
Two player imu pong
Dependencies: 4DGL-uLCD-SE IMUfilter LSM9DS0 PinDetect mbed
Paddle.cpp
00001 #include "paddle.h" 00002 00003 00004 Paddle::Paddle(int len, int width) 00005 { 00006 setLength(len); 00007 setWidth(width); 00008 setPaddleMove(8); 00009 y = 1; 00010 x = 118; 00011 oldy = y; 00012 resetScore(); 00013 00014 00015 } 00016 00017 Paddle::Paddle(int len, int wid, int xi, int yi) 00018 { 00019 setLength(len); 00020 setWidth(wid); 00021 setPaddleMove(8); 00022 y = yi; 00023 x = xi; 00024 oldy = y; 00025 resetScore(); 00026 00027 } 00028 //set functions 00029 void Paddle::setLength(int len) 00030 { 00031 length = len; 00032 00033 } 00034 void Paddle::setWidth(int wid) 00035 { 00036 width = wid; 00037 } 00038 00039 void Paddle::setPaddleMove(int move) 00040 { 00041 paddleMove = move; 00042 } 00043 /* 00044 void Paddle::setLimits(int top, int bot) 00045 { 00046 topLimit = top; 00047 bottomLimit = bot; 00048 } 00049 */ 00050 // get Function 00051 int Paddle::getScore() 00052 { 00053 return score; 00054 00055 } 00056 //Member Functions 00057 void Paddle::movePaddle(bool mv) 00058 { 00059 if (mv) 00060 {if(y > paddleMove) 00061 {y -=paddleMove;} // end of inner if 00062 } // end of outer if 00063 else 00064 { 00065 if(y < 127 - paddleMove - length) 00066 { y+= paddleMove;} 00067 }//end of else 00068 }//ends function 00069 00070 bool Paddle::checkHitX(int xpos, int ypos, int radius, bool left) 00071 { 00072 00073 if (left) 00074 { 00075 if ((xpos <= x+radius+width) && (ypos <= y +length) && (ypos>=y)) 00076 { score = score +1; 00077 return true; 00078 } 00079 else 00080 { return false;} 00081 00082 } 00083 else { 00084 if ((xpos>= x-radius) && (ypos <= y +length) && (ypos>=y)) 00085 { score = score +1; 00086 return true; 00087 } 00088 else 00089 { return false;} 00090 } 00091 } 00092 00093 bool Paddle::checkHitY(int xpos, int ypos, int radius) 00094 { 00095 if (((xpos >= x) && (xpos <= x+3)) && 00096 ((ypos>=y) && (ypos<=y +length))) 00097 { return true;} 00098 else 00099 {return false;} 00100 } 00101 00102 void Paddle::resetScore() 00103 { score = 0; 00104 00105 } 00106 00107 void Paddle::initDraw(uLCD_4DGL *screen) 00108 { 00109 screen->filled_rectangle(x, y, x+width, y+length, BLUE); 00110 00111 } 00112 00113 void Paddle::redraw(uLCD_4DGL *uLCD) 00114 { 00115 if(oldy > y) { 00116 uLCD->filled_rectangle(x, oldy-paddleMove+1, x+width, oldy, BLUE); 00117 uLCD->filled_rectangle(x, oldy+length-paddleMove+1, x+width, oldy+length, BLACK); 00118 oldy = y; 00119 } 00120 else if(oldy < y) { 00121 uLCD->filled_rectangle(x, oldy, x+width, oldy+paddleMove, BLACK); 00122 uLCD->filled_rectangle(x, oldy+length,x+width, oldy+length+paddleMove, BLUE); 00123 oldy = y; 00124 } 00125 } 00126 00127
Generated on Mon Jul 18 2022 23:37:14 by
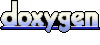