00126 /** Set the timeout for this profile. 00127 * 00128 * @param profile the HTTP profile handle. 00129 * @param timeout -1 blocking, else non-blocking timeout in milliseconds. 00130 * @return true if successful, otherwise false. 00131 */ 00132 bool httpSetTimeout(int profile, int timeout); 00133 00134 /** Reset a HTTP profile back to defaults. 00135 * 00136 * This may be called if the state of a HTTP profile 00137 * during parameter setting or exchange of HTTP commands 00138 * has become confusing/unknown. 00139 * 00140 * @param httpProfile the HTTP profile to be reset. 00141 * @return true if successful, false otherwise. 00142 */ 00143 bool httpResetProfile(int httpProfile); 00144 00145 /** Set HTTP parameters. 00146 * 00147 * This should be called as many times as is necessary 00148 * to set all the possible parameters (HttpOpCode). 00149 * 00150 * See section 28.1 of u-blox-ATCommands_Manual(UBX-13002752).pdf 00151 * for full details. By example: 00152 * 00153 * httpOpCode httpInPar 00154 * HTTP_IP_ADDRESS "145.33.18.10" (the target HTTP server IP address) 00155 * HTTP_SERVER_NAME "www.myhttpserver.com" (the target HTTP server name) 00156 * HTTP_USER_NAME "my_username" 00157 * HTTP_PASSWORD "my_password" 00158 * HTTP_AUTH_TYPE "0" for no authentication, "1" for username/password 00159 * authentication (the default is 0) 00160 * HTTP_SERVER_PORT "81" (default is port 80) 00161 * HTTP_SECURE "0" for no security, "1" for TLS (the default is 0), 00162 * not all modems support this parameter 00163 * 00164 * @param httpProfile the HTTP profile identifier. 00165 * @param httpOpCode the HTTP operation code. 00166 * @param httpInPar the HTTP input parameter. 00167 * @return true if successful, false otherwise. 00168 */ 00169 bool httpSetPar(int httpProfile, HttpOpCode httpOpCode, const char * httpInPar); 00170 00171 /** Perform a HTTP command. 00172 * 00173 * See section 28.3 of u-blox-ATCommands_Manual(UBX-13002752).pdf 00174 * for full details. By example, it works like this: 00175 * 00176 * httpCmd httpPath rspFile sendStr httpContentType httpCustomPar 00177 * HEAD "path/file.html" NULL NULL 0 NULL 00178 * GET "path/file.html" NULL NULL 0 NULL 00179 * DELETE "path/file.html" NULL NULL 0 NULL 00180 * PUT "path/file.html" NULL "myfile.txt" 0 to 6 Note 1 00181 * POST_FILE "path/file.html" NULL "myfile.txt" 0 to 6 Note 1 00182 * POST "path/file.html" NULL "hello there!" 0 to 6 Note 1 00183 * 00184 * Note 1: httpCustomPar is only applicable when httpContentType = HTTP_CONTENT_USER_DEFINED. 00185 * 00186 * The server to which this command is directed must have previously been 00187 * set with a call to httpSetPar(). If the server requires TLS (i.e. "HTTPS"), 00188 * then set that up with httpSetPar() also (HTTP_SECURE). 00189 * 00190 * rspFile may be left as NULL as the server response will be returned in buf. 00191 * Alternatively, a rspFile may be given (e.g. "myresponse.txt") and this can 00192 * later be read from the modem file system using readFile(). 00193 * 00194 * @param httpProfile the HTTP profile identifier. 00195 * @param httpCmd the HTTP command. 00196 * @param httpPath the path of resource on the HTTP server. 00197 * @param rspFile the local modem file where the server 00198 * response will be stored, use NULL for 00199 * don't care. 00200 * @param sendStr the filename or string to be sent 00201 * to the HTTP server with the command request. 00202 * @param httpContentType the HTTP Content-Type identifier. 00203 * @param httpCustomPar the parameter for a user defined HTTP Content-Type. 00204 * @param buf the buffer to read into. 00205 * @param len the size of the buffer to read into. 00206 * @param read_size Zero initialized variable address 00207 * @return NULL if successful, otherwise a pointer to 00208 * a Error struct containing the error class and error 00209 * code, see section Appendix A.B of 00210 * u-blox-ATCommands_Manual(UBX-13002752).pdf for details. 