
CANfestival - an open source CANopen framework
Embed:
(wiki syntax)
Show/hide line numbers
canopen_timer.h
00001 /* 00002 This file is part of CanFestival, a library implementing CanOpen Stack. 00003 00004 Copyright (C): Edouard TISSERANT and Francis DUPIN 00005 00006 See COPYING file for copyrights details. 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00021 */ 00022 00023 #ifndef canopen_timer_h 00024 #define canopen_timer_h 00025 00026 #include <timerscfg.h> 00027 #include <applicfg.h> 00028 00029 #define TIMER_HANDLE INTEGER16 00030 00031 #include "data.h" 00032 00033 #ifdef __cplusplus 00034 extern "C"{ 00035 #endif 00036 00037 /* --------- types and constants definitions --------- */ 00038 #define TIMER_FREE 0 00039 #define TIMER_ARMED 1 00040 #define TIMER_TRIG 2 00041 #define TIMER_TRIG_PERIOD 3 00042 00043 #define TIMER_NONE -1 00044 00045 typedef void (*TimerCallback_t)(CO_Data* d, UNS32 id); 00046 00047 struct struct_s_timer_entry { 00048 UNS8 state; 00049 CO_Data* d; 00050 TimerCallback_t callback; /* The callback func. */ 00051 UNS32 id; /* The callback func. */ 00052 TIMEVAL val; 00053 TIMEVAL interval; /* Periodicity */ 00054 }; 00055 00056 typedef struct struct_s_timer_entry s_timer_entry; 00057 00058 /* --------- prototypes --------- */ 00059 /*#define SetAlarm(d, id, callback, value, period) printf("%s, %d, SetAlarm(%s, %s, %s, %s, %s)\n",__FILE__, __LINE__, #d, #id, #callback, #value, #period); _SetAlarm(d, id, callback, value, period)*/ 00060 /** 00061 * @ingroup timer 00062 * @brief Set an alarm to execute a callback function when expired. 00063 * @param *d Pointer to a CAN object data structure 00064 * @param id The alarm Id 00065 * @param callback A callback function 00066 * @param value Call the callback function at current time + value 00067 * @param period Call periodically the callback function 00068 * @return handle The timer handle 00069 */ 00070 TIMER_HANDLE SetAlarm(CO_Data* d, UNS32 id, TimerCallback_t callback, TIMEVAL value, TIMEVAL period); 00071 00072 /** 00073 * @ingroup timer 00074 * @brief Delete an alarm before expiring. 00075 * @param handle A timer handle 00076 * @return The timer handle 00077 */ 00078 TIMER_HANDLE DelAlarm(TIMER_HANDLE handle); 00079 00080 void TimeDispatch(void); 00081 00082 /** 00083 * @ingroup timer 00084 * @brief Set a timerfor a given time. 00085 * @param value The time value. 00086 */ 00087 void setTimer(TIMEVAL value); 00088 00089 /** 00090 * @ingroup timer 00091 * @brief Get the time elapsed since latest timer occurence. 00092 * @return time elapsed since latest timer occurence 00093 */ 00094 TIMEVAL getElapsedTime(void); 00095 00096 #ifdef __cplusplus 00097 } 00098 #endif 00099 00100 #endif /* #define __timer_h__ */
Generated on Tue Jul 12 2022 17:11:51 by
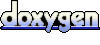