
HTTPClient Hello World with the WiflyInterface
Dependencies: HTTPClient WiflyInterface mbed
main.cpp
00001 #include "mbed.h" 00002 #include "WiflyInterface.h" 00003 #include "HTTPClient.h" 00004 00005 /* wifly interface: 00006 * - p9 and p10 are for the serial communication 00007 * - p19 is for the reset pin 00008 * - p26 is for the connection status 00009 * - "mbed" is the ssid of the network 00010 * - "password" is the password 00011 * - WPA is the security 00012 */ 00013 WiflyInterface wifly(p9, p10, p19, p26, "mbed", "password", WPA); 00014 00015 HTTPClient http; 00016 char str[512]; 00017 00018 int main() 00019 { 00020 wifly.init(); //Use DHCP 00021 00022 wifly.connect(); 00023 00024 //GET data 00025 printf("Trying to fetch page...\n"); 00026 int ret = http.get("http://mbed.org/media/uploads/donatien/hello.txt", str, 128); 00027 if (!ret) { 00028 printf("Page fetched successfully - read %d characters\n", strlen(str)); 00029 printf("Result: %s\n", str); 00030 } else { 00031 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00032 } 00033 00034 //POST data 00035 HTTPMap map; 00036 HTTPText text(str, 512); 00037 map.put("Hello", "World"); 00038 map.put("test", "1234"); 00039 printf("Trying to post data...\n"); 00040 ret = http.post("http://httpbin.org/post", map, &text); 00041 if (!ret) { 00042 printf("Executed POST successfully - read %d characters\n", strlen(str)); 00043 printf("Result: %s\n", str); 00044 } else { 00045 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00046 } 00047 00048 wifly.disconnect(); 00049 00050 while(1) { 00051 } 00052 }
Generated on Fri Jul 22 2022 03:45:35 by
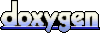