
Two player imu pong
Dependencies: 4DGL-uLCD-SE IMUfilter LSM9DS0 PinDetect mbed
main.cpp
00001 #include "mbed.h" 00002 #include "PinDetect.h" 00003 #include "uLCD_4DGL.h" 00004 #include "Speaker.h" 00005 #include "paddle.h" 00006 #include "ball.h" 00007 #include "soundBuilder.h" 00008 #include "LSM9DS0.h" 00009 00010 Serial pc(USBTX, USBRX); 00011 //IMU 00012 // SDO_XM and SDO_G are pulled up, so our addresses are: 00013 #define LSM9DS0_XM_ADDR 0x1D // Would be 0x1E if SDO_XM is LOW 00014 #define LSM9DS0_G_ADDR 0x6B // Would be 0x6A if SDO_G is LOW 00015 LSM9DS0 imu(p9, p10, LSM9DS0_G_ADDR, LSM9DS0_XM_ADDR); 00016 LSM9DS0 imu2(p28,p27, LSM9DS0_G_ADDR, LSM9DS0_XM_ADDR); 00017 00018 // Pushbuttons 00019 PinDetect pbUp(p15); 00020 PinDetect pbDown(p16); 00021 // uLCD 00022 uLCD_4DGL uLCD(p13, p14, p29); 00023 //Speaker 00024 Speaker mySpeaker(p21); 00025 00026 // Global variables needed for the push button interrupts 00027 Paddle paddle(40, 3, 118,1); 00028 Paddle paddle2(40,3, 8, 1); 00029 Ball ball(p19, 1.6,1.2,5,50,21); 00030 00031 // State machine definitions 00032 enum gameStateType {START, WAIT, GAME_SETUP, GAME, LOSE}; 00033 /* State Definitions: 00034 * START -- Creates the start screen 00035 * WAIT -- After the start screen, goes into wait where mbed spins and does nothing 00036 * GAME_SETUP -- Sets up one time things (like boarders, initializes beginning velocity 00037 * GAME -- When the user actually gets to play 00038 * LOSE -- clears the screen, prints you lose, waits, then goes back to start 00039 */ 00040 00041 // Global state machine variable (So that the pushbuttons can modify it) 00042 gameStateType gameState = START; 00043 00044 // Pushbutton callbacks 00045 // WARNING: Do not call to draw anything to the uLCD in these 00046 // as this will cause the uLCD to crash sometimes. Update positions 00047 // and draw elsewhere (like it's done here). 00048 // Only modify the logic inside the callback functions. 00049 void pbUp_hit_callback (void) 00050 { 00051 switch (gameState) 00052 { 00053 case WAIT: 00054 gameState = GAME_SETUP; 00055 break; 00056 case GAME: 00057 paddle.movePaddle(true); 00058 paddle2.movePaddle(true); 00059 break; 00060 } 00061 } 00062 00063 void pbDown_hit_callback (void) 00064 { 00065 switch (gameState) 00066 { 00067 case WAIT: 00068 gameState = GAME_SETUP; 00069 break; 00070 case GAME: 00071 paddle.movePaddle(false); 00072 paddle2.movePaddle(false); 00073 break; 00074 } 00075 } 00076 00077 int main() 00078 { 00079 00080 //IMU 00081 uint16_t status = imu.begin(); 00082 //Make sure communication is working 00083 pc.printf("LSM9DS0 WHO_AM_I's returned: 0x%X\n", status); 00084 pc.printf("Should be 0x49D4\n\n"); 00085 00086 uint16_t status2 = imu2.begin(); 00087 //Make sure communication is working 00088 pc.printf("LSM9DS0 #2 WHO_AM_I's returned: 0x%X\n", status2); 00089 pc.printf("Should be 0x49D4\n\n"); 00090 00091 00092 00093 float IMU_offset = 0.14; //detrmined empirically 00094 00095 // This is setting up the pushbuttons 00096 // Don't modify this code. 00097 pbUp.mode(PullUp); 00098 pbDown.mode(PullUp); 00099 wait(0.1); 00100 pbUp.attach_deasserted(&pbUp_hit_callback); 00101 pbDown.attach_deasserted(&pbDown_hit_callback); 00102 pbUp.setSampleFrequency(); 00103 pbDown.setSampleFrequency(); 00104 // Don't modify this code. 00105 00106 uLCD.display_control(PORTRAIT); 00107 uLCD.cls(); 00108 uLCD.baudrate(BAUD_3000000); 00109 uLCD.background_color(BLACK); 00110 00111 // Initialize all your variables outside the while/switch statement 00112 // to avoid compiler warning/errors 00113 int i = 0; 00114 int random; 00115 00116 //paddle stuff 00117 int pmoveMin = 25; 00118 int pmoveMax = 70; 00119 int pmove; 00120 00121 while (1) 00122 { 00123 switch (gameState) 00124 { 00125 case START: 00126 uLCD.cls(); 00127 uLCD.locate(0,0); 00128 uLCD.printf("Pong!!!