
Basis aansturing projectgroep 3
Dependencies: Biquad HIDScope MODSERIAL QEI mbed
BiQuad Class Reference
BiQuad class implements a single filter. More...
#include <BiQuad.h>
Public Member Functions | |
BiQuad () | |
Initialize a unity TF biquad. | |
BiQuad (double b0, double b1, double b2, double a1, double a2) | |
Initialize a normalized biquad filter. | |
BiQuad (double b0, double b1, double b2, double a0, double a1, double a2) | |
Initialize a biquad filter with all six coefficients. | |
void | PIDF (double Kp, double Ki, double Kd, double N, double Ts) |
Initialize a PIDF biquad. | |
double | step (double x) |
Execute one digital timestep and return the result... | |
std::vector< std::complex < double > > | poles () |
Return poles of the BiQuad filter. | |
std::vector< std::complex < double > > | zeros () |
Return zeros of the BiQuad filter. | |
bool | stable () |
Is this biquad stable? Checks if all poles lie within the unit-circle. | |
void | setResetStateOnGainChange (bool v) |
Determines if the state variables are reset to zero on gain change. |
Detailed Description
BiQuad class implements a single filter.
author: T.J.W. Lankhorst <t.j.w.lankhorst@student.utwente.nl>
Filters that - in the z domain - are the ratio of two quadratic functions. The general form is:
b0 + b1 z^-1 + b2 z^-2 H(z) = ---------------------- a0 + a1 z^-1 + a2 z^-2
Which is often normalized by dividing all coefficients by a0.
Example:
#include "mbed.h" #include <complex> // Example: 4th order Butterworth LP (w_c = 0.1*f_nyquist) BiQuad bq1( 4.16599e-04, 8.33198e-04, 4.16599e-04, -1.47967e+00, 5.55822e-01 ); BiQuad bq2( 1.00000e+00, 2.00000e+00, 1.00000e+00, -1.70096e+00, 7.88500e-01 ); BiQuadChain bqc; int main() { // Add the biquads to the chain bqc.add( &bq1 ).add( &bq2 ); // Find the poles of the filter std::cout << "Filter poles" << std::endl; std::vector< std::complex<double> > poles = bqc.poles(); for( size_t i = 0; i < poles.size(); i++ ) std::cout << "\t" << poles[i] << std::endl; // Find the zeros of the filter std::cout << "Filter zeros" << std::endl; std::vector< std::complex<double> > zeros = bqc.zeros(); for( size_t i = 0; i < poles.size(); i++ ) std::cout << "\t" << zeros[i] << std::endl; // Is the filter stable? std::cout << "This filter is " << (bqc.stable() ? "stable" : "instable") << std::endl; // Output the step-response of 20 samples std::cout << "Step response 20 samples" << std::endl; for( int i = 0; i < 20; i++ ) std::cout << "\t" << bqc.step( 1.0 ) << std::endl; }
https://github.com/tomlankhorst/biquad
Definition at line 62 of file BiQuad.h.
Constructor & Destructor Documentation
BiQuad | ( | ) |
BiQuad | ( | double | b0, |
double | b1, | ||
double | b2, | ||
double | a1, | ||
double | a2 | ||
) |
Initialize a normalized biquad filter.
- Parameters:
-
b0 b1 b2 a1 a2
- Returns:
- BiQuad instance
Definition at line 8 of file BiQuad.cpp.
BiQuad | ( | double | b0, |
double | b1, | ||
double | b2, | ||
double | a0, | ||
double | a1, | ||
double | a2 | ||
) |
Initialize a biquad filter with all six coefficients.
- Parameters:
-
b0 b1 b2 a0 a1 a2
- Returns:
- BiQuad instance
Definition at line 13 of file BiQuad.cpp.
Member Function Documentation
void PIDF | ( | double | Kp, |
double | Ki, | ||
double | Kd, | ||
double | N, | ||
double | Ts | ||
) |
Initialize a PIDF biquad.
Based on Tustin-approx (trapezoidal) of the continous time version. Behaviour equivalent to the PID controller created with the following MATLAB expression:
C = pid( Kp, Ki, Kd, 1/N, Ts, 'IFormula', 'Trapezoidal', 'DFormula', 'Trapezoidal' );
- Parameters:
-
Kp Proportional gain Ki Integral gain Kd Derivative gain N Filter coefficient ( N = 1/Tf ) Ts Timestep
Definition at line 18 of file BiQuad.cpp.
std::vector< std::complex< double > > poles | ( | ) |
Return poles of the BiQuad filter.
- Returns:
- vector of std::complex poles
Definition at line 61 of file BiQuad.cpp.
void setResetStateOnGainChange | ( | bool | v ) |
Determines if the state variables are reset to zero on gain change.
Can be used for changing gain parameters on the fly.
- Parameters:
-
v Value of the reset boolean
Definition at line 97 of file BiQuad.cpp.
bool stable | ( | ) |
Is this biquad stable? Checks if all poles lie within the unit-circle.
- Returns:
- boolean whether the filter is stable or not
Definition at line 89 of file BiQuad.cpp.
double step | ( | double | x ) |
Execute one digital timestep and return the result...
- Parameters:
-
x input of the filer
- Returns:
- output of the filter
Definition at line 45 of file BiQuad.cpp.
std::vector< std::complex< double > > zeros | ( | ) |
Return zeros of the BiQuad filter.
- Returns:
- vector of std::complex zeros
Definition at line 75 of file BiQuad.cpp.
Generated on Thu Jul 14 2022 07:24:36 by
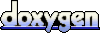