
USB to I2C converter
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut led(LED1); 00004 Serial pc(USBTX,USBRX); //UART0 via OpenSDA 00005 I2CSlave i2c(I2C_SDA, I2C_SCL); 00006 int BUFFER_SIZE = 2; 00007 00008 int main() { 00009 pc.baud(115200); 00010 pc.printf("%s\r\n", "ok"); 00011 led = 0; 00012 char buf[BUFFER_SIZE]; 00013 //pc.attach(pc_recv); 00014 i2c.frequency(400000); 00015 i2c.address(0x18); 00016 while(true) { 00017 for(int i = 0; i < BUFFER_SIZE; i++){ 00018 buf[i] = 0; 00019 } 00020 switch (i2c.receive()) { 00021 case I2CSlave::ReadAddressed: 00022 // Write back the buffer from the master 00023 i2c.write(buf, BUFFER_SIZE); 00024 pc.printf("Written to master (addressed): %s\r\n", buf); 00025 break; 00026 case I2CSlave::WriteGeneral: 00027 i2c.read(buf, BUFFER_SIZE); 00028 pc.printf("Read from master (general): %s\r\n", buf); 00029 break; 00030 case I2CSlave::WriteAddressed: 00031 i2c.read(buf, BUFFER_SIZE); 00032 pc.printf("Read from master (addressed): %d\r\n", buf[0]); 00033 for(int i = 1; i < BUFFER_SIZE; i++){ 00034 pc.printf("%d ", buf[i]); 00035 } 00036 pc.printf("\r\n"); 00037 break; 00038 } 00039 } 00040 }
Generated on Fri Jul 15 2022 05:26:37 by
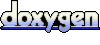