
RPC hello world using the Wifly Interface
Dependencies: MbedJSONRpc MbedJSONValue WebSocketClient WiflyInterface mbed
main.cpp
00001 #include "mbed.h" 00002 #include "WiflyInterface.h" 00003 #include "Websocket.h" 00004 #include "MbedJSONRpc.h" 00005 00006 BusOut l(LED1, LED2, LED3, LED4); 00007 00008 /* wifly interface: 00009 * - p9 and p10 are for the serial communication 00010 * - p19 is for the reset pin 00011 * - p26 is for the connection status 00012 * - "mbed" is the ssid of the network 00013 * - "password" is the password 00014 * - WPA is the security 00015 */ 00016 WiflyInterface wifly(p9, p10, p19, p26, "mbed", "password", WPA); 00017 00018 //websocket: configuration with sub-network = demo and mbed_id = mbed_led 00019 Websocket ws("ws://sockets.mbed.org/rpc/demo/mbed_led"); 00020 00021 //RPC object attached to the websocket server 00022 MbedJSONRpc rpc(&ws); 00023 00024 //Test class 00025 class Test { 00026 public: 00027 Test() {}; 00028 void led(MbedJSONValue& in, MbedJSONValue& out) { 00029 int id = in[0].get<int>(); 00030 l = (1 << (id - 1)); 00031 wait(0.2); 00032 l = 0; 00033 } 00034 }; 00035 00036 Test test; 00037 00038 int main() { 00039 00040 wifly.init(); //Use DHCP 00041 wifly.connect(); 00042 printf("IP Address is %s\n\r", wifly.getIPAddress()); 00043 00044 ws.connect(); 00045 00046 RPC_TYPE t; 00047 00048 //------------register led---------------// 00049 if((t = rpc.registerMethod("led", &test, &Test::led)) == REGISTER_OK) 00050 printf("led is registered\r\n"); 00051 else 00052 printType(t); 00053 00054 //wait for incoming CALL requests 00055 rpc.work(); 00056 }
Generated on Sat Jul 16 2022 22:47:52 by
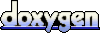