
Test program for capacitive sensing using the Freescale MPR121 on the Sparkfun SEN-10250 BoB
Fork of MPR121_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "MPR121.h" 00003 00004 Serial pc(USBTX, USBRX); 00005 DigitalOut myled(LED1); 00006 00007 #if defined TARGET_LPC1768 || TARGET_LPC11U24 00008 I2C i2c(p28, p27); 00009 InterruptIn irq(p26); 00010 MPR121 touch_pad(i2c, irq, MPR121::ADDR_VSS); 00011 00012 #elif defined TARGET_KL25Z 00013 I2C i2c(PTC9, PTC8); 00014 InterruptIn irq(PTA5); 00015 MPR121 touch_pad(i2c, irq, MPR121::ADDR_VSS); 00016 00017 #else 00018 #error TARGET NOT TESTED 00019 #endif 00020 00021 int main() 00022 { 00023 touch_pad.init(); 00024 touch_pad.enable(); 00025 touch_pad.registerDump(pc); 00026 00027 while(1) 00028 { 00029 if(touch_pad.isPressed()) 00030 { 00031 uint16_t button_val = touch_pad.buttonPressed(); 00032 printf("button = 0x%04x\n", button_val); 00033 myled = (button_val>0) ? 1 : 0; 00034 } 00035 } 00036 } 00037
Generated on Tue Jul 12 2022 21:37:17 by
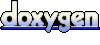