
Two player imu pong
Dependencies: 4DGL-uLCD-SE IMUfilter LSM9DS0 PinDetect mbed
paddle.h
00001 #include "uLCD_4DGL.h" 00002 00003 class Paddle 00004 { 00005 public: 00006 // Constructors 00007 Paddle(int, int); 00008 Paddle(int, int, int, int); 00009 // Set Functions 00010 void setLength(int); 00011 void setWidth(int); 00012 void setPaddleMove(int); 00013 void setLimits(int, int); // upper and lower limits of the paddle 00014 // Get Function 00015 int getScore(); 00016 // Member Functions 00017 void movePaddle(bool); // moves the paddle locations (does not draw!) 00018 bool checkHitX(int, int, int, bool); // Using a position and radius, checks to see if something has hit the paddle in the x direction. The bool is to determine if it is the left or right paddle 00019 bool checkHitY(int, int, int); // Using a position and radius, checks to see if something has hit the paddle in the y direction 00020 void resetScore(); // sets score to 0 00021 void initDraw(uLCD_4DGL *uLCD); // draw the paddle initially (draws the whole thing) 00022 void redraw(uLCD_4DGL *uLCD); // draws the paddle for a move (does NOT draw the whole thing) 00023 00024 00025 private: 00026 // Data members are suggestions, feel free to add/remove 00027 int score; 00028 int x; 00029 int y; 00030 int oldy; 00031 int length; 00032 int width; 00033 int paddleMove; 00034 int topLimit; 00035 int bottomLimit; 00036 }; 00037
Generated on Mon Jul 18 2022 23:37:14 by
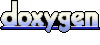