00211 */ 00212 Error * httpCommand(int httpProfile, HttpCmd httpCmd, const char* httpPath, 00213 const char* rspFile, const char* sendStr, 00214 int httpContentType, const char* httpCustomPar, 00215 char* buf, int len, int *read_size = NULL); 00216 00217 /********************************************************************** 00218 * PUBLIC: FTP 00219 **********************************************************************/ 00220 00221 /** FTP configuration parameters (reference to FTP control +UFTP). 00222 */ 00223 typedef enum { 00224 FTP_IP_ADDRESS = 0, 00225 FTP_SERVER_NAME = 1, 00226 FTP_USER_NAME = 2, 00227 FTP_PASSWORD = 3, 00228 FTP_ACCOUNT = 4, 00229 FTP_INACTIVITY_TIMEOUT = 5, 00230 FTP_MODE = 6, 00231 FTP_SERVER_PORT = 7, 00232 FTP_SECURE = 8, 00233 NUM_FTP_OP_CODES 00234 } FtpOpCode; 00235 00236 /** Type of FTP Command. 00237 */ 00238 typedef enum { 00239 FTP_LOGOUT = 0, 00240 FTP_LOGIN = 1, 00241 FTP_DELETE_FILE = 2, 00242 FTP_RENAME_FILE = 3, 00243 FTP_GET_FILE = 4, 00244 FTP_PUT_FILE = 5, 00245 FTP_GET_DIRECT = 6, 00246 FTP_PUT_DIRECT = 7, 00247 FTP_CD = 8, 00248 FTP_MKDIR = 10, 00249 FTP_RMDIR = 11, 00250 FTP_FILE_INFO = 13, 00251 FTP_LS = 14, 00252 FTP_FOTA_FILE = 100 00253 } FtpCmd; 00254 00255 /** Set the timeout for FTP operations. 00256 * 00257 * @param timeout -1 blocking, else non-blocking timeout in milliseconds. 00258 * @return true if successful, otherwise false. 00259 */ 00260 bool ftpSetTimeout(int timeout); 00261 00262 /** Reset the FTP configuration back to defaults. 00263 */ 00264 void ftpResetPar(); 00265 00266 /** Set FTP parameters. 00267 * 00268 * This should be called as many times as is necessary 00269 * to set all the possible parameters (FtpOpCode). 00270 * 00271 * See section 27.1 of u-blox-ATCommands_Manual(UBX-13002752).pdf 00272 * for full details. By example: 00273 * 00274 * ftpOpCode ftpInPar 00275 * FTP_IP_ADDRESS "145.33.18.10" (the target FTP server IP address) 00276 * FTP_SERVER_NAME "www.ftpserver.com" (the target FTP server name) 00277 * FTP_USER_NAME "my_username" 00278 * FTP_PASSWORD "my_password" 00279 * FTP_ACCOUNT "my_account" (not required by most FTP servers) 00280 * FTP_INACTIVITY_TIMEOUT "60" (the default is 0, which means no timeout) 00281 * FTP_MODE "0" for active, "1" for passive (the default is 0) 00282 * FTP_SERVER_PORT "25" (default is port 21) 00283 * FTP_SECURE "0" for no security, "1" for SFTP (the default is 0) 00284 * 00285 * @param ftpOpCode the FTP operation code. 00286 * @param ftpInPar the FTP input parameter. 00287 * @return true if successful, false otherwise. 00288 */ 00289 bool ftpSetPar(FtpOpCode ftpOpCode, const char * ftpInPar); 00290 00291 /** Perform an FTP command. 00292 * 00293 * Connect() must have been called previously to establish a data 00294 * connection. 00295 * 00296 * See section 27.2 of u-blox-ATCommands_Manual(UBX-13002752).pdf 00297 * for full details. By example, it works like this: 00298 * 00299 * ftpCmd file1 file2 buf len offset 00300 * FTP_LOGOUT N/A N/A N/A N/A N/A 00301 * FTP_LOGIN N/A N/A N/A N/A N/A 00302 * FTP_DELETE_FILE "the_file" N/A N/A N/A N/A 00303 * FTP_RENAME_FILE "old_name" "new_name" N/A N/A N/A 00304 * FTP_GET_FILE "the_file" Note 1 N/A N/A 0 - 1 (Notes 2 & 3) 00305 * FTP_PUT_FILE "the_file" Note 1 N/A N/A 0 - 65535 (Notes 2 & 4 & 5) 00306 * FTP_CD "dir1\dir2" N/A N/A N/A N/A 00307 * FTP_MKDIR "newdir" N/A N/A N/A N/A 00308 * FTP_RMDIR "dir" N/A N/A N/A N/A 00309 * FTP_FILE_INFO "the_path" N/A Note 6 N/A 00310 * FTP_LS "the_path" N/A Note 6 N/A 00311 * FTP_FOTA_FILE "the_file" N/A Note 7 N/A 00312 * 00313 * Note 1: for this case, file2 is the name that the file should be 00314 * given when it arrives (in the modem file system for a GET, at the FTP 00315 * server for a PUT); if set to NULL then file1 is used. 00316 * Note 2: the file will placed into the modem file system for the 00317 * GET case (and can be read with readFile()), or must already be in the 00318 * modem file system, (can be written using writeFile()) for the PUT case. 00319 * Note 3: if offset is 1 then, where supported, the FTP GET 00320 * will be continued from the point it previously stopped. 