
integrating vr 1.0
Dependencies: SDFileSystem mbed-rtos mbed-src mbed
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "pin_config.h" 00004 #include "SDFileSystem.h" 00005 #include "string.h" 00006 00007 const int addr = 0x20; //slave address 00008 I2C master (PIN32,PIN31); //configure pins p27,p28 as I2C master 00009 //I2C master (PIN32,PIN31); 00010 Serial pc (USBTX,USBRX); 00011 DigitalOut interrupt(PIN4); 00012 InterruptIn data_ready(PIN39); 00013 //DigitalOut interrupt(D9); 00014 //InterruptIn data_ready(D10); 00015 int reset; 00016 Timer t; 00017 Timer t1; 00018 Timer t2; 00019 Timer t3; 00020 00021 void store_data(); 00022 00023 typedef struct 00024 { 00025 char data; // To avoid dynamic memory allocation 00026 int length; 00027 }i2c_data; 00028 00029 00030 00031 //Mail<i2c_data,16> i2c_data_receive; 00032 Mail<i2c_data,16> i2c_data_send; 00033 00034 Thread * ptr_t_i2c; 00035 void FUNC_I2C_MASTER_FSLAVE(char * data,int length) 00036 { 00037 00038 00039 bool ack0 =true; 00040 00041 interrupt = 1; 00042 t1.start(); 00043 //wait_ms(20); 00044 ack0 = master.read(addr|1,data,length); 00045 t1.stop(); 00046 00047 00048 if(!ack0) 00049 { 00050 printf("\n master has read %s from slave\n\r",data); 00051 00052 } 00053 //master.stop(); 00054 00055 store_data(); 00056 printf("\n%d\n\r",t1.read_us()); 00057 t1.reset(); 00058 00059 interrupt=0; 00060 00061 } 00062 00063 typedef struct { 00064 char Voltage[9]; 00065 char Current[5]; 00066 char Temperature[2]; 00067 char PanelTemperature[3];//read by the 4 thermistors on solar panels 00068 char BatteryTemperature; //to be populated 00069 char faultpoll; //polled faults 00070 char faultir; //interrupted faults 00071 char power_mode; //power modes 00072 char AngularSpeed[3]; 00073 char Bnewvalue[3]; 00074 00075 //float magnetometer,gyro=>to be addes 00076 } hk_data; 00077 hk_data decode_data; 00078 00079 /*void TC_DECODE(char *data_hk) //getting the structure back from hk data sent by bae 00080 { 00081 for(int i=0;i<=7;i++) 00082 { 00083 decode_data.Voltage[i] = data_hk[i]; 00084 decode_data.Voltage[8] = '\0'; 00085 } 00086 for(int i=0;i<=3;i++) 00087 { 00088 decode_data.Current[i] = data_hk[8+i]; 00089 decode_data.Current[4] = '\0'; 00090 } 00091 decode_data.Temperature[0] = data_hk[12]; 00092 decode_data.Temperature[1] = '\0'; 00093 for(int i=0;i<=1;i++) 00094 { 00095 decode_data.PanelTemperature[i] = data_hk[13+i]; 00096 decode_data.PanelTemperature[2] = '\0'; 00097 } 00098 decode_data.BatteryTemperature = data_hk[15]; 00099 decode_data.faultpoll = data_hk[16]; 00100 decode_data.faultir = data_hk[17]; 00101 decode_data.power_mode = data_hk[18]; 00102 for(int i=0;i<=1;i++) 00103 { 00104 decode_data.AngularSpeed[i] = data_hk[19+i]; 00105 decode_data.AngularSpeed[2] = '\0'; 00106 } 00107 for(int i=0;i<=1;i++) 00108 { 00109 decode_data.Bnewvalue[i] = data_hk[21+i]; 00110 decode_data.Bnewvalue[2] = '\0'; 00111 } 00112 printf("\n voltage %s\n\r",decode_data.Voltage); 00113 printf("\n current %s\n\r",decode_data.Current); 00114 printf("\n faultpoll %c\n\r",decode_data.faultpoll); 00115 }*/ 00116 00117 00118 void T_I2C_MASTER_FSLAVE(void const *args) 00119 { 00120 char data_receive[25]; 00121 //char data_receive; 00122 while(1) 00123 { 00124 Thread::signal_wait(0x1); 00125 00126 00127 FUNC_I2C_MASTER_FSLAVE(data_receive,25); 00128 /*i2c_data * i2c_data_r = i2c_data_receive.alloc(); 00129 strcpy(i2c_data_r->data , data_receive); 00130 i2c_data_r->length = 25; 00131 i2c_data_receive.put(i2c_data_r);*/ 00132 printf("\n Data received from slave is %s\n\r",data_receive); 00133 //TC_DECODE(data_receive); 00134 } 00135 } 00136 00137 void FUNC_INT() 00138 { 00139 00140 ptr_t_i2c->signal_set(0x1); 00141 00142 } 00143 00144 char writedata; 00145 bool write2slave; 00146 bool master_status_write; 00147 void FUNC_MASTER_WRITE() 00148 { //wait(1); 00149 write2slave=true; 00150 00151 00152 00153 char data = pc.getc(); 00154 interrupt = 1; 00155 t.start(); 00156 t3.start(); 00157 // wait_ms(20); 00158 i2c_data * i2c_data_s = i2c_data_send.alloc(); 00159 i2c_data_s->data = data; 00160 i2c_data_s->length = 1; 00161 i2c_data_send.put(i2c_data_s); 00162 master_status_write = true; 00163 00164 // interrupt = 1; 00165 00166 osEvent evt = i2c_data_send.get(); 00167 if (evt.status == osEventMail) 00168 { 00169 i2c_data *i2c_data_s = (i2c_data*)evt.value.p; 00170 writedata = i2c_data_s -> data; 00171 t.stop(); 00172 //t3.start(); 00173 master_status_write = (bool) master.write(addr|0x00,&writedata,1); 00174 t3.stop(); 00175 if(master_status_write==0) 00176 { 00177 printf("\n\r CDMS has written %c to PAYLOAD\n\r",writedata); 00178 write2slave=false; 00179 } 00180 i2c_data_send.free(i2c_data_s); 00181 printf("\n%d",t.read_us()); 00182 t.reset(); 00183 printf("\n%d\n",t3.read_us()); 00184 t3.reset(); 00185 } 00186 interrupt = 0; 00187 } 00188 00189 /********************************** Function Prototypes declaration ***********************************/ 00190 int FUNC_CDMS_hex2int(int); // Need to convert the RTC time values to integers 00191 void FUNC_CDMS_Gettime(void); // Function to get the time values from RTC registers 00192 void FUNC_CDMS_init_values(void); // Function to initialize the registers in the RTC 00193 00194 00195 /***************** Configure the SPI1 of the CDMS uc as the data bus for the RTC***********************/ 00196 SPI spi(PTE1,PTE3, PTE2); // mosi, miso, sclk 00197 DigitalOut cs(PTE29); // PTE29 is used for chip select 00198 00199 char ch; 00200 SDFileSystem sd(PTE1, PTE3, PTE2, PTE22, "sd"); // the pinout on the mbed Cool Components workshop board 00201 //Serial pc(USBTX, USBRX); // tx, rx 00202 char time_stamp[15]; 00203 00204 /***********************Initialization function of the RTC********************************************/ 00205 void FUNC_CDMS_init_values(void) 00206 { 00207 cs=0; 00208 spi.format(8,3); // Set the data bit with to be of 8 bits, 00209 // data tx mode is 3 00210 spi.frequency(1000000); // Set Data rate to be 1 MHz 00211 00212 cs = 0; // Set chip select pin to be 0: Activate RTC chip 00213 spi.write(0x80); // Send the address of the Seconds register 0x80 00214 spi.write(0x00); // Set seconds value to 0 00215 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00216 00217 cs=0; // Set chip select pin to be 0: Activate RTC chip 00218 spi.write(0x81); // Send the address of the Minutes register 0x81 00219 spi.write(0x00); // Set minutes value to 0 00220 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00221 00222 cs=0; // Set chip select pin to be 0: Activate RTC chip 00223 spi.write(0x82); // Send the address of the Hours register 0x82 00224 spi.write(0x00); // Set hours value to 0 00225 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00226 00227 cs=0; // Set chip select pin to be 0: Activate RTC chip 00228 spi.write(0x83); // Send the address of the Day register 0x83 00229 spi.write(0x01); // Set the day to 01 00230 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00231 00232 cs=0; // Set chip select pin to be 0: Activate RTC chip 00233 spi.write(0x84); // Send the address of the date register 0x84 00234 spi.write(0x01); // Set date of the month to 01 00235 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00236 00237 cs=0; // Set chip select pin to be 0: Activate RTC chip 00238 spi.write(0x85); // Send the address of the Month register 0x80 00239 spi.write(0x01); // Set month to 01 00240 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00241 00242 cs=0; // Set chip select pin to be 0: Activate RTC chip 00243 spi.write(0x86); // Send the address of the year register 0x80 00244 spi.write(0x00); // Set year to 00(2000) 00245 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00246 }// End of INIT Function 00247 00248 00249 /*********************************Function to read the RTC registers*********************************/ 00250 void FUNC_CDMS_Gettime() 00251 { 00252 00253 spi.format(8,3); // Set the data bit with to be of 8 bits, 00254 // data tx mode is 3 00255 spi.frequency(1000000); // Set Data rate to be 1 MHz 00256 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00257 00258 cs=0; // Set chip select pin to be 0: Activate RTC chip 00259 spi.write(0x00); // Sending address of seconds register 00260 int seconds = spi.write(0x00); // Read the value by sending dummy byte 00261 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00262 00263 cs=0; // Set chip select pin to be 0: Activate RTC chip 00264 spi.write(0x01); // Sending address of Minutes register 00265 int minutes =spi.write(0x01); // Read the value by sending dummy byte 00266 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00267 00268 cs=0; // Set