wolfSSL SSL/TLS library, support up to TLS1.3
Embed:
(wiki syntax)
Show/hide line numbers
evp.h
Go to the documentation of this file.
00001 /* evp.h 00002 * 00003 * Copyright (C) 2006-2017 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 00023 00024 /*! 00025 \file wolfssl/openssl/evp.h 00026 \brief evp.h defines mini evp openssl compatibility layer 00027 */ 00028 00029 00030 #ifndef WOLFSSL_EVP_H_ 00031 #define WOLFSSL_EVP_H_ 00032 00033 #include <wolfssl/wolfcrypt/settings.h> 00034 00035 #ifdef WOLFSSL_PREFIX 00036 #include "prefix_evp.h" 00037 #endif 00038 00039 #ifndef NO_MD4 00040 #include <wolfssl/openssl/md4.h> 00041 #endif 00042 #ifndef NO_MD5 00043 #include <wolfssl/openssl/md5.h> 00044 #endif 00045 #include <wolfssl/openssl/sha.h> 00046 #include <wolfssl/openssl/ripemd.h> 00047 #include <wolfssl/openssl/rsa.h> 00048 #include <wolfssl/openssl/dsa.h> 00049 #include <wolfssl/openssl/ec.h> 00050 00051 #include <wolfssl/wolfcrypt/aes.h> 00052 #include <wolfssl/wolfcrypt/des3.h> 00053 #include <wolfssl/wolfcrypt/arc4.h> 00054 #include <wolfssl/wolfcrypt/hmac.h> 00055 #ifdef HAVE_IDEA 00056 #include <wolfssl/wolfcrypt/idea.h> 00057 #endif 00058 #include <wolfssl/wolfcrypt/pwdbased.h> 00059 00060 #ifdef __cplusplus 00061 extern "C" { 00062 #endif 00063 00064 00065 typedef char WOLFSSL_EVP_CIPHER; 00066 #ifndef WOLFSSL_EVP_TYPE_DEFINED /* guard on redeclaration */ 00067 typedef char WOLFSSL_EVP_MD; 00068 typedef struct WOLFSSL_EVP_PKEY WOLFSSL_EVP_PKEY; 00069 #define WOLFSSL_EVP_TYPE_DEFINED 00070 #endif 00071 00072 typedef WOLFSSL_EVP_PKEY EVP_PKEY; 00073 typedef WOLFSSL_EVP_PKEY PKCS8_PRIV_KEY_INFO; 00074 00075 #ifndef NO_MD4 00076 WOLFSSL_API const WOLFSSL_EVP_MD* wolfSSL_EVP_md4(void); 00077 #endif 00078 #ifndef NO_MD5 00079 WOLFSSL_API const WOLFSSL_EVP_MD* wolfSSL_EVP_md5(void); 00080 #endif 00081 WOLFSSL_API const WOLFSSL_EVP_MD* wolfSSL_EVP_sha1(void); 00082 WOLFSSL_API const WOLFSSL_EVP_MD* wolfSSL_EVP_sha224(void); 00083 WOLFSSL_API const WOLFSSL_EVP_MD* wolfSSL_EVP_sha256(void); 00084 WOLFSSL_API const WOLFSSL_EVP_MD* wolfSSL_EVP_sha384(void); 00085 WOLFSSL_API const WOLFSSL_EVP_MD* wolfSSL_EVP_sha512(void); 00086 WOLFSSL_API const WOLFSSL_EVP_MD* wolfSSL_EVP_ripemd160(void); 00087 00088 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_aes_128_ecb(void); 00089 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_aes_192_ecb(void); 00090 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_aes_256_ecb(void); 00091 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_aes_128_cbc(void); 00092 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_aes_192_cbc(void); 00093 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_aes_256_cbc(void); 00094 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_aes_128_ctr(void); 00095 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_aes_192_ctr(void); 00096 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_aes_256_ctr(void); 00097 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_des_ecb(void); 00098 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_des_ede3_ecb(void); 00099 