Morse Encoder/Decoder Library. Transforms char array to binary array and vice-versa.
Embed:
(wiki syntax)
Show/hide line numbers
MorseDecoder.cpp
00001 /* 00002 Copyright (c) 2014 Romain Berrada 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 furnished to do so, subject to the following conditions: 00009 00010 The above copyright notice and this permission notice shall be included in all copies or 00011 substantial portions of the Software. 00012 00013 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #include "Morse.h" 00021 #include "mbed.h" 00022 00023 struct _morse_tree { 00024 char value; 00025 const struct _morse_tree* dot; 00026 const struct _morse_tree* dash; 00027 }; 00028 00029 // Depth 5 of the tree 00030 static const struct _morse_tree _morse_tree_1 = {'1', 0, 0}; 00031 static const struct _morse_tree _morse_tree_2 = {'2', 0, 0}; 00032 static const struct _morse_tree _morse_tree_3 = {'3', 0, 0}; 00033 static const struct _morse_tree _morse_tree_4 = {'4', 0, 0}; 00034 static const struct _morse_tree _morse_tree_5 = {'5', 0, 0}; 00035 static const struct _morse_tree _morse_tree_6 = {'6', 0, 0}; 00036 static const struct _morse_tree _morse_tree_7 = {'7', 0, 0}; 00037 static const struct _morse_tree _morse_tree_8 = {'8', 0, 0}; 00038 static const struct _morse_tree _morse_tree_9 = {'9', 0, 0}; 00039 static const struct _morse_tree _morse_tree_0 = {'0', 0, 0}; 00040 static const struct _morse_tree _morse_tree_AR= {'@', 0, 0}; // @ added as DOT,DOT,DOT,DASH,DOT 00041 static const struct _morse_tree _morse_tree_IN= {'?', 0, 0}; // ? added as DOT,DOT,DASH,DOT,DOT 00042 00043 // Depth 4 of the tree 00044 static const struct _morse_tree _morse_tree_H = {'H', &_morse_tree_5, &_morse_tree_4}; 00045 static const struct _morse_tree _morse_tree_V = {'V',&_morse_tree_AR ,&_morse_tree_3}; 00046 static const struct _morse_tree _morse_tree_F = {'F',&_morse_tree_IN, 0}; 00047 static const struct _morse_tree _morse_tree_MN= {'-', 0, &_morse_tree_2}; // - added as DOT,DOT,DASH,DASH 00048 static const struct _morse_tree _morse_tree_L = {'L', 0, 0}; 00049 static const struct _morse_tree _morse_tree_SL= {'/', 0, 0}; // / added as DOT,DASH,DOT,DASH 00050 static const struct _morse_tree _morse_tree_P = {'P', 0, 0}; 00051 static const struct _morse_tree _morse_tree_J = {'J', 0, &_morse_tree_1}; 00052 static const struct _morse_tree _morse_tree_B = {'B', &_morse_tree_6, 0}; 00053 static const struct _morse_tree _morse_tree_X = {'X', 0, 0}; 00054 static const struct _morse_tree _morse_tree_C = {'C', 0, 0}; 00055 static const struct _morse_tree _morse_tree_Y = {'Y', 0, 0}; 00056 static const struct _morse_tree _morse_tree_Z = {'Z', &_morse_tree_7, 0}; 00057 static const struct _morse_tree _morse_tree_Q = {'Q', 0, 0}; 00058 static const struct _morse_tree _morse_tree_UN= {'_', &_morse_tree_8, 0}; // _ added as DASH,DASH,DASH,DOT 00059 static const struct _morse_tree _morse_tree_DO= {'.', &_morse_tree_9, &_morse_tree_0}; // . added as DASH,DASH,DASH,DASH 00060 00061 // Depth 3 of the tree 00062 static const struct _morse_tree _morse_tree_S = {'S', &_morse_tree_H, &_morse_tree_V}; 00063 static const struct _morse_tree _morse_tree_U = {'U', &_morse_tree_F, &_morse_tree_MN}; 00064 static const struct _morse_tree _morse_tree_R = {'R', &_morse_tree_L, &_morse_tree_SL}; 00065 static const struct _morse_tree _morse_tree_W = {'W', &_morse_tree_P, &_morse_tree_J}; 00066 static const struct _morse_tree _morse_tree_D = {'D', &_morse_tree_B, &_morse_tree_X}; 00067 static const struct _morse_tree _morse_tree_K = {'K', &_morse_tree_C, &_morse_tree_Y}; 00068 static const struct _morse_tree _morse_tree_G = {'G', &_morse_tree_Z, &_morse_tree_Q}; 00069 static const struct _morse_tree _morse_tree_O = {'O', &_morse_tree_UN,&_morse_tree_DO}; 00070 00071 // Depth 2 of the tree 00072 static const struct _morse_tree _morse_tree_I = {'I', &_morse_tree_S, &_morse_tree_U}; 00073 static const struct _morse_tree _morse_tree_A = {'A', &_morse_tree_R, &_morse_tree_W}; 00074 static const struct _morse_tree _morse_tree_N = {'N', &_morse_tree_D, &_morse_tree_K}; 00075 static const struct _morse_tree _morse_tree_M = {'M', &_morse_tree_G, &_morse_tree_O}; 00076 00077 // Depth 1 of the tree 00078 static const struct _morse_tree _morse_tree_E = {'E', &_morse_tree_I, &_morse_tree_A}; 00079 static const struct _morse_tree _morse_tree_T = {'T', &_morse_tree_N, &_morse_tree_M}; 00080 00081 static const struct _morse_tree _morse_tree_root = {'#', &_morse_tree_E, &_morse_tree_T}; 00082 00083 unsigned int morse_getWordSize(Morse_data* data) { 00084 unsigned int i; 00085 unsigned int blank_counter=0; 00086 unsigned int size=0; 00087 00088 for (i=0; i<data->length; i++) { 00089 if (data->data[i]==false) blank_counter++; 00090 else { // else true 00091 // checks all the blanks so far, and then reset the counter 00092 if (blank_counter==3) size++; // if blanks to separate two chars 00093 else if (blank_counter==7) size+=2; // if blanks for a space : acknowledge the space and the char before the space 00094 blank_counter=0; 00095 } 00096 } 00097 size++; // acknowledge the last char 00098 if (blank_counter>=7) size++; 00099 return size; 00100 } 00101 00102 void morse_decode(Morse_data* data, char* word) { 00103 unsigned int i; 00104 unsigned int char_i=0; 00105 unsigned int false_counter=0; 00106 unsigned int true_counter=0; 00107 const struct _morse_tree* tree = &_morse_tree_root; // CHANGE TO THE BINRARY TREE IMPLEMENTATION AND PUT THE ROOT INSTEAD 00108 00109 for (i=0; i<data->length; i++) { 00110 if (data->data[i]==false) { 00111 // if false, increase the false_counter, manage the number of true and false before, and reset the true_counter 00112 false_counter++; 00113 00114 if (true_counter==1) tree = tree->dot; 00115 else if (true_counter==3) tree = tree->dash; 00116 00117 if (false_counter==3) { 00118 word[char_i++] = tree->value; 00119 tree = &_morse_tree_root; 00120 } 00121 else if (false_counter==7) word[char_i++] = ' '; 00122 00123 true_counter=0; 00124 } 00125 else { // else true 00126 true_counter++; 00127 false_counter=0; 00128 } 00129 } 00130 00131 if (true_counter==1) word[char_i++] = tree->dot->value; 00132 else if (true_counter==3) word[char_i++] = tree->dash->value; 00133 00134 word[char_i] = '\0'; // finally, end the char array 00135 }
Generated on Sun Jul 31 2022 00:23:01 by
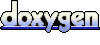