Utility functions for working with data streams
Embed:
(wiki syntax)
Show/hide line numbers
checksum.h
Go to the documentation of this file.
00001 /** 00002 * @file checksum.h 00003 * @brief Utility Function - Data integrity helpers 00004 * @author sam grove 00005 * @version 1.0 00006 * 00007 * Copyright (c) 2013 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); 00010 * you may not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, 00017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 */ 00021 00022 #ifndef CHECKSUM_H 00023 #define CHECKSUM_H 00024 00025 #include <stdint.h> 00026 00027 /** Helpers for working data streams 00028 * 00029 * Example: 00030 * @code 00031 * #include "mbed.h" 00032 * #include "checksum.h" 00033 * 00034 * DigitalOut myled(LED1); 00035 * 00036 * template <int T> 00037 * struct data{ 00038 * uint8_t buf[T]; 00039 * uint8_t checksum; 00040 * }; 00041 * data<12> all_ones = {{1,1,1,1,1,1,1,1,1,1,1,1}}; 00042 * 00043 * int main() 00044 * { 00045 * calculateChecksum( all_ones.buf, sizeof(all_ones) ); 00046 * 00047 * for(int i=0; i<sizeof(all_ones); i++) 00048 * { 00049 * printf("%02d: %d\n", i, *(all_ones.buf+i)); 00050 * } 00051 * 00052 * printf("checksum test: %s\n", (result)?"passed":"failed"); 00053 * 00054 * while(1) 00055 * { 00056 * myled = 1; 00057 * wait(0.2); 00058 * myled = 0; 00059 * wait(0.2); 00060 * } 00061 * } 00062 * @endcode 00063 */ 00064 00065 /** Calculate the checksum of a data stream 00066 * Assumes the checksum is in the last position, 8-bits and 0 - the sum of data 00067 * @param pkt - A pointer to the data 00068 * @param length - The amount of data that the checksum calculates 00069 * @returns true if the checksum is correct and false otherwise 00070 */ 00071 bool validateChecksum( uint8_t const *pkt, uint32_t const length ); 00072 00073 /** Calculate and store the checksum into the last position of a data stream 00074 * 00075 * @param pkt - A pointer to the data 00076 * @param length - The amount of data that the checksum calculates 00077 */ 00078 void calculateChecksum( uint8_t *pkt, uint32_t const length ); 00079 00080 #endif
Generated on Wed Jul 13 2022 03:02:51 by
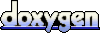