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_des_cbc(void); 00100 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_des_ede3_cbc(void); 00101 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_rc4(void); 00102 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_idea_cbc(void); 00103 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_enc_null(void); 00104 00105 00106 typedef union { 00107 #ifndef NO_MD4 00108 WOLFSSL_MD4_CTX md4; 00109 #endif 00110 #ifndef NO_MD5 00111 WOLFSSL_MD5_CTX md5; 00112 #endif 00113 WOLFSSL_SHA_CTX sha; 00114 #ifdef WOLFSSL_SHA224 00115 WOLFSSL_SHA224_CTX sha224; 00116 #endif 00117 WOLFSSL_SHA256_CTX sha256; 00118 #ifdef WOLFSSL_SHA384 00119 WOLFSSL_SHA384_CTX sha384; 00120 #endif 00121 #ifdef WOLFSSL_SHA512 00122 WOLFSSL_SHA512_CTX sha512; 00123 #endif 00124 #ifdef WOLFSSL_RIPEMD 00125 WOLFSSL_RIPEMD_CTX ripemd; 00126 #endif 00127 } WOLFSSL_Hasher; 00128 00129 00130 typedef struct WOLFSSL_EVP_MD_CTX { 00131 union { 00132 WOLFSSL_Hasher digest; 00133 Hmac hmac; 00134 } hash; 00135 unsigned char macType; 00136 } WOLFSSL_EVP_MD_CTX; 00137 00138 00139 typedef union { 00140 #ifndef NO_AES 00141 Aes aes; 00142 #endif 00143 #ifndef NO_DES3 00144 Des des; 00145 Des3 des3; 00146 #endif 00147 Arc4 arc4; 00148 #ifdef HAVE_IDEA 00149 Idea idea; 00150 #endif 00151 } WOLFSSL_Cipher; 00152 00153 00154 enum { 00155 AES_128_CBC_TYPE = 1, 00156 AES_192_CBC_TYPE = 2, 00157 AES_256_CBC_TYPE = 3, 00158 AES_128_CTR_TYPE = 4, 00159 AES_192_CTR_TYPE = 5, 00160 AES_256_CTR_TYPE = 6, 00161 AES_128_ECB_TYPE = 7, 00162 AES_192_ECB_TYPE = 8, 00163 AES_256_ECB_TYPE = 9, 00164 DES_CBC_TYPE = 10, 00165 DES_ECB_TYPE = 11, 00166 DES_EDE3_CBC_TYPE = 12, 00167 DES_EDE3_ECB_TYPE = 13, 00168 ARC4_TYPE = 14, 00169 NULL_CIPHER_TYPE = 15, 00170 EVP_PKEY_RSA = 16, 00171 EVP_PKEY_DSA = 17, 00172 EVP_PKEY_EC = 18, 00173 #ifdef HAVE_IDEA 00174 IDEA_CBC_TYPE = 19, 00175 #endif 00176 NID_sha1 = 64, 00177 NID_sha224 = 65, 00178 NID_md2 = 77, 00179 NID_md5 = 4, 00180 NID_hmac = 855, 00181 EVP_PKEY_HMAC = NID_hmac 00182 }; 00183 00184 enum { 00185 NID_aes_128_cbc = 419, 00186 NID_aes_192_cbc = 423, 00187 NID_aes_256_cbc = 427, 00188 NID_aes_128_ctr = 904, 00189 NID_aes_192_ctr = 905, 00190 NID_aes_256_ctr = 906, 00191 NID_aes_128_ecb = 418, 00192 NID_aes_192_ecb = 422, 00193 NID_aes_256_ecb = 426, 00194 NID_des_cbc = 31, 00195 NID_des_ecb = 29, 00196 NID_des_ede3_cbc= 44, 00197 NID_des_ede3_ecb= 33, 00198 NID_idea_cbc = 34, 00199 }; 00200 00201 #define WOLFSSL_EVP_BUF_SIZE 16 00202 typedef struct WOLFSSL_EVP_CIPHER_CTX { 00203 int keyLen; /* user may set for variable */ 00204 int block_size; 00205 unsigned long flags; 00206 unsigned char enc; /* if encrypt side, then true */ 00207 unsigned char cipherType; 00208 #ifndef NO_AES 00209 /* working iv pointer into cipher */ 00210 ALIGN16 unsigned char iv[AES_BLOCK_SIZE]; 00211 #elif !defined(NO_DES3) 00212 /* working iv pointer into cipher */ 00213 ALIGN16 unsigned char iv[DES_BLOCK_SIZE]; 00214 #endif 00215 WOLFSSL_Cipher cipher; 00216 ALIGN16 byte buf[WOLFSSL_EVP_BUF_SIZE]; 00217 int bufUsed; 00218 ALIGN16 byte lastBlock[WOLFSSL_EVP_BUF_SIZE]; 