00321 * Note 4: if the file already exists in the modem file system some 00322 * modems will return an error. It is up to the caller to ensure that 00323 * the file does not exist before the FTP PUT operation. 00324 * Note 5: where supported, offset is the position in the file to continue 00325 * the FTP PUT from. 00326 * Note 6: buf should point to the location where the file info 00327 * or directory listing is to be stored and len should be the maximum 00328 * length that can be stored. 00329 * Note 7: a hex string representing the MD5 sum of the FOTA file will be 00330 * stored at buf; len must be at least 32 as an MD5 sum is 16 bytes. 00331 * FTP_FOTA_FILE is not supported on SARA-U2. 00332 * Note 8: FTP_GET_DIRECT and FTP_PUT_DIRECT are not supported by 00333 * this driver. 00334 * 00335 * @param ftpCmd the FTP command. 00336 * @param file1 the first file name if required (NULL otherwise). 00337 * @param file2 the second file name if required (NULL otherwise). 00338 * @param buf pointer to a buffer, required for FTP_DIRECT mode 00339 * and FTP_LS only. 00340 * @param len the size of buf. 00341 * @param continue if true then attempt to continue a download that 00342 * was previously interrupted. 00343 * @return NULL if successful, otherwise a pointer to 00344 * a Error struct containing the error class and error 00345 * code, see section Appendix A.B of 00346 * u-blox-ATCommands_Manual(UBX-13002752).pdf for details. 00347 */ 00348 Error *ftpCommand(FtpCmd ftpCmd, const char* file1 = NULL, const char* file2 = NULL, 00349 char* buf = NULL, int len = 0, int offset = 0); 00350 00351 /********************************************************************** 00352 * PUBLIC: Cell Locate 00353 **********************************************************************/ 00354 00355 /** Which form of Cell Locate sensing to use. 00356 */ 00357 typedef enum { 00358 CELL_LAST, 00359 CELL_GNSS, 00360 CELL_LOCATE, 00361 CELL_HYBRID 00362 } CellSensType; 00363 00364 /** Types of Cell Locate response. 00365 */ 00366 typedef enum { 00367 CELL_DETAILED = 1, 00368 CELL_MULTIHYP = 2 00369 } CellRespType; 00370 00371 /** Cell Locate data. 00372 */ 00373 typedef struct { 00374 volatile bool validData; //!< Flag for indicating if data is valid. 00375 volatile struct tm time; //!< GPS Timestamp. 00376 volatile float longitude; //!< Estimated longitude, in degrees. 00377 volatile float latitude; //!< Estimated latitude, in degrees. 00378 volatile int altitude; //!< Estimated altitude, in meters^2. 00379 volatile int uncertainty; //!< Maximum possible error, in meters. 00380 volatile int speed; //!< Speed over ground m/s^2. 00381 volatile int direction; //!< Course over ground in degrees. 00382 volatile int verticalAcc; //!< Vertical accuracy, in meters^2. 00383 volatile CellSensType sensor; //!< Sensor used for last calculation. 00384 volatile int svUsed; //!< number of satellite used. 00385 } CellLocData; 00386 00387 /** Configure the Cell Locate TCP aiding server. 00388 * 00389 * Connect() must have been called previously to establish 00390 * a data connection. 00391 * 00392 * @param server_1 host name of the primary MGA server. 00393 * @param server_2 host name of the secondary MGA server. 00394 * @param token authentication token for MGA server access. 00395 * @param days the number of days into the future the off-line 00396 * data for the u-blox 7. 00397 * @param period the number of weeks into the future the off-line 00398 * data for u-blox M8. 