
USBMIDI example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Hello World example for the USBMIDI library 00002 00003 #include "mbed.h" 00004 #include "USBMIDI.h" 00005 00006 //USBMIDI object 00007 USBMIDI midi; 00008 00009 00010 // Leds which will be switch on or off according to a MIDImessage 00011 BusOut leds(LED1, LED2, LED3, LED4); 00012 00013 BusInOut buttons(p22, p23, p24, p25); 00014 00015 void show_message(MIDIMessage msg) { 00016 switch (msg.type()) { 00017 case MIDIMessage::NoteOnType: 00018 switch (msg.key()) { 00019 case 48: 00020 leds = (1 << 0); 00021 break; 00022 case 49: 00023 leds = (1 << 1); 00024 break; 00025 case 50: 00026 leds = (1 << 2); 00027 break; 00028 case 51: 00029 leds = (1 << 3); 00030 break; 00031 } 00032 break; 00033 case MIDIMessage::NoteOffType: 00034 default: 00035 leds = 0; 00036 } 00037 } 00038 00039 int main() { 00040 uint8_t bus = 0; 00041 uint8_t p_bus = 0; 00042 00043 // call back for messages received 00044 midi.attach(show_message); 00045 00046 while (1) { 00047 00048 //if buttons state changes, send a MIDI message 00049 bus = buttons.read(); 00050 for (int i = 0; i < 4; i++) { 00051 if ( (bus & (1 << i)) != (p_bus & (1 << i))) { 00052 if (bus & (1 << i)) { 00053 midi.write(MIDIMessage::NoteOn(48 + i)); 00054 } else if ( !(bus & (1 << i)) ) { 00055 midi.write(MIDIMessage::NoteOff(48 + i)); 00056 } 00057 00058 } 00059 } 00060 wait(0.001); 00061 p_bus = bus; 00062 } 00063 }
Generated on Tue Jul 12 2022 18:00:23 by
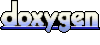