
Two player imu pong
Dependencies: 4DGL-uLCD-SE IMUfilter LSM9DS0 PinDetect mbed
ball.h
00001 #include "tempModule.h" 00002 #include "uLCD_4DGL.h" 00003 00004 class Ball 00005 { 00006 public: 00007 // Constructors 00008 //Ball (); 00009 // Ball (float, float, int); 00010 /* For Part 3: 00011 * you need to be able to pass a pin to the 00012 * tempModule object from main, so you should 00013 * add in a PinName argument. 00014 */ 00015 Ball(PinName); 00016 Ball(PinName, float, float, int, int, int); 00017 //*/ 00018 // Set Functions 00019 void setBaseVx(float); // This sets the lowest velocity of vx (for Thermal pong) or the constant velocity 00020 void setBaseVy(float); // This sets the lowest velocity of vy (for Thermal pong) or the constant velocity 00021 void setVxSign(int); 00022 void setVySign(int); 00023 void setX (int); 00024 void setY (int); 00025 void setRand(int seed); 00026 // Get Functions 00027 int getVx(); 00028 int getVy(); 00029 int getFutureX(); // get estimate of where the ball will be in the next update() 00030 int getFutureY(); // get estimate of where the ball will be in the next update() 00031 int getRadius(); 00032 // Member Functions 00033 void reverseXDirection(); // negate the sign for when a ball hits something 00034 void reverseYDirection(); // negate the sign for when a ball hits something 00035 //void reset(int, int, int, int, uLCD_4DGL *); // takes in a new location and new directions and draws the starting point for the ball 00036 void dispTemp(uLCD_4DGL *); 00037 void update(uLCD_4DGL *); // moves the ball on the screen one vx and vy 00038 bool CheckHit(); //check if the ball hit walls 00039 bool CheckLose(); //check if the ball is out of bounds 00040 private: 00041 // Data members are suggestions, feel free to add/remove 00042 TempModule *tempSensor; // Pointer -- it's recommended to use "new" operator to create this 00043 int vxSign; 00044 int vySign; 00045 float basevx; 00046 float basevy; 00047 float fx; 00048 float fy; 00049 int x; 00050 int y; 00051 int radius; 00052 bool lose; 00053 };
Generated on Mon Jul 18 2022 23:37:14 by
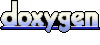