
Two player imu pong
Dependencies: 4DGL-uLCD-SE IMUfilter LSM9DS0 PinDetect mbed
tempModule.h
00001 #include "uLCD_4DGL.h" 00002 00003 /* Recommendation: 00004 * This class doesn't need to be declared outside the Ball class, 00005 * so you can create it inside the Ball class and use it only 00006 * in the Ball class. 00007 * 00008 * If the main function or multiple objects use a piece of hardware 00009 * or a class object (like uLCD or mySpeaker), you should pass a 00010 * pointer into the object rather than creating the object (either 00011 * in the stack or using new). 00012 */ 00013 00014 class TempModule 00015 { 00016 public: 00017 // Constructors 00018 TempModule(PinName pin); 00019 TempModule(PinName pin, float vx, float vy); 00020 // Reading temperature values 00021 float read(); 00022 // Set Functions 00023 void setBaseVx(float); // This sets the lowest velocity of vx 00024 void setBaseVy(float); // This sets the lowest velocity of vy 00025 // Get Functions/Member Functions 00026 float getVx(); // Calculates a speed based off of the temp 00027 float getVy(); 00028 float getVx(uLCD_4DGL *); // Same thing as getVx(), except it 00029 // also writes the temp to the screen. 00030 00031 private: 00032 // Data members are suggestions, feel free to add/remove 00033 AnalogIn _sensor; //_pin 00034 float roomTemp; 00035 float holdTemp; 00036 float basevx; 00037 float basevy; 00038 int counter; 00039 }; 00040 00041
Generated on Mon Jul 18 2022 23:37:14 by
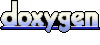