
by Rob Toulson and Tim Wilmshurst from textbook "Fast and Effective Embedded Systems Design: Applying the ARM mbed"
main.cpp
00001 /*Program Example 11.2 Low pass filter function 00002 */ 00003 float LPF(float LPF_in){ 00004 00005 float a[4]={1,2.6235518066,-2.3146825811,0.6855359773}; 00006 float b[4]={0.0006993496,0.0020980489,0.0020980489,0.0006993496}; 00007 static float LPF_out; 00008 static float x[4], y[4]; 00009 00010 x[3] = x[2]; x[2] = x[1]; x[1] = x[0]; //move x values by one sample 00011 y[3] = y[2]; y[2] = y[1]; y[1] = y[0]; //move y values by one sample 00012 00013 x[0] = LPF_in; // new value for x[0] 00014 y[0] = (b[0]*x[0]) + (b[1]*x[1]) + (b[2]*x[2]) + (b[3]*x[3]) 00015 + (a[1]*y[1]) + (a[2]*y[2]) + (a[3]*y[3]); 00016 00017 LPF_out = y[0]; 00018 return LPF_out; // output filtered value 00019 } 00020
Generated on Tue Aug 2 2022 01:27:52 by
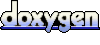