Aded CMSIS5 DSP and NN folder. Needs some work
Complex FFT Tables
[Complex FFT Functions]
Variables | |
const uint16_t | armBitRevTable [1024] |
const float32_t | twiddleCoef_16 [32] |
const float32_t | twiddleCoef_32 [64] |
const float32_t | twiddleCoef_64 [128] |
const float32_t | twiddleCoef_128 [256] |
const float32_t | twiddleCoef_256 [512] |
const float32_t | twiddleCoef_512 [1024] |
const float32_t | twiddleCoef_1024 [2048] |
const float32_t | twiddleCoef_2048 [4096] |
const float32_t | twiddleCoef_4096 [8192] |
const q31_t | twiddleCoef_16_q31 [24] |
const q31_t | twiddleCoef_32_q31 [48] |
const q31_t | twiddleCoef_64_q31 [96] |
const q31_t | twiddleCoef_128_q31 [192] |
const q31_t | twiddleCoef_256_q31 [384] |
const q31_t | twiddleCoef_512_q31 [768] |
const q31_t | twiddleCoef_1024_q31 [1536] |
const q31_t | twiddleCoef_2048_q31 [3072] |
const q31_t | twiddleCoef_4096_q31 [6144] |
const q15_t | twiddleCoef_16_q15 [24] |
const q15_t | twiddleCoef_32_q15 [48] |
const q15_t | twiddleCoef_64_q15 [96] |
const q15_t | twiddleCoef_128_q15 [192] |
const q15_t | twiddleCoef_256_q15 [384] |
const q15_t | twiddleCoef_512_q15 [768] |
const q15_t | twiddleCoef_1024_q15 [1536] |
const q15_t | twiddleCoef_2048_q15 [3072] |
const q15_t | twiddleCoef_4096_q15 [6144] |
Variable Documentation
const uint16_t armBitRevTable[1024] |
- Pseudo code for Generation of Bit reversal Table is
for(l=1;l <= N/4;l++) { for(i=0;i<logN2;i++) { a[i]=l&(1<<i); } for(j=0; j<logN2; j++) { if (a[j]!=0) y[l]+=(1<<((logN2-1)-j)); } y[l] = y[l] >> 1; }
- where N = 4096 logN2 = 12
- N is the maximum FFT Size supported
Definition at line 67 of file arm_common_tables.c.
const float32_t twiddleCoef_1024[2048] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 1024 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 1309 of file arm_common_tables.c.
const q15_t twiddleCoef_1024_q15[1536] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 1024 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 13358 of file arm_common_tables.c.
const q31_t twiddleCoef_1024_q31[1536] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 1024 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 9181 of file arm_common_tables.c.
const float32_t twiddleCoef_128[256] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 128 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 360 of file arm_common_tables.c.
const q15_t twiddleCoef_128_q15[192] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 128 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 12959 of file arm_common_tables.c.
const q31_t twiddleCoef_128_q31[192] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 128 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8670 of file arm_common_tables.c.
const float32_t twiddleCoef_16[32] |
{ 1.000000000f, 0.000000000f, 0.923879533f, 0.382683432f, 0.707106781f, 0.707106781f, 0.382683432f, 0.923879533f, 0.000000000f, 1.000000000f, -0.382683432f, 0.923879533f, -0.707106781f, 0.707106781f, -0.923879533f, 0.382683432f, -1.000000000f, 0.000000000f, -0.923879533f, -0.382683432f, -0.707106781f, -0.707106781f, -0.382683432f, -0.923879533f, -0.000000000f, -1.000000000f, 0.382683432f, -0.923879533f, 0.707106781f, -0.707106781f, 0.923879533f, -0.382683432f }
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 16 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 193 of file arm_common_tables.c.
const q15_t twiddleCoef_16_q15[24] |
{ (q15_t)0x7FFF, (q15_t)0x0000, (q15_t)0x7641, (q15_t)0x30FB, (q15_t)0x5A82, (q15_t)0x5A82, (q15_t)0x30FB, (q15_t)0x7641, (q15_t)0x0000, (q15_t)0x7FFF, (q15_t)0xCF04, (q15_t)0x7641, (q15_t)0xA57D, (q15_t)0x5A82, (q15_t)0x89BE, (q15_t)0x30FB, (q15_t)0x8000, (q15_t)0x0000, (q15_t)0x89BE, (q15_t)0xCF04, (q15_t)0xA57D, (q15_t)0xA57D, (q15_t)0xCF04, (q15_t)0x89BE }
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 16 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 12836 of file arm_common_tables.c.