00399 * @param resolution resolution of off-line data for u-blox M8: 1 every 00400 * day, 0 every other day. 00401 * @return true if the request is successful, otherwise false. 00402 */ 00403 bool cellLocSrvTcp(const char* token, const char* server_1 = "cell-live1.services.u-blox.com", 00404 const char* server_2 = "cell-live2.services.u-blox.com", 00405 int days = 14, int period = 4, int resolution = 1); 00406 00407 /** Configure Cell Locate UDP aiding server. 00408 * 00409 * Connect() must have been called previously to establish 00410 * a data connection. 00411 * 00412 * @param server_1 host name of the primary MGA server. 00413 * @param port server port. 00414 * @param latency expected network latency in seconds from 0 to 10000 milliseconds. 00415 * @param mode Assist Now management, mode of operation: 00416 * 0 data downloaded at GNSS power up, 00417 * 1 automatically kept alive, manual download. 00418 * @return true if the request is successful, otherwise false. 00419 */ 00420 bool cellLocSrvUdp(const char* server_1 = "cell-live1.services.u-blox.com", 00421 int port = 46434, int latency = 1000, int mode = 0); 00422 00423 /** Configure Cell Locate location sensor. 00424 * 00425 * @param scanMode network scan mode: 0 normal, 1 deep scan. 00426 * @return true if the request is successful, otherwise false. 00427 */ 00428 bool cellLocConfig(int scanMode); 00429 00430 /** Request a one-shot Cell Locate. 00431 * 00432 * This function is non-blocking, the result is retrieved using cellLocGetxxx. 00433 * 00434 * Note: none of the CellLocate methods switch on the GNSS receiver chip. 00435 * That should be done by instantiating the GnssSerial or GnssI2C classes and 00436 * calling the init() method. 00437 * 00438 * Note: during the location process, unsolicited result codes will be returned 00439 * by the modem indicating progress and potentially flagging interesting errors. 00440 * In order to see these errors, instantiate this class with debugOn set to true. 00441 * 00442 * @param sensor sensor selection. 00443 * @param timeout timeout period in seconds (1 - 999). 00444 * @param accuracy target accuracy in meters (1 - 999999). 00445 * @param type detailed or multi-hypothesis. 00446 * @param hypothesis maximum desired number of responses from CellLocate (up to 16), 00447 * must be 1 if type is CELL_DETAILED. 00448 * @return true if the request is successful, otherwise false. 00449 */ 00450 bool cellLocRequest(CellSensType sensor, int timeout, int accuracy, 00451 CellRespType type = CELL_DETAILED, int hypothesis = 1); 00452 00453 /** Get a position record. 00454 * 00455 * @param data pointer to a CellLocData structure where the location will be put. 00456 * @param index of the position to retrieve. 00457 * @return true if data has been retrieved and copied, false otherwise. 00458 */ 00459 bool cellLocGetData(CellLocData *data, int index = 0); 00460 00461 /** Get the number of position records received. 00462 * 00463 * @return number of position records received. 00464 */ 00465 int cellLocGetRes(); 00466 00467 /** Get the number of position records expected to be received. 00468 * 00469 * @return number of position records expected to be received. 00470 */ 00471 int cellLocGetExpRes(); 00472 00473 protected: 00474 00475 /********************************************************************** 00476 * PROTECTED: HTTP 00477 **********************************************************************/ 00478 00479 /** Check for timeout. 00480 */ 00481 #define TIMEOUT(t, ms) ((ms != TIMEOUT_BLOCKING) && (ms < t.read_ms())) 00482 00483 /** Check for a valid profile. 00484 */ 00485 #define IS_PROFILE(p) (((p) >= 0) && (((unsigned int) p) < (sizeof(_httpProfiles)/sizeof(_httpProfiles[0]))) \ 00486 && (_httpProfiles[p].modemHandle != HTTP_PROF_UNUSED)) 00487 00488 /** Management structure for HTTP profiles. 