Morse Encoder/Decoder Library. Transforms char array to binary array and vice-versa.
Embed:
(wiki syntax)
Show/hide line numbers
MorseEncoder.cpp
00001 /* 00002 Copyright (c) 2014 Romain Berrada 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 furnished to do so, subject to the following conditions: 00009 00010 The above copyright notice and this permission notice shall be included in all copies or 00011 substantial portions of the Software. 00012 00013 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #include "Morse.h" 00021 00022 // Definitions of encoder/decoder data and Morse letter definitions 00023 #define DOT false 00024 #define DASH true 00025 00026 #define MORSE_MN {DOT, DOT, DASH, DASH, 0 } 00027 #define MORSE_DO {DASH, DASH, DASH, DASH, 0 } 00028 #define MORSE_SL {DOT, DASH, DOT, DASH, 0 } 00029 #define MORSE_0 {DASH, DASH, DASH, DASH, DASH} 00030 #define MORSE_1 {DOT, DASH, DASH, DASH, DASH} 00031 #define MORSE_2 {DOT, DOT, DASH, DASH, DASH} 00032 #define MORSE_3 {DOT, DOT, DOT, DASH, DASH} 00033 #define MORSE_4 {DOT, DOT, DOT, DOT, DASH} 00034 #define MORSE_5 {DOT, DOT, DOT, DOT, DOT } 00035 #define MORSE_6 {DASH, DOT, DOT, DOT, DOT } 00036 #define MORSE_7 {DASH, DASH, DOT, DOT, DOT } 00037 #define MORSE_8 {DASH, DASH, DASH, DOT, DOT } 00038 #define MORSE_9 {DASH, DASH, DASH, DASH, DOT } 00039 #define MORSE_IN {DOT, DOT, DASH, DOT, DOT } 00040 #define MORSE_AR {DOT, DOT, DOT, DASH, DOT } 00041 #define MORSE_A {DOT, DASH, 0, 0, 0 } 00042 #define MORSE_B {DASH, DOT, DOT, DOT, 0 } 00043 #define MORSE_C {DASH, DOT, DASH, DOT, 0 } 00044 #define MORSE_D {DASH, DOT, DOT, 0, 0 } 00045 #define MORSE_E {DOT, 0, 0, 0, 0 } 00046 #define MORSE_F {DOT, DOT, DASH, DOT, 0 } 00047 #define MORSE_G {DASH, DASH, DOT, 0, 0 } 00048 #define MORSE_H {DOT, DOT, DOT, DOT, 0 } 00049 #define MORSE_I {DOT, DOT, 0, 0, 0 } 00050 #define MORSE_J {DOT, DASH, DASH, DASH, 0 } 00051 #define MORSE_K {DASH, DOT, DASH, 0, 0 } 00052 #define MORSE_L {DOT, DASH, DOT, DOT, 0 } 00053 #define MORSE_M {DASH, DASH, 0, 0, 0 } 00054 #define MORSE_N {DASH, DOT, 0, 0, 0 } 00055 #define MORSE_O {DASH, DASH, DASH, 0, 0 } 00056 #define MORSE_P {DOT, DASH, DASH, DOT, 0 } 00057 #define MORSE_Q {DASH, DASH, DOT, DASH, 0 } 00058 #define MORSE_R {DOT, DASH, DOT, 0, 0 } 00059 #define MORSE_S {DOT, DOT, DOT, 0, 0 } 00060 #define MORSE_T {DASH, 0, 0, 0, 0 } 00061 #define MORSE_U {DOT, DOT, DASH, 0, 0 } 00062 #define MORSE_V {DOT, DOT, DOT, DASH, 0 } 00063 #define MORSE_W {DOT, DASH, DASH, 0 , 0 } 00064 #define MORSE_X {DASH, DOT, DOT, DASH, 0 } 00065 #define MORSE_Y {DASH, DOT, DASH, DASH, 0 } 00066 #define MORSE_Z {DASH, DASH, DOT, DOT, 0 } 00067 #define MORSE_UN {DASH, DASH, DASH, DOT, 0 } 00068 00069 #define MORSE_MN_SIZE 11 00070 #define MORSE_DO_SIZE 15 00071 #define MORSE_SL_SIZE 11 00072 #define MORSE_0_SIZE 19 00073 #define MORSE_1_SIZE 17 00074 #define MORSE_2_SIZE 15 00075 #define MORSE_3_SIZE 13 00076 #define MORSE_4_SIZE 11 00077 #define MORSE_5_SIZE 9 00078 #define MORSE_6_SIZE 11 00079 #define MORSE_7_SIZE 13 00080 #define MORSE_8_SIZE 15 00081 #define MORSE_9_SIZE 17 00082 #define MORSE_IN_SIZE 11 00083 #define MORSE_AR_SIZE 11 00084 #define MORSE_A_SIZE 5 00085 #define MORSE_B_SIZE 9 00086 #define MORSE_C_SIZE 11 00087 #define MORSE_D_SIZE 7 00088 #define MORSE_E_SIZE 1 00089 #define MORSE_F_SIZE 9 00090 #define MORSE_G_SIZE 9 00091 #define MORSE_H_SIZE 7 00092 #define MORSE_I_SIZE 3 00093 #define MORSE_J_SIZE 13 00094 #define MORSE_K_SIZE 9 00095 #define MORSE_L_SIZE 9 00096 #define MORSE_M_SIZE 7 00097 #define MORSE_N_SIZE 5 00098 #define MORSE_O_SIZE 11 00099 #define MORSE_P_SIZE 11 00100 #define MORSE_Q_SIZE 13 00101 #define MORSE_R_SIZE 7 00102 #define MORSE_S_SIZE 5 00103 #define MORSE_T_SIZE 3 00104 #define MORSE_U_SIZE 7 00105 #define MORSE_V_SIZE 9 00106 #define MORSE_W_SIZE 9 00107 #define MORSE_X_SIZE 11 00108 #define MORSE_Y_SIZE 13 00109 #define MORSE_Z_SIZE 11 00110 #define MORSE_UN_SIZE 13 00111 00112 // Array containing the morse letter definitions and the size of each letter (size is in bits) 00113 // Example: A = DOT-DASH. 00114 // DOT has size 1 and DASH 3. Plus the blank between the DOT and the DASH 00115 // So A has size 1+3+1 = 5 00116 static const unsigned int _morse_values_size[42] = {MORSE_MN_SIZE, MORSE_DO_SIZE, MORSE_SL_SIZE, MORSE_0_SIZE, MORSE_1_SIZE, MORSE_2_SIZE, 00117 MORSE_3_SIZE, MORSE_4_SIZE, MORSE_5_SIZE, MORSE_6_SIZE, MORSE_7_SIZE, MORSE_8_SIZE, 00118 MORSE_9_SIZE, MORSE_IN_SIZE, MORSE_AR_SIZE, MORSE_A_SIZE, MORSE_B_SIZE, MORSE_C_SIZE, 00119 MORSE_D_SIZE, MORSE_E_SIZE, MORSE_F_SIZE, MORSE_G_SIZE, MORSE_H_SIZE, MORSE_I_SIZE, 00120 MORSE_J_SIZE, MORSE_K_SIZE, MORSE_L_SIZE, MORSE_M_SIZE, MORSE_N_SIZE, MORSE_O_SIZE, 00121 MORSE_P_SIZE, MORSE_Q_SIZE, MORSE_R_SIZE, MORSE_S_SIZE, MORSE_T_SIZE, MORSE_U_SIZE, 00122 MORSE_V_SIZE, MORSE_W_SIZE, MORSE_X_SIZE, MORSE_Y_SIZE, MORSE_Z_SIZE, MORSE_UN_SIZE}; 00123 00124 static const bool _morse_values[42][5] = {MORSE_MN, MORSE_DO, MORSE_SL, MORSE_0, MORSE_1, MORSE_2, 00125 MORSE_3, MORSE_4, MORSE_5, MORSE_6, MORSE_7, MORSE_8, 00126 MORSE_9, MORSE_IN, MORSE_AR, MORSE_A, MORSE_B, MORSE_C, 00127 MORSE_D, MORSE_E, MORSE_F, MORSE_G, MORSE_H, MORSE_I, 00128 MORSE_J, MORSE_K, MORSE_L, MORSE_M, MORSE_N, MORSE_O, 00129 MORSE_P, MORSE_Q, MORSE_R, MORSE_S, MORSE_T, MORSE_U, 00130 MORSE_V, MORSE_W, MORSE_X, MORSE_Y, MORSE_Z, MORSE_UN}; 00131 00132 // Returns the index of the letter in the above arrays. returns -1 if not found 00133 static int _morse_find(char c) { 00134 int i=-1; 00135 if (c>='-' && c<='9') i=c-'-'; 00136 else if (c>='?' && c<='Z') i=c-'?'