for ST7567 LCD
Fork of st7565LCD by
Embed:
(wiki syntax)
Show/hide line numbers
st7565LCD.h
00001 /* 00002 $Id:$ 00003 00004 ST7565 LCD library! 00005 00006 Copyright (C) 2010 Limor Fried, Adafruit Industries 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA 00021 00022 // some of this code was written by <cstone@pobox.com> originally; it is in the public domain. 00023 */ 00024 00025 00026 #ifndef _st7565LCD_H_ 00027 #define _st7565LCD_H_ 00028 00029 #include "mbed.h" 00030 00031 #define swap(a, b) { uint8_t t = a; a = b; b = t; } 00032 00033 #define BLACK 1 00034 #define WHITE 0 00035 00036 #define LCDWIDTH 128 00037 #define LCDHEIGHT 64 00038 00039 #define INITIAL_CONTRAST 0x18 00040 00041 #define CMD_DISPLAY_OFF 0xAE 00042 #define CMD_DISPLAY_ON 0xAF 00043 00044 #define CMD_SET_DISP_START_LINE 0x40 00045 #define CMD_SET_PAGE 0xB0 00046 00047 #define CMD_SET_COLUMN_UPPER 0x10 00048 #define CMD_SET_COLUMN_LOWER 0x00 00049 00050 #define CMD_SET_ADC_NORMAL 0xA0 00051 #define CMD_SET_ADC_REVERSE 0xA1 00052 00053 #define CMD_SET_DISP_NORMAL 0xA6 00054 #define CMD_SET_DISP_REVERSE 0xA7 00055 00056 #define CMD_SET_ALLPTS_NORMAL 0xA4 00057 #define CMD_SET_ALLPTS_ON 0xA5 00058 #define CMD_SET_BIAS_9 0xA2 00059 #define CMD_SET_BIAS_7 0xA3 00060 00061 #define CMD_RMW 0xE0 00062 #define CMD_RMW_CLEAR 0xEE 00063 #define CMD_INTERNAL_RESET 0xE2 00064 #define CMD_SET_COM_NORMAL 0xC0 00065 #define CMD_SET_COM_REVERSE 0xC8 00066 #define CMD_SET_POWER_CONTROL 0x28 00067 #define CMD_SET_RESISTOR_RATIO 0x20 00068 #define CMD_SET_VOLUME_FIRST 0x81 00069 #define CMD_SET_VOLUME_SECOND 0x00 00070 #define CMD_SET_STATIC_OFF 0xAC 00071 #define CMD_SET_STATIC_ON 0xAD 00072 #define CMD_SET_STATIC_REG 0x0 00073 #define CMD_SET_BOOSTER_FIRST 0xF8 00074 #define CMD_SET_BOOSTER_234 0 00075 #define CMD_SET_BOOSTER_5 1 00076 #define CMD_SET_BOOSTER_6 3 00077 #define CMD_NOP 0xE3 00078 #define CMD_TEST 0xF0 00079 00080 class ST7565 { 00081 00082 private: 00083 SPI _spi; 00084 DigitalOut _cs; 00085 DigitalOut _rst; 00086 DigitalOut _a0; 00087 void spiwrite(uint8_t c); 00088 void my_setpixel(uint8_t x, uint8_t y, uint8_t color); 00089 00090 //uint8_t buffer[128*64/8]; 00091 public: 00092 ST7565(PinName mosi, PinName sclk, PinName cs, PinName rst, PinName a0); 00093 ~ST7565() {}; 00094 void st7565_init(void); 00095 void begin(uint8_t contrast); 00096 void st7565_command(uint8_t c); 00097 void st7565_data(uint8_t c); 00098 void st7565_set_brightness(uint8_t val); 00099 void clear_display(void); 00100 void clear(); 00101 void display(); 00102 00103 void setpixel(uint8_t x, uint8_t y, uint8_t color); 00104 uint8_t getpixel(uint8_t x, uint8_t y); 00105 void fillcircle(uint8_t x0, uint8_t y0, uint8_t r, 00106 uint8_t color); 00107 void drawcircle(uint8_t x0, uint8_t y0, uint8_t r, 00108 uint8_t color); 00109 void drawrect(uint8_t x, uint8_t y, uint8_t w, uint8_t h, 00110 uint8_t color); 00111 void fillrect(uint8_t x, uint8_t y, uint8_t w, uint8_t h, 00112 uint8_t color); 00113 void drawline(uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1, 00114 uint8_t color); 00115 void drawchar(uint8_t x, uint8_t line, char c); 00116 void drawstring(uint8_t x, uint8_t line, char *c); 00117 /*void drawbitmap(uint8_t x, uint8_t y, 00118 const uint8_t *bitmap, uint8_t w, uint8_t h, 00119 uint8_t color);*/ 00120 uint8_t _BV(uint8_t bit); 00121 uint8_t _nBV(uint8_t bit); 00122 00123 void set_spi_frequency(int frequency); 00124 00125 }; 00126 00127 #endif
Generated on Fri Jul 29 2022 03:19:06 by
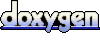