const q31_t twiddleCoef_16_q31[24] |
{ (q31_t)0x7FFFFFFF, (q31_t)0x00000000, (q31_t)0x7641AF3C, (q31_t)0x30FBC54D, (q31_t)0x5A82799A, (q31_t)0x5A82799A, (q31_t)0x30FBC54D, (q31_t)0x7641AF3C, (q31_t)0x00000000, (q31_t)0x7FFFFFFF, (q31_t)0xCF043AB2, (q31_t)0x7641AF3C, (q31_t)0xA57D8666, (q31_t)0x5A82799A, (q31_t)0x89BE50C3, (q31_t)0x30FBC54D, (q31_t)0x80000000, (q31_t)0x00000000, (q31_t)0x89BE50C3, (q31_t)0xCF043AB2, (q31_t)0xA57D8666, (q31_t)0xA57D8666, (q31_t)0xCF043AB2, (q31_t)0x89BE50C3 }
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 16 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8539 of file arm_common_tables.c.
const float32_t twiddleCoef_2048[4096] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 2048 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 2351 of file arm_common_tables.c.
const q15_t twiddleCoef_2048_q15[3072] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 2048 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 13763 of file arm_common_tables.c.
const q31_t twiddleCoef_2048_q31[3072] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 2048 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 9714 of file arm_common_tables.c.
const float32_t twiddleCoef_256[512] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 256 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 506 of file arm_common_tables.c.
const q15_t twiddleCoef_256_q15[384] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 256 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 13028 of file arm_common_tables.c.
const q31_t twiddleCoef_256_q31[384] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 256 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8755 of file arm_common_tables.c.
const float32_t twiddleCoef_32[64] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 32 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 227 of file arm_common_tables.c.
const q15_t twiddleCoef_32_q15[48] |
{ (q15_t)0x7FFF, (q15_t)0x0000, (q15_t)0x7D8A, (q15_t)0x18F8, (q15_t)0x7641, (q15_t)0x30FB, (q15_t)0x6A6D, (q15_t)0x471C, (q15_t)0x5A82, (q15_t)0x5A82, (q15_t)0x471C, (q15_t)0x6A6D, (q15_t)0x30FB, (q15_t)0x7641, (q15_t)0x18F8, (q15_t)0x7D8A, (q15_t)0x0000, (q15_t)0x7FFF, (q15_t)0xE707, (q15_t)0x7D8A, (q15_t)0xCF04, (q15_t)0x7641, (q15_t)0xB8E3, (q15_t)0x6A6D, (q15_t)0xA57D, (q15_t)0x5A82, (q15_t)0x9592, (q15_t)0x471C, (q15_t)0x89BE, (q15_t)0x30FB, (q15_t)0x8275, (q15_t)0x18F8, (q15_t)0x8000, (q15_t)0x0000, (q15_t)0x8275, (q15_t)0xE707, (q15_t)0x89BE, (q15_t)0xCF04, (q15_t)0x9592, (q15_t)0xB8E3, (q15_t)0xA57D, (q15_t)0xA57D, (q15_t)0xB8E3, (q15_t)0x9592, (q15_t)0xCF04, (q15_t)0x89BE, (q15_t)0xE707, (q15_t)0x8275 }
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 32 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 12869 of file arm_common_tables.c.
const q31_t twiddleCoef_32_q31[48] |
{ (q31_t)0x7FFFFFFF, (q31_t)0x00000000, (q31_t)0x7D8A5F3F, (q31_t)0x18F8B83C, (q31_t)0x7641AF3C, (q31_t)0x30FBC54D, (q31_t)0x6A6D98A4, (q31_t)0x471CECE6, (q31_t)0x5A82799A, (q31_t)0x5A82799A, (q31_t)0x471CECE6, (q31_t)0x6A6D98A4, (q31_t)0x30FBC54D, (q31_t)0x7641AF3C, (q31_t)0x18F8B83C, (q31_t)0x7D8A5F3F, (q31_t)0x00000000, (q31_t)0x7FFFFFFF, (q31_t)0xE70747C3, (q31_t)0x7D8A5F3F, (q31_t)0xCF043AB2, (q31_t)0x7641AF3C, (q31_t)0xB8E31319, (q31_t)0x6A6D98A4, (q31_t)0xA57D8666, (q31_t)0x5A82799A, (q31_t)0x9592675B, (q31_t)0x471CECE6, (q31_t)0x89BE50C3, (q31_t)0x30FBC54D, (q31_t)0x8275A0C0, (q31_t)0x18F8B83C, (q31_t)0x80000000, (q31_t)0x00000000, (q31_t)0x8275A0C0, (q31_t)0xE70747C3, (q31_t)0x89BE50C3, (q31_t)0xCF043AB2, (q31_t)0x9592675B, (q31_t)0xB8E31319, (q31_t)0xA57D8666, (q31_t)0xA57D8666, (q31_t)0xB8E31319, (q31_t)0x9592675B, (q31_t)0xCF043AB2, (q31_t)0x89BE50C3, (q31_t)0xE70747C3, (q31_t)0x8275A0C0 }
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 32 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8572 of file arm_common_tables.c.
