
A simple example how to receive ethernet packages and display them over stdout with an hexviewer
Embed:
(wiki syntax)
Show/hide line numbers
hexview.h
00001 /* hexview functions 00002 * Copyright (c) 2009 rmeyer 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 /* Function: hexview 00007 * Prints an array of char to stdout in hex. 00008 * The data is grouped in two 8 byte groups per line. 00009 * Each byte is displayed as 2 hex digits and every 00010 * line starts with the address of the first byte. 00011 * 00012 * There is no text view of a line. 00013 * 00014 * Variables: 00015 * buffer - The array to display. 00016 * size - The length of buffer. 00017 */ 00018 inline void hexview(const char *buffer, unsigned int size) { 00019 for(int i = 0; i < size; ++i) { 00020 if((i%16)!=0) { 00021 printf(" "); 00022 } else { 00023 printf("%04X: ", (i)); 00024 } 00025 printf("%02hhx", buffer[i]); 00026 if((i%16) == 7) { 00027 printf(" "); 00028 } 00029 if((i%16) == 15) { 00030 printf("\n"); 00031 } 00032 } 00033 printf("\n\n\n"); 00034 } 00035 00036 /* Function: hexview 00037 * Prints an array of char to stdout in hex. 00038 * The data is grouped in two 8 byte groups per line. 00039 * Each byte is displayed as 2 hex digits and every 00040 * line starts with the address of the first byte. 00041 * Each line ends ub with an ASCII representation 00042 * of the bytes. 00043 * 00044 * This implementation takes more stack space than the other. 00045 * It will allocate two char arrays with a agregated size of 70 bytes. 00046 * Therefore its faster than the fierst implementation. 00047 * It operates directly on the char arrays and make no use of 00048 * string manipulation functions. printf is called one time a line. 00049 * 00050 * Variables: 00051 * buffer - The array to display. 00052 * size - The length of buffer. 00053 */ 00054 inline void hexview2(const char *buffer, unsigned int size) { 00055 char byte[50]; 00056 char text[20]; 00057 bool big = false; 00058 int i; 00059 for(i = 0; i < size; ++i) { 00060 if((i&0xF) == 0x0) { 00061 if(big) 00062 printf("%04X: %-49s: %-20s\n", (i&~0xF), byte, text); 00063 big = false; 00064 byte[0] = '\0'; 00065 text[0] = '\0'; 00066 } else if((i&0xF) == 0x8) { 00067 big = true; 00068 byte[(i&0xF) * 3] = ' '; 00069 text[(i&0xF)] = ' '; 00070 } 00071 unsigned char value = buffer[i]; 00072 text[(i&0xF) + 0 + big] = (value < 0x20 || value > 0x7F)? '.': value; 00073 text[(i&0xF) + 1 + big] = '\0'; 00074 value = (buffer[i] &0xF0) >> 4; 00075 byte[(i&0xF) * 3 + 0 + big] = (value < 0xA)? (value + 0x30): (value + 0x37); 00076 value = (buffer[i] &0x0F); 00077 byte[(i&0xF) * 3 + 1 + big] = (value < 0xA)? (value + 0x30): (value + 0x37); 00078 byte[(i&0xF) * 3 + 2 + big] = ' '; 00079 byte[(i&0xF) * 3 + 3 + big] = '\0'; 00080 } 00081 if(byte[0]) { 00082 printf("%04X: %-49s: %-20s\n", (i&~0xF), byte, text); 00083 } 00084 printf("\n"); 00085 }
Generated on Fri Jul 15 2022 03:13:59 by
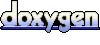