00219 int lastUsed; 00220 } WOLFSSL_EVP_CIPHER_CTX; 00221 00222 typedef struct WOLFSSL_EVP_PKEY_CTX { 00223 WOLFSSL_EVP_PKEY *pkey; 00224 int op; /* operation */ 00225 int padding; 00226 } WOLFSSL_EVP_PKEY_CTX; 00227 00228 typedef int WOLFSSL_ENGINE ; 00229 typedef WOLFSSL_ENGINE ENGINE; 00230 typedef WOLFSSL_EVP_PKEY_CTX EVP_PKEY_CTX; 00231 00232 #define EVP_PKEY_OP_ENCRYPT (1 << 6) 00233 #define EVP_PKEY_OP_DECRYPT (1 << 7) 00234 00235 WOLFSSL_API void wolfSSL_EVP_init(void); 00236 WOLFSSL_API int wolfSSL_EVP_MD_size(const WOLFSSL_EVP_MD* md); 00237 WOLFSSL_API int wolfSSL_EVP_MD_type(const WOLFSSL_EVP_MD *md); 00238 00239 WOLFSSL_API WOLFSSL_EVP_MD_CTX *wolfSSL_EVP_MD_CTX_new (void); 00240 WOLFSSL_API void wolfSSL_EVP_MD_CTX_free(WOLFSSL_EVP_MD_CTX* ctx); 00241 WOLFSSL_API void wolfSSL_EVP_MD_CTX_init(WOLFSSL_EVP_MD_CTX* ctx); 00242 WOLFSSL_API int wolfSSL_EVP_MD_CTX_cleanup(WOLFSSL_EVP_MD_CTX* ctx); 00243 WOLFSSL_API int wolfSSL_EVP_MD_CTX_copy(WOLFSSL_EVP_MD_CTX *out, const WOLFSSL_EVP_MD_CTX *in); 00244 WOLFSSL_API int wolfSSL_EVP_MD_CTX_copy_ex(WOLFSSL_EVP_MD_CTX *out, const WOLFSSL_EVP_MD_CTX *in); 00245 WOLFSSL_API int wolfSSL_EVP_MD_CTX_type(const WOLFSSL_EVP_MD_CTX *ctx); 00246 WOLFSSL_API const WOLFSSL_EVP_MD *wolfSSL_EVP_MD_CTX_md(const WOLFSSL_EVP_MD_CTX *ctx); 00247 WOLFSSL_API const WOLFSSL_EVP_CIPHER *wolfSSL_EVP_get_cipherbyname(const char *name); 00248 WOLFSSL_API const WOLFSSL_EVP_MD *wolfSSL_EVP_get_digestbyname(const char *name); 00249 00250 WOLFSSL_API int wolfSSL_EVP_DigestInit(WOLFSSL_EVP_MD_CTX* ctx, 00251 const WOLFSSL_EVP_MD* type); 00252 WOLFSSL_API int wolfSSL_EVP_DigestInit_ex(WOLFSSL_EVP_MD_CTX* ctx, 00253 const WOLFSSL_EVP_MD* type, 00254 WOLFSSL_ENGINE *impl); 00255 WOLFSSL_API int wolfSSL_EVP_DigestUpdate(WOLFSSL_EVP_MD_CTX* ctx, const void* data, 00256 size_t sz); 00257 WOLFSSL_API int wolfSSL_EVP_DigestFinal(WOLFSSL_EVP_MD_CTX* ctx, unsigned char* md, 00258 unsigned int* s); 00259 WOLFSSL_API int wolfSSL_EVP_DigestFinal_ex(WOLFSSL_EVP_MD_CTX* ctx, 00260 unsigned char* md, unsigned int* s); 00261 00262 WOLFSSL_API int wolfSSL_EVP_DigestSignInit(WOLFSSL_EVP_MD_CTX *ctx, 00263 WOLFSSL_EVP_PKEY_CTX **pctx, 00264 const WOLFSSL_EVP_MD *type, 00265 WOLFSSL_ENGINE *e, 00266 WOLFSSL_EVP_PKEY *pkey); 00267 WOLFSSL_API int wolfSSL_EVP_DigestSignUpdate(WOLFSSL_EVP_MD_CTX *ctx, 00268 const void *d, unsigned int cnt); 00269 WOLFSSL_API int wolfSSL_EVP_DigestSignFinal(WOLFSSL_EVP_MD_CTX *ctx, 00270 unsigned char *sig, size_t *siglen); 00271 00272 WOLFSSL_API int wolfSSL_EVP_BytesToKey(const WOLFSSL_EVP_CIPHER*, 00273 const WOLFSSL_EVP_MD*, const unsigned char*, 00274 const unsigned char*, int, int, unsigned char*, 00275 unsigned char*); 00276 00277 WOLFSSL_API void wolfSSL_EVP_CIPHER_CTX_init(WOLFSSL_EVP_CIPHER_CTX* ctx); 00278 WOLFSSL_API int wolfSSL_EVP_CIPHER_CTX_cleanup(WOLFSSL_EVP_CIPHER_CTX* ctx); 00279 00280 WOLFSSL_API int wolfSSL_EVP_CIPHER_CTX_iv_length(const WOLFSSL_EVP_CIPHER_CTX*); 00281 WOLFSSL_API int wolfSSL_EVP_CIPHER_iv_length(const WOLFSSL_EVP_CIPHER*); 00282 WOLFSSL_API int wolfSSL_EVP_Cipher_key_length(const WOLFSSL_EVP_CIPHER* c); 