const float32_t twiddleCoef_4096[8192] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 4096 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 4417 of file arm_common_tables.c.
const q15_t twiddleCoef_4096_q15[6144] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 4096 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 14552 of file arm_common_tables.c.
const q31_t twiddleCoef_4096_q31[6144] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 4096 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 10759 of file arm_common_tables.c.
const float32_t twiddleCoef_512[1024] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 512 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 780 of file arm_common_tables.c.
const q15_t twiddleCoef_512_q15[768] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 512 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 13145 of file arm_common_tables.c.
const q31_t twiddleCoef_512_q31[768] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 512 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8904 of file arm_common_tables.c.
const float32_t twiddleCoef_64[128] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 64 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 277 of file arm_common_tables.c.
const q15_t twiddleCoef_64_q15[96] |
{ (q15_t)0x7FFF, (q15_t)0x0000, (q15_t)0x7F62, (q15_t)0x0C8B, (q15_t)0x7D8A, (q15_t)0x18F8, (q15_t)0x7A7D, (q15_t)0x2528, (q15_t)0x7641, (q15_t)0x30FB, (q15_t)0x70E2, (q15_t)0x3C56, (q15_t)0x6A6D, (q15_t)0x471C, (q15_t)0x62F2, (q15_t)0x5133, (q15_t)0x5A82, (q15_t)0x5A82, (q15_t)0x5133, (q15_t)0x62F2, (q15_t)0x471C, (q15_t)0x6A6D, (q15_t)0x3C56, (q15_t)0x70E2, (q15_t)0x30FB, (q15_t)0x7641, (q15_t)0x2528, (q15_t)0x7A7D, (q15_t)0x18F8, (q15_t)0x7D8A, (q15_t)0x0C8B, (q15_t)0x7F62, (q15_t)0x0000, (q15_t)0x7FFF, (q15_t)0xF374, (q15_t)0x7F62, (q15_t)0xE707, (q15_t)0x7D8A, (q15_t)0xDAD7, (q15_t)0x7A7D, (q15_t)0xCF04, (q15_t)0x7641, (q15_t)0xC3A9, (q15_t)0x70E2, (q15_t)0xB8E3, (q15_t)0x6A6D, (q15_t)0xAECC, (q15_t)0x62F2, (q15_t)0xA57D, (q15_t)0x5A82, (q15_t)0x9D0D, (q15_t)0x5133, (q15_t)0x9592, (q15_t)0x471C, (q15_t)0x8F1D, (q15_t)0x3C56, (q15_t)0x89BE, (q15_t)0x30FB, (q15_t)0x8582, (q15_t)0x2528, (q15_t)0x8275, (q15_t)0x18F8, (q15_t)0x809D, (q15_t)0x0C8B, (q15_t)0x8000, (q15_t)0x0000, (q15_t)0x809D, (q15_t)0xF374, (q15_t)0x8275, (q15_t)0xE707, (q15_t)0x8582, (q15_t)0xDAD7, (q15_t)0x89BE, (q15_t)0xCF04, (q15_t)0x8F1D, (q15_t)0xC3A9, (q15_t)0x9592, (q15_t)0xB8E3, (q15_t)0x9D0D, (q15_t)0xAECC, (q15_t)0xA57D, (q15_t)0xA57D, (q15_t)0xAECC, (q15_t)0x9D0D, (q15_t)0xB8E3, (q15_t)0x9592, (q15_t)0xC3A9, (q15_t)0x8F1D, (q15_t)0xCF04, (q15_t)0x89BE, (q15_t)0xDAD7, (q15_t)0x8582, (q15_t)0xE707, (q15_t)0x8275, (q15_t)0xF374, (q15_t)0x809D }
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 64 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 12914 of file arm_common_tables.c.
const q31_t twiddleCoef_64_q31[96] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 64 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8617 of file arm_common_tables.c.
Generated on Tue Jul 12 2022 16:47:30 by
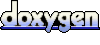