
USBHostHub Hello World
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "USBHostKeyboard.h" 00003 #include "USBHostMouse.h" 00004 00005 DigitalOut led(LED1); 00006 00007 void onKey(uint8_t key) { 00008 printf("Key: %c\r\n", key); 00009 } 00010 00011 void onMouseEvent(uint8_t buttons, int8_t x, int8_t y, int8_t z) { 00012 printf("buttons: %d, x: %d, y: %d, z: %d\r\n", buttons, x, y, z); 00013 } 00014 00015 void keyboard_task(void const *) { 00016 00017 USBHostKeyboard keyboard; 00018 00019 while(1) { 00020 // try to connect a USB keyboard 00021 while(!keyboard.connect()) 00022 Thread::wait(500); 00023 00024 // when connected, attach handler called on keyboard event 00025 keyboard.attach(onKey); 00026 00027 // wait until the keyboard is disconnected 00028 while(keyboard.connected()) 00029 Thread::wait(500); 00030 } 00031 } 00032 00033 void mouse_task(void const *) { 00034 00035 USBHostMouse mouse; 00036 00037 while(1) { 00038 // try to connect a USB mouse 00039 while(!mouse.connect()) 00040 Thread::wait(500); 00041 00042 // when connected, attach handler called on mouse event 00043 mouse.attachEvent(onMouseEvent); 00044 00045 // wait until the mouse is disconnected 00046 while(mouse.connected()) 00047 Thread::wait(500); 00048 } 00049 } 00050 00051 int main() { 00052 Thread keyboardTask(keyboard_task, NULL, osPriorityNormal, 256 * 4); 00053 Thread mouseTask(mouse_task, NULL, osPriorityNormal, 256 * 4); 00054 while(1) { 00055 led=!led; 00056 Thread::wait(500); 00057 } 00058 }
Generated on Tue Jul 12 2022 20:54:55 by
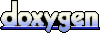