00283 00284 00285 WOLFSSL_API int wolfSSL_EVP_CipherInit(WOLFSSL_EVP_CIPHER_CTX* ctx, 00286 const WOLFSSL_EVP_CIPHER* type, 00287 const unsigned char* key, 00288 const unsigned char* iv, 00289 int enc); 00290 WOLFSSL_API int wolfSSL_EVP_CipherInit_ex(WOLFSSL_EVP_CIPHER_CTX* ctx, 00291 const WOLFSSL_EVP_CIPHER* type, 00292 WOLFSSL_ENGINE *impl, 00293 const unsigned char* key, 00294 const unsigned char* iv, 00295 int enc); 00296 WOLFSSL_API int wolfSSL_EVP_EncryptInit(WOLFSSL_EVP_CIPHER_CTX* ctx, 00297 const WOLFSSL_EVP_CIPHER* type, 00298 const unsigned char* key, 00299 const unsigned char* iv); 00300 WOLFSSL_API int wolfSSL_EVP_EncryptInit_ex(WOLFSSL_EVP_CIPHER_CTX* ctx, 00301 const WOLFSSL_EVP_CIPHER* type, 00302 WOLFSSL_ENGINE *impl, 00303 const unsigned char* key, 00304 const unsigned char* iv); 00305 WOLFSSL_API int wolfSSL_EVP_DecryptInit(WOLFSSL_EVP_CIPHER_CTX* ctx, 00306 const WOLFSSL_EVP_CIPHER* type, 00307 const unsigned char* key, 00308 const unsigned char* iv); 00309 WOLFSSL_API int wolfSSL_EVP_DecryptInit_ex(WOLFSSL_EVP_CIPHER_CTX* ctx, 00310 const WOLFSSL_EVP_CIPHER* type, 00311 WOLFSSL_ENGINE *impl, 00312 const unsigned char* key, 00313 const unsigned char* iv); 00314 WOLFSSL_API int wolfSSL_EVP_CipherUpdate(WOLFSSL_EVP_CIPHER_CTX *ctx, 00315 unsigned char *out, int *outl, 00316 const unsigned char *in, int inl); 00317 WOLFSSL_API int wolfSSL_EVP_CipherFinal(WOLFSSL_EVP_CIPHER_CTX *ctx, 00318 unsigned char *out, int *outl); 00319 WOLFSSL_API int wolfSSL_EVP_CipherFinal_ex(WOLFSSL_EVP_CIPHER_CTX *ctx, 00320 unsigned char *out, int *outl, int enc); 00321 WOLFSSL_API int wolfSSL_EVP_EncryptFinal(WOLFSSL_EVP_CIPHER_CTX *ctx, 00322 unsigned char *out, int *outl); 00323 WOLFSSL_API int wolfSSL_EVP_EncryptFinal_ex(WOLFSSL_EVP_CIPHER_CTX *ctx, 00324 unsigned char *out, int *outl); 00325 WOLFSSL_API int wolfSSL_EVP_DecryptFinal(WOLFSSL_EVP_CIPHER_CTX *ctx, 00326 unsigned char *out, int *outl); 00327 WOLFSSL_API int wolfSSL_EVP_DecryptFinal_ex(WOLFSSL_EVP_CIPHER_CTX *ctx, 00328 unsigned char *out, int *outl); 00329 00330 WOLFSSL_API WOLFSSL_EVP_CIPHER_CTX *wolfSSL_EVP_CIPHER_CTX_new(void); 00331 WOLFSSL_API void wolfSSL_EVP_CIPHER_CTX_free(WOLFSSL_EVP_CIPHER_CTX *ctx); 00332 WOLFSSL_API int wolfSSL_EVP_CIPHER_CTX_key_length(WOLFSSL_EVP_CIPHER_CTX* ctx); 00333 WOLFSSL_API int wolfSSL_EVP_CIPHER_CTX_set_key_length(WOLFSSL_EVP_CIPHER_CTX* ctx, 00334 int keylen); 00335 WOLFSSL_API int wolfSSL_EVP_Cipher(WOLFSSL_EVP_CIPHER_CTX* ctx, 00336 unsigned char* dst, unsigned char* src, 00337 unsigned int len); 00338 00339 WOLFSSL_API const WOLFSSL_EVP_CIPHER* wolfSSL_EVP_get_cipherbynid(int); 00340 WOLFSSL_API const WOLFSSL_EVP_MD* wolfSSL_EVP_get_digestbynid(int); 00341 00342 WOLFSSL_API WOLFSSL_RSA* wolfSSL_EVP_PKEY_get1_RSA(WOLFSSL_EVP_PKEY*); 00343 WOLFSSL_API WOLFSSL_DSA* wolfSSL_EVP_PKEY_get1_DSA(WOLFSSL_EVP_PKEY*); 00344 WOLFSSL_API WOLFSSL_EC_KEY *wolfSSL_EVP_PKEY_get1_EC_KEY(WOLFSSL_EVP_PKEY *key); 00345 WOLFSSL_API int wolfSSL_EVP_PKEY_set1_RSA(WOLFSSL_EVP_PKEY *pkey, WOLFSSL_RSA *key); 00346 00347 WOLFSSL_API WOLFSSL_EVP_PKEY* wolfSSL_EVP_PKEY_new_mac_key(int