Routines to drive a chain of APA102 Leds.
Dependents: blink_led_threading
APA102.h
00001 #ifndef MBED_APA102_H 00002 00003 #define MBED_PING_H 00004 00005 #include "mbed.h" 00006 00007 00008 00009 /** Create an APA102 Object 00010 */ 00011 class APA102{ 00012 public: 00013 /** Create an APA102 object connected to the specified mosi,miso,sclk pins 00014 * 00015 * @param mosi : SPI Master Out Slave In pin 00016 * @param miso : SPI Master In Slave Out pin (ignored) 00017 * @param sclk : SPI Clock 00018 * @param rate : SPI Rate 00019 */ 00020 APA102(PinName mosi,PinName miso,PinName sclk,int rate); 00021 00022 /** Set the Buffer 00023 * 00024 * @param buffer[] : a buffer of unsigned integers (4 bytes) *Rows*Stride in size 00025 * @param Rows : Number of Rows 00026 * @param Cols : Number of Columns 00027 * @param Stride : The actual number of columns (useful for data alignment) 00028 * @param Offset : The offset into a row 00029 * @param ZigZag : A boolean, do we alternate count up/ count down per row? 00030 * @param Wrap : A boolean, do we wrap if (Offset+Cols) > Stride? (handy for scrolling messages) 00031 */ 00032 void SetBuffer(unsigned int Buffer[],int Rows,int Cols, int Stride,int Offset, bool ZigZag,bool Wrap); 00033 00034 /** Repaint the Strip 00035 * 00036 * @param none 00037 */ 00038 void Repaint(); 00039 00040 /** Create an IRGB helper function to construct a 4 byte LED Frame 00041 * 00042 * @param I : 5 bits of intensity (0,1,2,...,31) 00043 * @param R : 8 bits of Red (0,1,...,255) 00044 * @param G : 8 bits of Green (0,1,...,255) 00045 * @param B : 8 bits of Blue (0,1,...,255) 00046 */ 00047 00048 int IRGB(unsigned char I,unsigned char R,unsigned char G,unsigned char B) { 00049 return ((0xE0 + 0x1F&I)<<24)|((0xFF&R)<<16)|((0xFF&G)<<8)|(0xFF&B);} 00050 00051 protected: 00052 00053 SPI _spi; 00054 private: 00055 int NR; 00056 int NC; 00057 int NS; 00058 int off; 00059 bool ZF; 00060 bool WF; 00061 unsigned int * Buf; 00062 }; 00063 00064 #endif
Generated on Sun Jul 17 2022 00:46:40 by
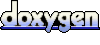