
CANfestival - an open source CANopen framework
Embed:
(wiki syntax)
Show/hide line numbers
nmtMaster.h
00001 /* 00002 This file is part of CanFestival, a library implementing CanOpen Stack. 00003 00004 Copyright (C): Edouard TISSERANT and Francis DUPIN 00005 00006 See COPYING file for copyrights details. 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00021 */ 00022 00023 /** @defgroup networkmanagement Network Management 00024 * @ingroup userapi 00025 */ 00026 /** @defgroup nmtmaster NMT Master 00027 * @brief NMT master provides mechanisms that control and monitor the state of nodes and their behavior in the network. 00028 * @ingroup networkmanagement 00029 */ 00030 00031 #ifndef __nmtMaster_h__ 00032 #define __nmtMaster_h__ 00033 00034 #include "data.h" 00035 00036 #ifdef __cplusplus 00037 extern "C"{ 00038 #endif 00039 00040 /** 00041 * @ingroup nmtmaster 00042 * @brief Transmit a NMT message on the network to the slave whose nodeId is node ID. 00043 * 00044 * @param *d Pointer to a CAN object data structure 00045 * @param nodeId Id of the slave node 00046 * @param cs The order of state changement \n\n 00047 * 00048 * Allowed states : 00049 * - cs = NMT_Start_Node // Put the node in operational mode 00050 * - cs = NMT_Stop_Node // Put the node in stopped mode 00051 * - cs = NMT_Enter_PreOperational // Put the node in pre_operational mode 00052 * - cs = NMT_Reset_Node // Put the node in initialization mode 00053 * - cs = NMT_Reset_Comunication // Put the node in initialization mode 00054 * 00055 * The mode is changed according to the slave state machine mode : 00056 * - initialisation ---> pre-operational (Automatic transition) 00057 * - pre-operational <--> operational 00058 * - pre-operational <--> stopped 00059 * - pre-operational, operational, stopped -> initialisation 00060 * \n\n 00061 * @return errorcode 00062 * - 0 if the NMT message was send 00063 * - 1 if an error occurs 00064 */ 00065 UNS8 masterSendNMTstateChange (CO_Data* d, UNS8 nodeId, UNS8 cs); 00066 00067 /** 00068 * @ingroup nmtmaster 00069 * @brief Transmit a NodeGuard message on the network to the slave whose nodeId is node ID 00070 * 00071 * @param *d Pointer to a CAN object data structure 00072 * @param nodeId Id of the slave node 00073 * @return 00074 * - 0 is returned if the NodeGuard message was send. 00075 * - 1 is returned if an error occurs. 00076 */ 00077 UNS8 masterSendNMTnodeguard (CO_Data* d, UNS8 nodeId); 00078 00079 /** 00080 * @ingroup nmtmaster 00081 * @brief Ask the state of the slave node whose nodeId is node Id. 00082 * 00083 * To ask states of all nodes on the network (NMT broadcast), nodeId must be equal to 0 00084 * @param *d Pointer to a CAN object data structure 00085 * @param nodeId Id of the slave node 00086 */ 00087 UNS8 masterRequestNodeState (CO_Data* d, UNS8 nodeId); 00088 00089 #ifdef __cplusplus 00090 } 00091 #endif 00092 00093 #endif /* __nmtMaster_h__ */
Generated on Tue Jul 12 2022 17:11:51 by
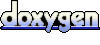