type, ENGINE* e, 00348 const unsigned char* key, int keylen); 00349 WOLFSSL_API const unsigned char* wolfSSL_EVP_PKEY_get0_hmac(const WOLFSSL_EVP_PKEY* pkey, 00350 size_t* len); 00351 WOLFSSL_API int wolfSSL_EVP_PKEY_bits(const WOLFSSL_EVP_PKEY *pkey); 00352 WOLFSSL_API int wolfSSL_EVP_PKEY_CTX_free(WOLFSSL_EVP_PKEY_CTX *ctx); 00353 WOLFSSL_API WOLFSSL_EVP_PKEY_CTX *wolfSSL_EVP_PKEY_CTX_new(WOLFSSL_EVP_PKEY *pkey, WOLFSSL_ENGINE *e); 00354 WOLFSSL_API int wolfSSL_EVP_PKEY_CTX_set_rsa_padding(WOLFSSL_EVP_PKEY_CTX *ctx, int padding); 00355 WOLFSSL_API int wolfSSL_EVP_PKEY_decrypt(WOLFSSL_EVP_PKEY_CTX *ctx, 00356 unsigned char *out, size_t *outlen, 00357 const unsigned char *in, size_t inlen); 00358 WOLFSSL_API int wolfSSL_EVP_PKEY_decrypt_init(WOLFSSL_EVP_PKEY_CTX *ctx); 00359 WOLFSSL_API int wolfSSL_EVP_PKEY_encrypt(WOLFSSL_EVP_PKEY_CTX *ctx, 00360 unsigned char *out, size_t *outlen, 00361 const unsigned char *in, size_t inlen); 00362 WOLFSSL_API int wolfSSL_EVP_PKEY_encrypt_init(WOLFSSL_EVP_PKEY_CTX *ctx); 00363 WOLFSSL_API WOLFSSL_EVP_PKEY *wolfSSL_EVP_PKEY_new(void); 00364 WOLFSSL_API void wolfSSL_EVP_PKEY_free(WOLFSSL_EVP_PKEY*); 00365 WOLFSSL_API int wolfSSL_EVP_PKEY_size(WOLFSSL_EVP_PKEY *pkey); 00366 WOLFSSL_API int wolfSSL_EVP_PKEY_type(int type); 00367 WOLFSSL_API int wolfSSL_EVP_PKEY_base_id(const EVP_PKEY *pkey); 00368 WOLFSSL_API int wolfSSL_EVP_SignFinal(WOLFSSL_EVP_MD_CTX *ctx, unsigned char *sigret, 00369 unsigned int *siglen, WOLFSSL_EVP_PKEY *pkey); 00370 WOLFSSL_API int wolfSSL_EVP_SignInit(WOLFSSL_EVP_MD_CTX *ctx, const WOLFSSL_EVP_MD *type); 00371 WOLFSSL_API int wolfSSL_EVP_SignUpdate(WOLFSSL_EVP_MD_CTX *ctx, const void *data, size_t len); 00372 WOLFSSL_API int wolfSSL_EVP_VerifyFinal(WOLFSSL_EVP_MD_CTX *ctx, 00373 unsigned char* sig, unsigned int sig_len, WOLFSSL_EVP_PKEY *pkey); 00374 WOLFSSL_API int wolfSSL_EVP_VerifyInit(WOLFSSL_EVP_MD_CTX *ctx, const WOLFSSL_EVP_MD *type); 00375 WOLFSSL_API int wolfSSL_EVP_VerifyUpdate(WOLFSSL_EVP_MD_CTX *ctx, const void *data, size_t len); 00376 00377 00378 /* these next ones don't need real OpenSSL type, for OpenSSH compat only */ 00379 WOLFSSL_API void* wolfSSL_EVP_X_STATE(const WOLFSSL_EVP_CIPHER_CTX* ctx); 00380 WOLFSSL_API int wolfSSL_EVP_X_STATE_LEN(const WOLFSSL_EVP_CIPHER_CTX* ctx); 00381 00382 WOLFSSL_API void wolfSSL_3des_iv(WOLFSSL_EVP_CIPHER_CTX* ctx, int doset, 00383 unsigned char* iv, int len); 00384 WOLFSSL_API void wolfSSL_aes_ctr_iv(WOLFSSL_EVP_CIPHER_CTX* ctx, int doset, 00385 unsigned char* iv, int len); 00386 00387 WOLFSSL_API int wolfSSL_StoreExternalIV(WOLFSSL_EVP_CIPHER_CTX* ctx); 00388 WOLFSSL_API int wolfSSL_SetInternalIV(WOLFSSL_EVP_CIPHER_CTX* ctx); 00389 00390 WOLFSSL_API int wolfSSL_EVP_CIPHER_CTX_block_size(const WOLFSSL_EVP_CIPHER_CTX *ctx); 00391 WOLFSSL_API int wolfSSL_EVP_CIPHER_block_size(const WOLFSSL_EVP_CIPHER *cipher); 00392 WOLFSSL_API unsigned long WOLFSSL_EVP_CIPHER_mode(const WOLFSSL_EVP_CIPHER *cipher); 00393 WOLFSSL_API unsigned long WOLFSSL_CIPHER_mode(const WOLFSSL_EVP_CIPHER *cipher); 00394 WOLFSSL_API unsigned long wolfSSL_EVP_CIPHER_flags(const WOLFSSL_EVP_CIPHER *cipher); 00395 WOLFSSL_API void wolfSSL_EVP_CIPHER_CTX_set_flags(WOLFSSL_EVP_CIPHER_CTX *ctx, int flags); 00396 WOLFSSL_API unsigned long wolfSSL_EVP_CIPHER_CTX_mode(const WOLFSSL_EVP_CIPHER_CTX *ctx); 00397 WOLFSSL_API int wolfSSL_EVP_CIPHER_CTX_set_padding(WOLFSSL_EVP_CIPHER_CTX *c, int pad); 00398 WOLFSSL_API int wolfSSL_EVP_add_digest(const WOLFSSL_EVP_MD *digest); 00399 WOLFSSL_API int wolfSSL_EVP_add_cipher(const WOLFSSL_EVP_CIPHER *cipher); 00400 WOLFSSL_API void wolfSSL_EVP_cleanup(void); 00401 WOLFSSL_API int wolfSSL_add_all_algorithms(void); 00402 00403 #ifdef OPENSSL_EXTRA 00404 WOLFSSL_API int wolfSSL_OPENSSL_add_all_algorithms_noconf(void); 00405 #endif 00406 00407 WOLFSSL_API int wolfSSL_PKCS5_PBKDF2_HMAC_SHA1(const char * pass, int passlen, 00408 const unsigned char * salt, 00409 int saltlen, int iter, 00410 int keylen, unsigned char *out); 00411 00412 #define EVP_CIPH_STREAM_CIPHER WOLFSSL_EVP_CIPH_STREAM_CIPHER 00413 #define EVP_CIPH_ECB_MODE WOLFSSL_EVP_CIPH_ECB_MODE 00414 #define EVP_CIPH_CBC_MODE WOLFSSL_EVP_CIPH_CBC_MODE 00415 #define EVP_CIPH_CFB_MODE WOLFSSL_EVP_CIPH_CFB_MODE 00416 #define EVP_CIPH_OFB_MODE WOLFSSL_EVP_CIPH_OFB_MODE 00417 #define EVP_CIPH_CTR_MODE WOLFSSL_EVP_CIPH_CTR_MODE 00418 #define EVP_CIPH_GCM_MODE WOLFSSL_EVP_CIPH_GCM_MODE 00419 #define EVP_CIPH_CCM_MODE WOLFSSL_EVP_CIPH_CCM_MODE 00420 00421 #define WOLFSSL_EVP_CIPH_MODE 0x0007 00422 #define WOLFSSL_EVP_CIPH_STREAM_CIPHER 0x0 00423 #define WOLFSSL_EVP_CIPH_ECB_MODE 0x1 00424 #define WOLFSSL_EVP_CIPH_CBC_MODE 0x2 00425 #define WOLFSSL_EVP_CIPH_CFB_MODE 0x3 00426 #define WOLFSSL_EVP_CIPH_OFB_MODE 0x4 00427 #define WOLFSSL_EVP_CIPH_CTR_MODE 0x5 00428 #define WOLFSSL_EVP_CIPH_GCM_MODE 0x6 00429 #define WOLFSSL_EVP_CIPH_CCM_MODE 0x7 00430 #define WOLFSSL_EVP_CIPH_NO_PADDING 0x100 00431 #define WOLFSSL_EVP_CIPH_TYPE_INIT 0xff 00432 00433 /* end OpenSSH compat */ 00434 00435 typedef WOLFSSL_EVP_MD EVP_MD; 00436 typedef WOLFSSL_EVP_CIPHER EVP_CIPHER; 00437 typedef WOLFSSL_EVP_MD_CTX EVP_MD_CTX; 00438 typedef WOLFSSL_EVP_CIPHER_CTX EVP_CIPHER_CTX; 00439 00440 #ifndef NO_MD4 00441 #define EVP_md4 wolfSSL_EVP_md4 00442 #endif 00443 #ifndef NO_MD5 00444 #define EVP_md5 wolfSSL_EVP_md5 00445 #endif 00446 #define EVP_sha1 wolfSSL_EVP_sha1 00447 #define EVP_dds1 wolfSSL_EVP_sha1 00448 #define EVP_sha224 wolfSSL_EVP_sha224 00449 #define EVP_sha256 wolfSSL_EVP_sha256 00450 #define EVP_sha384 wolfSSL_EVP_sha384 00451 #define EVP_sha512 wolfSSL_EVP_sha512 00452 #define EVP_ripemd160 wolfSSL_EVP_ripemd160 00453 00454 #define EVP_aes_128_cbc wolfSSL_EVP_aes_128_cbc 00455 #define EVP_aes_192_cbc wolfSSL_EVP_aes_192_cbc 00456 #define EVP_aes_256_cbc wolfSSL_EVP_aes_256_cbc 00457 #define EVP_aes_128_ecb wolfSSL_EVP_aes_128_ecb 00458 #define EVP_aes_192_ecb wolfSSL_EVP_aes_192_ecb 00459 #define EVP_aes_256_ecb wolfSSL_EVP_aes_256_ecb 00460 #define EVP_aes_128_ctr wolfSSL_EVP_aes_128_ctr 00461 #define EVP_aes_192_ctr wolfSSL_EVP_aes_192_ctr 00462 #define EVP_aes_256_ctr wolfSSL_EVP_aes_256_ctr 00463 #define EVP_des_cbc wolfSSL_EVP_des_cbc 00464 #define EVP_des_ecb wolfSSL_EVP_des_ecb 00465 #define