My attempt to made a more useful lib. You can get the accelerator and magnetometer.
Fork of LSM303DLH by
LSM303DLH.h
00001 #include "mbed.h" 00002 #include "vector.h" 00003 00004 #include "LSM303DLH_RegisterDef.h" 00005 00006 #ifndef M_PI 00007 #define M_PI 3.14159265358979323846 00008 #endif 00009 00010 /** Tilt-compensated compass interface Library for the STMicro LSM303DLH 3-axis magnetometer, 3-axis acceleromter 00011 * 00012 * This file is subject to the terms and conditions of the GNU Lesser 00013 * General Public License v2.1. See the file LICENSE in the top level 00014 * directory for more details. 00015 * 00016 * 00017 * 00018 * Base on the @see doccumentation https://cdn-shop.adafruit.com/datasheets/LSM303DLHC.PDF 00019 * @code 00020 * #include "mbed.h" 00021 * #include "LSM303DLH.h" 00022 * 00023 * Serial debug(USBTX,USBRX); 00024 * LSM303DLH compass(p28, p27); 00025 * 00026 * int main() { 00027 * float hdg; 00028 * debug.format(8,Serial::None,1); 00029 * debug.baud(115200); 00030 * debug.printf("LSM303DLH Test\x0d\x0a"); 00031 * compass.setOffset(29.50, -0.50, 4.00); // example calibration 00032 * compass.setScale(1.00, 1.03, 1.21); // example calibration 00033 * while(1) { 00034 * hdg = compass.heading(); 00035 * debug.printf("Heading: %.2f\n", hdg); 00036 * wait(0.1); 00037 * } 00038 * } 00039 * @endcode 00040 * 00041 * @author Salco <JeSuisSalco@gmail.com> 00042 */ 00043 00044 /** enable for MSB @ lower address */ 00045 //#define LSM303_LITLE_ENDIAN 00046 00047 class LSM303DLH { 00048 public: 00049 /** Create a new interface for an LSM303DLH 00050 * 00051 * @param sda is the pin for the I2C SDA line 00052 * @param scl is the pin for the I2C SCL line 00053 */ 00054 LSM303DLH(PinName sda, PinName scl); 00055 /** Create a new interface for an LSM303DLH 00056 * 00057 * @param ptrI2C is a pointer from existing I2C 00058 */ 00059 LSM303DLH(I2C* ptrI2C); 00060 /** Destructor of the class 00061 */ 00062 ~LSM303DLH(); 00063 /** sets the x, y, and z offset corrections for hard iron calibration 00064 * 00065 * Calibration details here: 00066 * http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/ 00067 * 00068 * If you gather raw magnetometer data and find, for example, x is offset 00069 * by hard iron by -20 then pass +20 to this member function to correct 00070 * for hard iron. 00071 * 00072 * @param x is the offset correction for the x axis 00073 * @param y is the offset correction for the y axis 00074 * @param z is the offset correction for the z axis 00075 */ 00076 void setOffset(float x, float y, float z); 00077 00078 /** sets the scale factor for the x, y, and z axes 00079 * 00080 * Calibratio details here: 00081 * http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/ 00082 * 00083 * Sensitivity of the three axes is never perfectly identical and this 00084 * function can help to correct differences in sensitivity. You're 00085 * supplying a multipler such that x, y and z will be normalized to the 00086 * same max/min values 00087 */ 00088 void setScale(float x, float y, float z); 00089 00090 /** read the raw accelerometer and compass values 00091 * 00092 * @param a is the accelerometer 3d vector, written by the function 00093 * @param m is the magnetometer 3d vector, written by the function 00094 */ 00095 bool read(vector &a, vector &m); 00096 /** read the raw accelerometer values 00097 * 00098 * @param a is the accelerometer 3d vector, written by the function 00099 */ 00100 bool read_acc_raw(vector *a); 00101 /** read the raw compass values 00102 * 00103 * @param m is the magnetometer 3d vector, written by the function 00104 */ 00105 bool read_mag_raw(vector *m); 00106 00107 /** returns the magnetic heading with respect to the y axis 00108 * 00109 */ 00110 float heading(void); 00111 00112 /** returns the heading with respect to the specified vector 00113 * 00114 */ 00115 float heading(vector from); 00116 00117 /** sets the I2C bus frequency 00118 * 00119 * @param frequency is the I2C bus/clock frequency, either standard (100000) or fast (400000) 00120 */ 00121 void frequency(int hz); 00122 00123 private: 00124 enum DEV_ADDRS { 00125 /* --- Mag --- */ 00126 addr_mag = 0x3c, 00127 /* --- Acc --- */ 00128 addr_acc = 0x32,//0x30; 00129 }; 00130 00131 enum REG_ADDRS { 00132 /* --- Mag --- */ 00133 CRA_REG_M = 0x00, 00134 CRB_REG_M = 0x01, 00135 MR_REG_M = 0x02, 00136 OUT_X_M = 0x03, 00137 OUT_Y_M = 0x05, 00138 OUT_Z_M = 0x07, 00139 SR_REG_M = 0x09, 00140 /* --- Acc --- */ 00141 CTRL_REG1_A = 0x20, 00142 CTRL_REG4_A = 0x23, 00143 STATUS_REG_A= 0x27, 00144 OUT_X_A = 0x28, 00145 OUT_Y_A = 0x2A, 00146 OUT_Z_A = 0x2C, 00147 }; 00148 00149 I2C* m_ptr_I2C;//_compass; 00150 float _offset_x; 00151 float _offset_y; 00152 float _offset_z; 00153 float _scale_x; 00154 float _scale_y; 00155 float _scale_z; 00156 long _filt_ax; 00157 long _filt_ay; 00158 long _filt_az; 00159 int8_t m_FS; 00160 int8_t m_GN; 00161 bool m_have_createdI2C; 00162 void init(void) ; 00163 00164 bool write_reg(int addr_i2c,int addr_reg, uint8_t v); 00165 bool write_reg(int addr_i2c,int addr_reg, char v); 00166 00167 bool read_reg(int addr_i2c,int addr_reg, uint8_t *v); 00168 bool read_reg(int addr_i2c,int addr_reg, char *v); 00169 00170 bool read_reg_short(int addr_i2c,int addr_reg, short *v); 00171 bool read_reg_short(DEV_ADDRS addr_i2c,REG_ADDRS addr_reg, OUT_XYZ_t *dataRead); 00172 00173 int8_t get_FullScall_selection(void); 00174 float get_acc_value_in_g(OUT_XYZ_t* dataOut); 00175 };
Generated on Fri Jul 15 2022 14:50:37 by
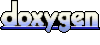