00489 * 00490 * It is possible to have up to 4 different HTTP profiles (LISA-C200, LISA-U200 and SARA-G350) having: 00491 * 00492 * @param handle the current HTTP profile is in handling state or not (default value is HTTP_ERROR). 00493 * @param timeout the timeout for the current HTTP command. 00494 * @param pending the status for the current HTTP command (in processing state or not). 00495 * @param cmd the code for the current HTTP command. 00496 * @param result the result for the current HTTP command once processed. 00497 */ 00498 typedef struct { 00499 int modemHandle; 00500 int timeout; 00501 volatile bool pending; 00502 volatile int cmd; 00503 volatile int result; 00504 Error httpError; 00505 } HttpProfCtrl; 00506 00507 /** The HTTP profile storage. 00508 */ 00509 HttpProfCtrl _httpProfiles[4]; 00510 00511 /** Callback to capture the response to an HTTP command. 00512 */ 00513 void UUHTTPCR_URC(); 00514 00515 /** Find a profile with a given handle. If no handle is given, find the next 00516 * free profile. 00517 * 00518 * @param modemHandle the handle of the profile to find. 00519 * @return the profile handle or negative if not found/created. 00520 */ 00521 int findProfile(int modemHandle = HTTP_PROF_UNUSED); 00522 00523 /** Helper function to get a HTTP command as a text string, useful 00524 * for debug purposes. 00525 * 00526 * @param httpCmdCode the HTTP command. 00527 * @return HTTP command in string format. 00528 */ 00529 const char* getHttpCmd(HttpCmd httpCmd); 00530 00531 /********************************************************************** 00532 * PROTECTED: FTP 00533 **********************************************************************/ 00534 00535 /** Unused FTP op code marker. 00536 */ 00537 #define FTP_OP_CODE_UNUSED -1 00538 00539 /** The FTP timeout in milliseconds. 00540 */ 00541 int _ftpTimeout; 00542 00543 /** A place to store the FTP op code for the last result. 00544 */ 00545 volatile int _lastFtpOpCodeResult; 00546 00547 /** A place to store the last FTP result. 00548 */ 00549 volatile int _lastFtpResult; 00550 00551 /** A place to store the last FTP op code for data response. 00552 */ 00553 volatile int _lastFtpOpCodeData; 00554 00555 /** A place to store data returns from an FTP operation. 00556 */ 00557 char * _ftpBuf; 00558 00559 /** The length of FTP data that can be stored (at _ftpBuf). 00560 */ 00561 int _ftpBufLen; 00562 00563 /** storage for the last FTP error 00564 */ 00565 Error _ftpError; 00566 00567 /** Callback to capture the result of an FTP command. 00568 */ 00569 void UUFTPCR_URC(); 00570 00571 /** Callback to capture data returned from an FTP command. 00572 */ 00573 void UUFTPCD_URC(); 00574 00575 /** Helper function to get an FTP command as a text string, useful 00576 * for debug purposes. 00577 * 00578 * @param ftpCmdCode the FTP command. 00579 * @return FTP command in string format. 00580 */ 00581 const char * getFtpCmd(FtpCmd ftpCmd); 00582 00583 /********************************************************************** 00584 * PROTECTED: Cell Locate 00585 **********************************************************************/ 00586 00587 /** The maximum number of hypotheses 00588 */ 00589 #define CELL_MAX_HYP (16 + 1) 00590 00591 /** Received positions. 00592 */ 00593 volatile int _locRcvPos; 00594 00595 /** Expected positions. 00596 */ 00597 volatile int _locExpPos; 00598 00599 /** The Cell Locate data. 00600 */ 00601 CellLocData _loc[CELL_MAX_HYP]; 00602 00603 /** Buffer for the URC to work with 00604 */ 00605 char urcBuf[128]; 00606 00607 /** Callback to capture +UULOCIND. 00608 */ 00609 void UULOCIND_URC(); 00610 00611 /** Callback to capture +UULOC. 00612 */ 00613 void UULOC_URC(); 00614 }; 00615 00616 #endif // _UBLOX_AT_CELLULAR_INTERFACE_EXT_ 00617
Generated on Fri Jul 15 2022 22:48:29 by
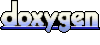