EVP_des_ede3_cbc wolfSSL_EVP_des_ede3_cbc 00466 #define EVP_des_ede3_ecb wolfSSL_EVP_des_ede3_ecb 00467 #define EVP_rc4 wolfSSL_EVP_rc4 00468 #define EVP_idea_cbc wolfSSL_EVP_idea_cbc 00469 #define EVP_enc_null wolfSSL_EVP_enc_null 00470 00471 #define EVP_MD_size wolfSSL_EVP_MD_size 00472 #define EVP_MD_CTX_new wolfSSL_EVP_MD_CTX_new 00473 #define EVP_MD_CTX_create wolfSSL_EVP_MD_CTX_new 00474 #define EVP_MD_CTX_free wolfSSL_EVP_MD_CTX_free 00475 #define EVP_MD_CTX_destroy wolfSSL_EVP_MD_CTX_free 00476 #define EVP_MD_CTX_init wolfSSL_EVP_MD_CTX_init 00477 #define EVP_MD_CTX_cleanup wolfSSL_EVP_MD_CTX_cleanup 00478 #define EVP_MD_CTX_md wolfSSL_EVP_MD_CTX_md 00479 #define EVP_MD_CTX_type wolfSSL_EVP_MD_CTX_type 00480 #define EVP_MD_type wolfSSL_EVP_MD_type 00481 00482 #define EVP_DigestInit wolfSSL_EVP_DigestInit 00483 #define EVP_DigestInit_ex wolfSSL_EVP_DigestInit_ex 00484 #define EVP_DigestUpdate wolfSSL_EVP_DigestUpdate 00485 #define EVP_DigestFinal wolfSSL_EVP_DigestFinal 00486 #define EVP_DigestFinal_ex wolfSSL_EVP_DigestFinal_ex 00487 #define EVP_DigestSignInit wolfSSL_EVP_DigestSignInit 00488 #define EVP_DigestSignUpdate wolfSSL_EVP_DigestSignUpdate 00489 #define EVP_DigestSignFinal wolfSSL_EVP_DigestSignFinal 00490 #define EVP_BytesToKey wolfSSL_EVP_BytesToKey 00491 00492 #define EVP_get_cipherbyname wolfSSL_EVP_get_cipherbyname 00493 #define EVP_get_digestbyname wolfSSL_EVP_get_digestbyname 00494 00495 #define EVP_CIPHER_CTX_init wolfSSL_EVP_CIPHER_CTX_init 00496 #define EVP_CIPHER_CTX_cleanup wolfSSL_EVP_CIPHER_CTX_cleanup 00497 #define EVP_CIPHER_CTX_iv_length wolfSSL_EVP_CIPHER_CTX_iv_length 00498 #define EVP_CIPHER_CTX_key_length wolfSSL_EVP_CIPHER_CTX_key_length 00499 #define EVP_CIPHER_CTX_set_key_length wolfSSL_EVP_CIPHER_CTX_set_key_length 00500 #define EVP_CIPHER_CTX_mode wolfSSL_EVP_CIPHER_CTX_mode 00501 00502 #define EVP_CIPHER_iv_length wolfSSL_EVP_CIPHER_iv_length 00503 #define EVP_CIPHER_key_length wolfSSL_EVP_Cipher_key_length 00504 00505 #define EVP_CipherInit wolfSSL_EVP_CipherInit 00506 #define EVP_CipherInit_ex wolfSSL_EVP_CipherInit_ex 00507 #define EVP_EncryptInit wolfSSL_EVP_EncryptInit 00508 #define EVP_EncryptInit_ex wolfSSL_EVP_EncryptInit_ex 00509 #define EVP_DecryptInit wolfSSL_EVP_DecryptInit 00510 #define EVP_DecryptInit_ex wolfSSL_EVP_DecryptInit_ex 00511 00512 #define EVP_Cipher wolfSSL_EVP_Cipher 00513 #define EVP_CipherUpdate wolfSSL_EVP_CipherUpdate 00514 #define EVP_EncryptUpdate wolfSSL_EVP_CipherUpdate 00515 #define EVP_DecryptUpdate wolfSSL_EVP_CipherUpdate 00516 #define EVP_CipherFinal wolfSSL_EVP_CipherFinal 00517 #define EVP_CipherFinal_ex wolfSSL_EVP_CipherFinal 00518 #define EVP_EncryptFinal wolfSSL_EVP_CipherFinal 00519 #define EVP_EncryptFinal_ex wolfSSL_EVP_CipherFinal 00520 #define EVP_DecryptFinal wolfSSL_EVP_CipherFinal 00521 #define EVP_DecryptFinal_ex wolfSSL_EVP_CipherFinal 00522 00523 #define EVP_CIPHER_CTX_free wolfSSL_EVP_CIPHER_CTX_free 00524 #define EVP_CIPHER_CTX_new wolfSSL_EVP_CIPHER_CTX_new 00525 00526 #define EVP_get_cipherbynid wolfSSL_EVP_get_cipherbynid 00527 #define EVP_get_digestbynid wolfSSL_EVP_get_digestbynid 