
Pot and LDR value Wifi logging TO Node-red and from there to local phant
Fork of ESP8266_LocalPhant_KL25Z by
main.cpp
00001 #include "mbed.h" 00002 #include "ESP8266.h" 00003 00004 Serial pc(USBTX,USBRX); 00005 00006 //POT sensor 00007 AnalogIn pot(PTB0); 00008 AnalogIn ldr(PTB1); 00009 00010 //wifi UART port and baud rate 00011 ESP8266 wifi(PTE0, PTE1, 115200); 00012 00013 //buffers for wifi library 00014 char snd[255],resp[1000]; 00015 char http_cmd[300], comm[300]; 00016 00017 int timeout = 5000; //timeout for wifi commands 00018 00019 //SSID and password for connection 00020 #define SSID "eduvanceAP" 00021 #define PASS "winteriscoming" 00022 00023 //Remote IP 00024 #define IP "192.168.0.22" 00025 00026 //ldrvalue global variable 00027 float ldrval = 0; 00028 float potval = 0; 00029 00030 //Public and private keys for phant 00031 //char* Public_Key = "eaWkxgdlQZszLEDKpy6OFeDeO8x"; 00032 //char* Private_Key = "25xqpAeBmzSml4Yjpr05Hj3jOBq"; 00033 00034 //Wifi init function 00035 void wifi_initialize(void){ 00036 00037 pc.printf("******** Resetting wifi module ********\r\n"); 00038 wifi.Reset(); 00039 00040 //wait for 5 seconds for response, else display no response receiveed 00041 if (wifi.RcvReply(resp, 8000)) 00042 pc.printf("%s",resp); 00043 else 00044 pc.printf("No response"); 00045 00046 pc.printf("******** Setting Station mode of wifi with AP ********\r\n"); 00047 wifi.SetMode(1); // set transparent mode 00048 if (wifi.RcvReply(resp, timeout)) //receive a response from ESP 00049 pc.printf("%s",resp); //Print the response onscreen 00050 else 00051 pc.printf("No response while setting mode. \r\n"); 00052 00053 pc.printf("******** Joining network with SSID and PASS ********\r\n"); 00054 wifi.Join(SSID, PASS); 00055 if (wifi.RcvReply(resp, timeout)) 00056 pc.printf("%s",resp); 00057 else 00058 pc.printf("No response while connecting to network \r\n"); 00059 00060 pc.printf("******** Getting IP and MAC of module ********\r\n"); 00061 wifi.GetIP(resp); 00062 if (wifi.RcvReply(resp, timeout)) 00063 pc.printf("%s",resp); 00064 else 00065 pc.printf("No response while getting IP \r\n"); 00066 00067 pc.printf("******** Setting WIFI UART passthrough ********\r\n"); 00068 wifi.setTransparent(); 00069 if (wifi.RcvReply(resp, timeout)) 00070 pc.printf("%s",resp); 00071 else 00072 pc.printf("No response while setting wifi passthrough. \r\n"); 00073 wait(1); 00074 00075 pc.printf("******** Setting single connection mode ********\r\n"); 00076 wifi.SetSingle(); 00077 wifi.RcvReply(resp, timeout); 00078 if (wifi.RcvReply(resp, timeout)) 00079 pc.printf("%s",resp); 00080 else 00081 pc.printf("No response while setting single connection \r\n"); 00082 wait(1); 00083 } 00084 00085 void wifi_send(void){ 00086 00087 pc.printf("******** Starting TCP connection on IP and port ********\r\n"); 00088 wifi.startTCPConn(IP, 1880); //cipstart 00089 wifi.RcvReply(resp, timeout); 00090 if (wifi.RcvReply(resp, timeout)) 00091 pc.printf("%s",resp); 00092 else 00093 pc.printf("No response while starting TCP connection \r\n"); 00094 wait(1); 00095 00096 //create link 00097 sprintf(http_cmd,"/log?pot=%0.2f&ldr=%0.2f",potval,ldrval); 00098 pc.printf(http_cmd); 00099 00100 pc.printf("******** Sending URL to wifi ********\r\n"); 00101 wifi.sendURL(http_cmd, comm); //cipsend and get command 00102 if (wifi.RcvReply(resp, timeout)) 00103 pc.printf("%s",resp); 00104 else 00105 pc.printf("No response while sending URL \r\n"); 00106 00107 //wifi.SendCMD("AT+CIPCLOSE"); //Close the connection to server 00108 //wifi.RcvReply(resp, timeout); 00109 //pc.printf("%s", resp); 00110 } 00111 00112 int main () { 00113 00114 00115 wifi_initialize(); 00116 00117 while (1) { 00118 potval = pot.read(); 00119 ldrval = ldr.read(); 00120 pc.printf("Potentiometer potvalue=%.3f & ldrvalue=%.3f\r\n",potval,ldrval); 00121 00122 wifi_send(); 00123 wait(3); 00124 } 00125 } 00126 00127
Generated on Tue Jul 12 2022 14:13:57 by
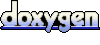