Block device class for creating a block device to access a SD/MMC card via SD/MMC interface on DISCO_F746NG
Dependencies: BSP_DISCO_F746NG
Dependents: DISCO-F746NG_BLOCK_DEVICE_SDCARD DISCO-F746NG_BLOCK_DEVICE_WITH_FAT_FILESYSTEM_ON_SDCARD SDReaderTest
SDBlockDeviceDISCOF746NG Class Reference
BD_SD_DISCO_F746NG class. More...
#include <SDBlockDeviceDISCOF746NG.h>
Public Member Functions | |
SDBlockDeviceDISCOF746NG () | |
Lifetime of the memory block device. | |
virtual int | init () |
Initialize a block device. | |
virtual int | deinit () |
Deinitialize a block device. | |
virtual int | read (void *buffer, bd_addr_t addr, bd_size_t size) |
Read blocks from a block device. | |
virtual int | program (const void *buffer, bd_addr_t addr, bd_size_t size) |
Program blocks to a block device. | |
virtual int | erase (bd_addr_t addr, bd_size_t size) |
Erase blocks on a block device. | |
virtual bd_size_t | get_read_size () const |
Get the size of a readable block. | |
virtual bd_size_t | get_program_size () const |
Get the size of a programable block. | |
virtual bd_size_t | get_erase_size () const |
Get the size of a eraseable block. | |
virtual bd_size_t | size () const |
Get the total size of the underlying device. |
Detailed Description
BD_SD_DISCO_F746NG class.
Block device class for creating a block device to access a SD/MMC card via SD/MMC interface on DISCO_F746NG
Example:
#include "mbed.h" #include "SDBlockDeviceDISCOF746NG.h" DigitalOut led (LED1); // Instantiate the Block Device for sd card on DISCO-F746NG SDBlockDeviceDISCOF746NG bd; uint8_t block[512] = "Hello World!\n"; int main () { Serial pc(SERIAL_TX, SERIAL_RX); pc.baud (115200); printf("Start\n"); // Call the SDBlockDevice-DISCO-F746NG instance initialisation method. printf("sd card init...\n"); if (0 != bd.init ()) { printf("Init failed \n"); return -1; } printf("sd size: %llu\n", bd.size ()); printf("sd read size: %llu\n", bd.get_read_size ()); printf("sd program size: %llu\n", bd.get_program_size ()); printf("sd erase size: %llu\n\n", bd.get_erase_size ()); printf("sd erase...\n"); if (0 != bd.erase (0, bd.get_erase_size ())) { printf ("Error Erasing block \n"); } // Write some the data block to the device printf("sd write: %s\n", block); if (0 == bd.program (block, 0, 512)) { // read the data block from the device printf ("sd read: "); if (0 == bd.read (block, 0, 512)) { // print the contents of the block printf ("%s", block); } } // Call the BD_SD_DISCO_F746NG instance de-initialisation method. printf("sd card deinit...\n"); if(0 != bd.deinit ()) { printf ("Deinit failed \n"); return -1; } // Blink led with 2 Hz while(true) { led = !led; wait (0.5); } }
Definition at line 95 of file SDBlockDeviceDISCOF746NG.h.
Constructor & Destructor Documentation
Lifetime of the memory block device.
Only a block size of 512 bytes is supported
Definition at line 58 of file SDBlockDeviceDISCOF746NG.cpp.
Member Function Documentation
int deinit | ( | ) | [virtual] |
Deinitialize a block device.
- Returns:
- 0 on success or a negative error code on failure
Definition at line 113 of file SDBlockDeviceDISCOF746NG.cpp.
int erase | ( | bd_addr_t | addr, |
bd_size_t | size | ||
) | [virtual] |
Erase blocks on a block device.
The state of an erased block is undefined until it has been programmed
- Parameters:
-
addr Address of block to begin erasing size Size to erase in bytes, must be a multiple of erase block size
- Returns:
- 0 on success, negative error code on failure
Definition at line 201 of file SDBlockDeviceDISCOF746NG.cpp.
bd_size_t get_erase_size | ( | ) | const [virtual] |
Get the size of a eraseable block.
- Returns:
- Size of a eraseable block in bytes
Definition at line 251 of file SDBlockDeviceDISCOF746NG.cpp.
bd_size_t get_program_size | ( | ) | const [virtual] |
Get the size of a programable block.
- Returns:
- Size of a programable block in bytes
Definition at line 246 of file SDBlockDeviceDISCOF746NG.cpp.
bd_size_t get_read_size | ( | ) | const [virtual] |
Get the size of a readable block.
- Returns:
- Size of a readable block in bytes
Definition at line 241 of file SDBlockDeviceDISCOF746NG.cpp.
int init | ( | ) | [virtual] |
Initialize a block device.
- Returns:
- 0 on success or a negative error code on failure
Definition at line 73 of file SDBlockDeviceDISCOF746NG.cpp.
int program | ( | const void * | buffer, |
bd_addr_t | addr, | ||
bd_size_t | size | ||
) | [virtual] |
Program blocks to a block device.
The blocks must have been erased prior to being programmed
- Parameters:
-
buffer Buffer of data to write to blocks addr Address of block to begin writing to size Size to write in bytes, must be a multiple of program block size
- Returns:
- 0 on success, negative error code on failure
Definition at line 163 of file SDBlockDeviceDISCOF746NG.cpp.
int read | ( | void * | buffer, |
bd_addr_t | addr, | ||
bd_size_t | size | ||
) | [virtual] |
Read blocks from a block device.
- Parameters:
-
buffer Buffer to read blocks into addr Address of block to begin reading from size Size to read in bytes, must be a multiple of read block size
- Returns:
- 0 on success, negative error code on failure
Definition at line 126 of file SDBlockDeviceDISCOF746NG.cpp.
bd_size_t size | ( | ) | const [virtual] |
Get the total size of the underlying device.
- Returns:
- Size of the underlying device in bytes
Definition at line 256 of file SDBlockDeviceDISCOF746NG.cpp.
Generated on Tue Jul 12 2022 19:08:01 by
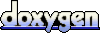