00528 #define EVP_get_cipherbyname wolfSSL_EVP_get_cipherbyname 00529 #define EVP_get_digestbyname wolfSSL_EVP_get_digestbyname 00530 00531 #define EVP_PKEY_get1_RSA wolfSSL_EVP_PKEY_get1_RSA 00532 #define EVP_PKEY_get1_DSA wolfSSL_EVP_PKEY_get1_DSA 00533 #define EVP_PKEY_set1_RSA wolfSSL_EVP_PKEY_set1_RSA 00534 #define EVP_PKEY_get1_EC_KEY wolfSSL_EVP_PKEY_get1_EC_KEY 00535 #define EVP_PKEY_get0_hmac wolfSSL_EVP_PKEY_get0_hmac 00536 #define EVP_PKEY_new_mac_key wolfSSL_EVP_PKEY_new_mac_key 00537 #define EVP_MD_CTX_copy wolfSSL_EVP_MD_CTX_copy 00538 #define EVP_MD_CTX_copy_ex wolfSSL_EVP_MD_CTX_copy_ex 00539 #define EVP_PKEY_bits wolfSSL_EVP_PKEY_bits 00540 #define EVP_PKEY_CTX_free wolfSSL_EVP_PKEY_CTX_free 00541 #define EVP_PKEY_CTX_new wolfSSL_EVP_PKEY_CTX_new 00542 #define EVP_PKEY_CTX_set_rsa_padding wolfSSL_EVP_PKEY_CTX_set_rsa_padding 00543 #define EVP_PKEY_decrypt wolfSSL_EVP_PKEY_decrypt 00544 #define EVP_PKEY_decrypt_init wolfSSL_EVP_PKEY_decrypt_init 00545 #define EVP_PKEY_encrypt wolfSSL_EVP_PKEY_encrypt 00546 #define EVP_PKEY_encrypt_init wolfSSL_EVP_PKEY_encrypt_init 00547 #define EVP_PKEY_new wolfSSL_PKEY_new 00548 #define EVP_PKEY_free wolfSSL_EVP_PKEY_free 00549 #define EVP_PKEY_size wolfSSL_EVP_PKEY_size 00550 #define EVP_PKEY_type wolfSSL_EVP_PKEY_type 00551 #define EVP_PKEY_base_id wolfSSL_EVP_PKEY_base_id 00552 #define EVP_SignFinal wolfSSL_EVP_SignFinal 00553 #define EVP_SignInit wolfSSL_EVP_SignInit 00554 #define EVP_SignUpdate wolfSSL_EVP_SignUpdate 00555 #define EVP_VerifyFinal wolfSSL_EVP_VerifyFinal 00556 #define EVP_VerifyInit wolfSSL_EVP_VerifyInit 00557 #define EVP_VerifyUpdate wolfSSL_EVP_VerifyUpdate 00558 00559 #define EVP_CIPHER_CTX_block_size wolfSSL_EVP_CIPHER_CTX_block_size 00560 #define EVP_CIPHER_block_size wolfSSL_EVP_CIPHER_block_size 00561 #define EVP_CIPHER_flags wolfSSL_EVP_CIPHER_flags 00562 #define EVP_CIPHER_CTX_set_flags wolfSSL_EVP_CIPHER_CTX_set_flags 00563 #define EVP_CIPHER_CTX_set_padding wolfSSL_EVP_CIPHER_CTX_set_padding 00564 #define EVP_CIPHER_CTX_flags wolfSSL_EVP_CIPHER_CTX_flags 00565 #define EVP_add_digest wolfSSL_EVP_add_digest 00566 #define EVP_add_cipher wolfSSL_EVP_add_cipher 00567 #define EVP_cleanup wolfSSL_EVP_cleanup 00568 00569 #define OpenSSL_add_all_digests() wolfCrypt_Init() 00570 #define OpenSSL_add_all_ciphers() wolfCrypt_Init() 00571 #define OpenSSL_add_all_algorithms wolfSSL_add_all_algorithms 00572 #define OPENSSL_add_all_algorithms_noconf wolfSSL_OPENSSL_add_all_algorithms_noconf 00573 00574 #define PKCS5_PBKDF2_HMAC_SHA1 wolfSSL_PKCS5_PBKDF2_HMAC_SHA1 00575 00576 #ifndef EVP_MAX_MD_SIZE 00577 #define EVP_MAX_MD_SIZE 64 /* sha512 */ 00578 #endif 00579 00580 #ifndef EVP_MAX_BLOCK_LENGTH 00581 #define EVP_MAX_BLOCK_LENGTH 32 /* 2 * blocklen(AES)? */ 00582 /* They define this as 32. Using the same value here. */ 00583 #endif 00584 00585 WOLFSSL_API void printPKEY(WOLFSSL_EVP_PKEY *k); 00586 00587 #ifdef __cplusplus 00588 } /* extern "C" */ 00589 #endif 00590 00591 00592 #endif /* WOLFSSL_EVP_H_ */ 00593
Generated on Wed Jul 13 2022 01:38:41 by
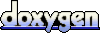