
mbed Window Dragging demo for TFT-320QVT module with SSD1289 and XPT2046 touchscreen. copyright GNU Public License, v2. or later Creates a window, which can be dragged within the screen, and can be closed with the standard WIndows-style "x"-button. Window appearance: initial position, size and colors can be changed via defines. Window in this demo can not be moved beyond the screen area except of the right side. May require a touchscreen calibration procedure using UTouchCD.h Made by Dmitry Shtatnov, SSSR Labs http://modularsynth.ru/
Dependencies: TFTLCD UTouch mbed
main.cpp
00001 /** \file main.cpp 00002 * \brief mbed Window Dragging demo for TFT-320QVT module with SSD1289 and XPT2046 touchscreen. 00003 * 00004 * \copyright GNU Public License, v2. or later 00005 * Creates a window, which can be dragged within the screen, and can be closed 00006 * with the standard WIndows-style "x"-button. 00007 * Window appearance: initial position, size and colors can be changed via defines 00008 * Window in this demo can not be moved beyond the screen area except of the right side. 00009 * May require a touchscreen calibration procedure using UTouchCD.h 00010 * 00011 * Made by Dmitry Shtatnov, SSSR Labs 00012 * http://modularsynth.ru/ 00013 ***************************************************************************************/ 00014 00015 #include "mbed.h" 00016 #include "ssd1289.h" 00017 #include "UTouch.h" 00018 00019 #define C_WINDOW_BODY 0xCCCCCC 00020 #define C_WINDOW_HIGHLIGHT 0xDDDDDD 00021 #define C_WINDOW_SHADOW 0xBBBBBB 00022 #define C_WINDOW_TITLE_BAR 0x0066DD 00023 00024 #define WINDOW_WIDTH 150 00025 #define WINDOW_HEIGHT 100 00026 00027 PortOut LCDPA(PortA,0xFF00); 00028 PortOut LCDPC(PortC,0x00FF); 00029 // CS, REST, RS, WR, DATA, BL, RD 00030 SSD1289_LCD myGLCD(PB_3, PB_4, PB_0, PB_1, &LCDPA, &LCDPC, NC, PB_2); 00031 00032 UTouch myTouch(PB_9, PB_8, PB_7, PB_6, PB_5); 00033 long x, y, oldx, oldy, wx = 100, wy = 100; 00034 float p; 00035 uint8_t av_cnt; 00036 int16_t rx, ry, rz1, rz2, pressure; 00037 char xc[10], yc[10], z1c[10], z2c[10], str[15]; 00038 bool drag = false; 00039 00040 bool title(uint16_t xcur, uint16_t ycur, uint16_t xwin, uint16_t ywin) { 00041 if((xcur>xwin+2)&&(xcur<xwin+WINDOW_WIDTH-18)&&(ycur>ywin+2)&&(ycur<ywin+22)) return true; 00042 else return false; 00043 } 00044 00045 bool close(uint16_t xcur, uint16_t ycur, uint16_t xwin, uint16_t ywin) { 00046 if((xcur>xwin+WINDOW_WIDTH-19)&&(xcur<xwin+WINDOW_WIDTH-1)&&(ycur>ywin+2)&&(ycur<ywin+21)) return true; 00047 else return false; 00048 } 00049 00050 void drawBox(uint16_t xpos, uint16_t ypos, uint16_t xsize, uint16_t ysize) { 00051 myGLCD.FillRect(xpos,ypos,xpos+xsize-1,ypos+ysize-1,C_WINDOW_BODY); 00052 myGLCD.DrawHLine(xpos,ypos,xsize,C_WINDOW_HIGHLIGHT); 00053 myGLCD.DrawHLine(xpos+1,ypos+ysize-1,xsize-1,C_WINDOW_SHADOW); 00054 myGLCD.DrawVLine(xpos,ypos,ysize,C_WINDOW_HIGHLIGHT); 00055 myGLCD.DrawVLine(xpos+xsize-1,ypos,ysize-1,C_WINDOW_SHADOW); 00056 } 00057 00058 void drawWindow(uint16_t xpos, uint16_t ypos, uint16_t xsize, uint16_t ysize) { 00059 drawBox(xpos, ypos, xsize, ysize); 00060 myGLCD.FillRect(xpos+2,ypos+2,xpos+xsize-3,ypos+21,C_WINDOW_TITLE_BAR); 00061 drawBox(xpos+xsize-21,ypos+3,18,18); 00062 myGLCD.DrawLine(xpos+xsize-18,ypos+6,xpos+xsize-7,ypos+17,COLOR_BLACK); 00063 myGLCD.DrawLine(xpos+xsize-18,ypos+17,xpos+xsize-7,ypos+6,COLOR_BLACK); 00064 } 00065 int main() { 00066 myGLCD.Initialize(); 00067 myGLCD.SetBackground(COLOR_WHITE); 00068 myGLCD.SetForeground(COLOR_BLACK); 00069 myGLCD.FillScreen(COLOR_WHITE); 00070 00071 drawWindow(wx,wy,WINDOW_WIDTH,WINDOW_HEIGHT); 00072 00073 myGLCD.SetFont(&TerminusFont); 00074 myTouch.InitTouch(); 00075 myTouch.SetPrecision(PREC_HI); 00076 00077 while(1==1) { 00078 if (myTouch.DataAvailable() == true) 00079 { 00080 if(myTouch.Read()) { 00081 x = myTouch.GetX(); 00082 y = myTouch.GetY(); 00083 if((oldx!=x)&&(oldy!=y)) { 00084 if(drag) { 00085 myGLCD.FillRect(wx, wy, wx+WINDOW_WIDTH-1, wy+WINDOW_HEIGHT-1, COLOR_WHITE); 00086 wx=wx+x-oldx; 00087 wy=wy+y-oldy; 00088 drawWindow(wx,wy,WINDOW_WIDTH,WINDOW_HEIGHT); 00089 } 00090 if(title(x, y, wx, wy)) { 00091 drag = true; 00092 } 00093 if(close(x, y, wx, wy)) { 00094 myGLCD.FillRect(wx, wy, wx+WINDOW_WIDTH-1, wy+WINDOW_HEIGHT-1, COLOR_WHITE); 00095 wx=320; wy=240; 00096 } 00097 oldx = x; 00098 oldy = y; 00099 } 00100 } 00101 } 00102 else { 00103 drag = false; 00104 } 00105 } 00106 }
Generated on Sun Jul 17 2022 11:06:57 by
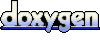