chip select pin to be 0: Activate RTC chip 00269 spi.write(0x02); // Sending address of hours register 00270 int hours =spi.write(0x01); // Read the value by sending dummy byte 00271 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00272 00273 cs=0; // Set chip select pin to be 0: Activate RTC chip 00274 spi.write(0x03); // Sending address of day register 00275 int day =spi.write(0x01); // Read the value by sending dummy byte 00276 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00277 00278 cs=0; // Set chip select pin to be 0: Activate RTC chip 00279 spi.write(0x04); // Sending address of date register 00280 int date =spi.write(0x01); // Read the value by sending dummy byte 00281 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00282 00283 cs=0; // Set chip select pin to be 0: Activate RTC chip 00284 spi.write(0x05); // Sending address of month register 00285 int month =spi.write(0x01); // Read the value by sending dummy byte 00286 cs=1; // Set chip select pin to be 1: DeActivate RTC chip 00287 00288 cs=0; // Set chip select pin to be 0: Activate RTC chip 00289 spi.write(0x06); // Sending address of year register 00290 int year =spi.write(0x01); // Read the value by sending dummy byte 00291 cs = 1; // Set chip select pin to be 1: DeActivate RTC chip 00292 00293 // RTC sends in BCD format.. SO we convert the values generated by RTC to integers 00294 year = FUNC_CDMS_hex2int(year); 00295 month = FUNC_CDMS_hex2int(month); 00296 date = FUNC_CDMS_hex2int(date); 00297 day = FUNC_CDMS_hex2int(day); 00298 hours = FUNC_CDMS_hex2int(hours); 00299 minutes = FUNC_CDMS_hex2int(minutes); 00300 seconds = FUNC_CDMS_hex2int(seconds); 00301 00302 // Print the obtained Time stamp 00303 //printf("The time is :%d %d %d %d %d %d %d \n\r",seconds,minutes,hours,day,date,month,year); 00304 sprintf(time_stamp,"%02d%02d%02d%02d%02d%02d",year,month,date,hours,minutes,seconds); 00305 printf(" \n\r Received HK data from BAE"); 00306 printf("\n HK_data stored in %s.txt",time_stamp); 00307 //puts(time_stamp); 00308 //printf(".txt"); 00309 }//End of Read Function 00310 00311 /**************************Function to convert Hex values to Int values*****************************/ 00312 int FUNC_CDMS_hex2int(int a) 00313 { 00314 a=(a/16)*10+(a%16); //function to convert hex type to int type 00315 return a; 00316 }// End of convert function 00317 00318 00319 /******************************************END OF RTC CODE ****************************************/ 00320 00321 char count = 10; 00322 void store_data() 00323 { 00324 FUNC_CDMS_Gettime(); 00325 00326 char hk_data[25]; 00327 count++; 00328 strcpy(hk_data,"hk_Data "); 00329 strcat(hk_data,"!@@#"); 00330 hk_data[10] = count; 00331 //storedata(hk_data); 00332 mkdir("/sd/hk", 0777); 00333 char add[20]; 00334 strcpy(add,"/sd/hk/"); 00335 strcat(add,time_stamp); 00336 strcat(add,".txt"); 00337 00338 /*FILE *fp = fopen(add, "w"); 00339 if(fp == NULL) { 00340 error("Could not open file for write\n"); 00341 } 00342 else 00343 { 00344 00345 fprintf(fp, "%s ",hk_data); 00346 fclose(fp); 00347 printf("\n File stored in SD card\n");*/ 00348 //printf("\n Reading from the file .... \n Data is %s\n",hk_data); 00349 00350 00351 } 00352 00353 00354 00355 //void create_file() 00356 //{ 00357 00358 /* 00359 fprintf(fp, "Hello fun SD Card World!\n"); 00360 fclose(fp); 00361 00362 fp = fopen("/sd/mydir/sdtest.txt", "r"); 00363 if(fp == NULL) { 00364 error("Could not open file for write\n"); 00365 } 00366 //fprintf(fp, "Hello fun SD Card World!"); 00367 // fprintf("The contents of %s file are :\n", "/sd/mydir/sdtest.txt"); 00368 while( ( ch = fgetc(fp) ) != '\n' ) 00369 { 00370 pc.printf("%c",ch); 00371 } 00372 //fprintf(fp); 00373 fclose(fp); 00374 00375 printf("Jai Mata Di..Goodbye World!\n");*/ 00376 //} 00377 00378 //Ticker t; 00379 00380 00381 int main() 00382 { 00383 interrupt=0; 00384 ptr_t_i2c = new Thread(T_I2C_MASTER_FSLAVE); 00385 data_ready.rise(&FUNC_INT); 00386 printf("\n\rIITMSAT CDMS EXECUTED\n"); 00387 master.frequency(100000); 00388 00389 FUNC_CDMS_init_values(); 00390 // t.attach(&store_data, 20.0); 00391 while(1) 00392 { //Thread::wait(9000); 00393 //interrupt = 1; 00394 00395 FUNC_MASTER_WRITE(); 00396 //interrupt = 0; 00397 ; 00398 } 00399 }
Generated on Sat Jul 16 2022 09:05:24 by
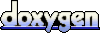