Morse Encoder/Decoder Library. Transforms char array to binary array and vice-versa.
Embed:
(wiki syntax)
Show/hide line numbers
Morse.h
00001 /* 00002 Copyright (c) 2014 Romain Berrada 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 furnished to do so, subject to the following conditions: 00009 00010 The above copyright notice and this permission notice shall be included in all copies or 00011 substantial portions of the Software. 00012 00013 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #ifndef INCLUDE_MORSE_H 00021 #define INCLUDE_MORSE_H 00022 00023 /** A Morse Encoding/Decoding Library \n 00024 Transforms char arrays into bool arrays and vice-versa 00025 00026 Morse code taken from http://en.wikipedia.org/wiki/Morse_code \n 00027 Added some more characters : \n 00028 minus : DOT DOT DASH DASH \n 00029 _ : DASH DASH DASH DOT \n 00030 . : DASH DASH DASH DASH \n 00031 / : DOT DASH DOT DASH \n 00032 @ : DOT DOT DOT DASH DOT \n 00033 ? : DOT DOT DASH DOT DOT \n 00034 00035 Here is an quick hello-world that show how to use this library \n 00036 @code 00037 #include "mbed.h 00038 #include "Morse.h" 00039 00040 Serial pc(USBTX, USBRX); 00041 00042 int main() { 00043 int i; 00044 Morse_data* data; 00045 char message[] = "Hello World"; 00046 00047 data = morse_create(morse_getBoolSize(message)); 00048 morse_encode(message, data); 00049 for (i=0; i<data->length; i++) pc.printf("%d", data->data[i]); 00050 00051 morse_decode(data, message); 00052 pc.printf("\nMessage was : %s\n", message); 00053 00054 while(1); 00055 } 00056 @endcode 00057 */ 00058 00059 /** Morse_data Structure 00060 */ 00061 typedef struct { 00062 int length; /**< Length of the data field */ 00063 bool* data; /**< data field containing 'length' booleans */ 00064 } Morse_data; 00065 00066 /** Give the size of the Morse_data that would be encoded from this word. 00067 @param word the word. 00068 @return the size of the encoded version of the word. 00069 */ 00070 unsigned int morse_getBoolSize(char* word); 00071 /** Give the size of the char array that would be decoded from this Morse_data. 00072 @param data the data. 00073 @return the size of the decoded version of the data. 00074 */ 00075 unsigned int morse_getWordSize(Morse_data* data); 00076 00077 /** Encode the char array and put the result into the Morse_data structure. 00078 @param word the word to encode. 00079 @param data the Morse_data structure to store the result. This structure must have a sufficient size. 00080 */ 00081 void morse_encode(char* word, Morse_data* data); 00082 /** Decode the Morse_data structure and put the result into the char array. 00083 @param data the data to decode. 00084 @param word the char array to store the result. The array must have a sufficient size. (Also think of the '\0') 00085 */ 00086 void morse_decode(Morse_data* data, char* word); 00087 00088 /** Allocate a Morse_data structure with the given size. 00089 @param length the size of the structure to create. 00090 @return the data pointer. 00091 */ 00092 Morse_data* morse_create(unsigned int length); 00093 /** Free a Morse_data structure. 00094 @param data the data to free. 00095 */ 00096 void morse_destroy(Morse_data* data); 00097 00098 #endif
Generated on Sun Jul 31 2022 00:23:01 by
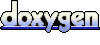