+13; 00137 else if (c>='a' && c<='z') i=c-'a'+15; 00138 else if (c=='_') i=41; 00139 return i; 00140 } 00141 00142 // Add a single char (index array) to the result array at the specified position 00143 static unsigned int _morse_addChar(unsigned int index_value, bool* result, unsigned int place) { 00144 unsigned char i,j; 00145 i=0; 00146 for (j=0; j<_morse_values_size[index_value]; j++) { 00147 if (_morse_values[index_value][i]==DOT) { // if DOT 00148 result[place+j] = true; 00149 } else { // else DASH 00150 result[place+j] = true; 00151 result[place+j+1]=true; 00152 result[place+j+2]=true; 00153 j+=2; 00154 } 00155 j++; // add a blank 00156 i++; // next element 00157 } 00158 00159 return j-1; 00160 } 00161 00162 unsigned int morse_getBoolSize(char* word) { 00163 unsigned int char_i; 00164 int morse_i; 00165 unsigned int size = 0; 00166 00167 for (char_i=0; word[char_i]!='\0'; char_i++) { 00168 if (word[char_i]!=' ') { // if not space 00169 morse_i = _morse_find(word[char_i]); 00170 if (morse_i==-1) continue; // if not found 00171 00172 size += _morse_values_size[morse_i]; 00173 size += 3; // plus the 3 blanks between chars 00174 } 00175 else { // else space 00176 if (char_i==0 || word[char_i-1]==' ') size+=7; 00177 else size+=4; // if not first letter, already 3 from the last char 00178 } 00179 } 00180 if (word[char_i-1]!=' ') size -= 3; // remove last space between elements (but not if space) 00181 00182 return size; 00183 } 00184 00185 void morse_encode(char* word, Morse_data* data) { 00186 unsigned int char_i; 00187 int morse_i; 00188 unsigned int res_counter; 00189 00190 res_counter = 0; 00191 for (char_i=0; word[char_i]!='\0'; char_i++) { 00192 if (word[char_i]!=' ') { 00193 morse_i = _morse_find(word[char_i]); 00194 if (morse_i==-1) continue; // if not found 00195 00196 res_counter += _morse_addChar(morse_i, data->data, res_counter); // if not space 00197 res_counter += 3; // add space between elements 00198 } 00199 else { // else space 00200 if (char_i==0 || word[char_i-1]==' ') res_counter+=7; // if not char last time 00201 else res_counter += 4; 00202 } 00203 } 00204 if (word[char_i-1]!=' ') res_counter -= 3; // remove last space between elements (but not if space) 00205 data->length = res_counter; 00206 } 00207 00208 Morse_data* morse_create(unsigned int length) { 00209 Morse_data* data = new Morse_data; 00210 data->length = length; 00211 data->data = new bool[length](); 00212 return data; 00213 } 00214 void morse_destroy(Morse_data* data) { 00215 delete[] data->data; 00216 delete data; 00217 }
Generated on Sun Jul 31 2022 00:23:01 by
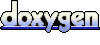