\n\n"); 00129 uLCD.printf("Press Key to Start"); 00130 gameState = WAIT; 00131 ball.setX(50); 00132 ball.setY(50); 00133 break; 00134 case GAME_SETUP: 00135 uLCD.cls(); 00136 uLCD.line(0, 0, 127, 0, 0xCFB53B); 00137 uLCD.line(127, 0, 127, 127, 0xCFB53B); 00138 uLCD.line(127, 127, 0, 127, 0xCFB53B); 00139 uLCD.line(0, 127, 0, 0, 0xCFB53B); 00140 ball.setBaseVx(2.0); 00141 ball.setBaseVy(2.0); 00142 random = rand() % 1; 00143 ball.setVxSign(-1); ball.setVySign(((float)random - 0.5)*2); 00144 paddle.initDraw(&uLCD); 00145 paddle2.initDraw(&uLCD); 00146 00147 Note note1(4000.0, 0.7, 0.5); 00148 Note note2(3000.0, 0.3, 0.5); 00149 Note note3(2000.0, 0.3, 0.5); 00150 Note note4(1000.0, 0.7, 0.5); 00151 Note note5(2500.0, 0.3, 0.5); 00152 SoundBuilder sound(&mySpeaker); 00153 sound.addNote(note1); 00154 sound.addNote(note2); 00155 sound.addNote(note3); 00156 sound.addNote(note4); 00157 sound.addNote(note5); 00158 sound.playSong(); 00159 gameState = GAME; 00160 break; 00161 case GAME: 00162 if(ball.CheckHit()) 00163 { 00164 Note note(1000.0, 0.03, 0.5); 00165 SoundBuilder sound(&mySpeaker); 00166 sound.addNote(note); 00167 sound.playSong(); 00168 } 00169 00170 if (paddle.checkHitY(ball.getFutureX(), ball.getFutureY(), ball.getRadius())) 00171 { 00172 00173 ball.reverseYDirection(); 00174 } 00175 00176 if (paddle2.checkHitY(ball.getFutureX(), ball.getFutureY(), ball.getRadius())) 00177 { 00178 00179 ball.reverseYDirection(); 00180 } 00181 00182 if (ball.CheckLose()) 00183 {gameState = LOSE;} 00184 if (paddle.checkHitX(ball.getFutureX(), ball.getFutureY(), ball.getRadius(),false)) 00185 { 00186 Note note(4000.0, 0.03, 0.5); 00187 SoundBuilder sound(&mySpeaker); 00188 sound.addNote(note); 00189 sound.playSong(); 00190 00191 ball.reverseXDirection(); 00192 uLCD.locate(1,1); 00193 } 00194 00195 if (paddle2.checkHitX(ball.getFutureX(), ball.getFutureY(), ball.getRadius(),true)) 00196 { 00197 Note note(4000.0, 0.03, 0.5); 00198 SoundBuilder sound(&mySpeaker); 00199 sound.addNote(note); 00200 sound.playSong(); 00201 ball.reverseXDirection(); 00202 uLCD.locate(1,1); 00203 } 00204 00205 00206 ball.update(&uLCD); 00207 imu.readAccel(); 00208 00209 imu2.readAccel(); 00210 00211 if ( imu.ax + IMU_offset > 0.1) 00212 { 00213 paddle.movePaddle(true); 00214 } 00215 else if (imu.ax+ IMU_offset < -0.1) 00216 { 00217 paddle.movePaddle(false); 00218 } 00219 00220 if ( imu2.ax + IMU_offset > 0.1) 00221 { 00222 paddle2.movePaddle(true); 00223 } 00224 else if (imu2.ax+ IMU_offset < -0.1) 00225 { 00226 paddle2.movePaddle(false); 00227 } 00228 00229 // We can assume that these for loops are quick enough that the paddle will move only one interval. 00230 // These movements of the paddle have been optimized. Feel free to draw it out to see how it's been done. 00231 paddle.redraw(&uLCD); 00232 paddle2.redraw(&uLCD); 00233 break; 00234 case LOSE: 00235 uLCD.cls(); 00236 uLCD.printf("YOU LOSE D:"); 00237 Note note6(3000.0, 0.7, 0.5); 00238 Note note7(2000.0, 0.3, 0.5); 00239 Note note8(1000.0, 0.3, 0.5); 00240 Note note9(2300.0, 0.7, 0.5); 00241 Note note10(2500.0, 0.3, 0.5); 00242 SoundBuilder sound2(&mySpeaker); 00243 sound2.addNote(note6); 00244 sound2.addNote(note7); 00245 sound2.addNote(note8); 00246 sound2.addNote(note9); 00247 sound2.addNote(note10); 00248 sound2.playSong(); 00249 paddle.resetScore(); 00250 paddle2.resetScore(); 00251 wait(5.0); 00252 gameState = START; 00253 break; 00254 case WAIT: 00255 // Used to seed the rand() function so we don't get the same starting position every time. 00256 i++; 00257 break; 00258 } 00259 } 00260 }
Generated on Mon Jul 18 2022 23:37:14 by
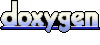