Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_cfft_radix4_init_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_cfft_radix4_init_q15.c 00004 * Description: Radix-4 Decimation in Frequency Q15 FFT & IFFT initialization function 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 #include "arm_common_tables.h" 00031 00032 /** 00033 * @ingroup groupTransforms 00034 */ 00035 00036 00037 /** 00038 * @addtogroup ComplexFFT 00039 * @{ 00040 */ 00041 00042 00043 /** 00044 * @brief Initialization function for the Q15 CFFT/CIFFT. 00045 * @deprecated Do not use this function. It has been superseded by \ref arm_cfft_q15 and will be removed 00046 * @param[in,out] *S points to an instance of the Q15 CFFT/CIFFT structure. 00047 * @param[in] fftLen length of the FFT. 00048 * @param[in] ifftFlag flag that selects forward (ifftFlag=0) or inverse (ifftFlag=1) transform. 00049 * @param[in] bitReverseFlag flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output. 00050 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if <code>fftLen</code> is not a supported value. 00051 * 00052 * \par Description: 00053 * \par 00054 * The parameter <code>ifftFlag</code> controls whether a forward or inverse transform is computed. 00055 * Set(=1) ifftFlag for calculation of CIFFT otherwise CFFT is calculated 00056 * \par 00057 * The parameter <code>bitReverseFlag</code> controls whether output is in normal order or bit reversed order. 00058 * Set(=1) bitReverseFlag for output to be in normal order otherwise output is in bit reversed order. 00059 * \par 00060 * The parameter <code>fftLen</code> Specifies length of CFFT/CIFFT process. Supported FFT Lengths are 16, 64, 256, 1024. 00061 * \par 00062 * This Function also initializes Twiddle factor table pointer and Bit reversal table pointer. 00063 */ 00064 00065 arm_status arm_cfft_radix4_init_q15( 00066 arm_cfft_radix4_instance_q15 * S, 00067 uint16_t fftLen, 00068 uint8_t ifftFlag, 00069 uint8_t bitReverseFlag) 00070 { 00071 /* Initialise the default arm status */ 00072 arm_status status = ARM_MATH_SUCCESS; 00073 /* Initialise the FFT length */ 00074 S->fftLen = fftLen; 00075 /* Initialise the Twiddle coefficient pointer */ 00076 S->pTwiddle = (q15_t *) twiddleCoef_4096_q15 ; 00077 /* Initialise the Flag for selection of CFFT or CIFFT */ 00078 S->ifftFlag = ifftFlag; 00079 /* Initialise the Flag for calculation Bit reversal or not */ 00080 S->bitReverseFlag = bitReverseFlag; 00081 00082 /* Initializations of structure parameters depending on the FFT length */ 00083 switch (S->fftLen) 00084 { 00085 case 4096U: 00086 /* Initializations of structure parameters for 4096 point FFT */ 00087 00088 /* Initialise the twiddle coef modifier value */ 00089 S->twidCoefModifier = 1U; 00090 /* Initialise the bit reversal table modifier */ 00091 S->bitRevFactor = 1U; 00092 /* Initialise the bit reversal table pointer */ 00093 S->pBitRevTable = (uint16_t *) armBitRevTable ; 00094 00095 break; 00096 00097 case 1024U: 00098 /* Initializations of structure parameters for 1024 point FFT */ 00099 S->twidCoefModifier = 4U; 00100 S->bitRevFactor = 4U; 00101 S->pBitRevTable = (uint16_t *) & armBitRevTable [3]; 00102 00103 break; 00104 00105 case 256U: 00106 /* Initializations of structure parameters for 256 point FFT */ 00107 S->twidCoefModifier = 16U; 00108 S->bitRevFactor = 16U; 00109 S->pBitRevTable = (uint16_t *) & armBitRevTable [15]; 00110 00111 break; 00112 00113 case 64U: 00114 /* Initializations of structure parameters for 64 point FFT */ 00115 S->twidCoefModifier = 64U; 00116 S->bitRevFactor = 64U; 00117 S->pBitRevTable = (uint16_t *) & armBitRevTable [63]; 00118 00119 break; 00120 00121 case 16U: 00122 /* Initializations of structure parameters for 16 point FFT */ 00123 S->twidCoefModifier = 256U; 00124 S->bitRevFactor = 256U; 00125 S->pBitRevTable = (uint16_t *) & armBitRevTable [255]; 00126 00127 break; 00128 00129 default: 00130 /* Reporting argument error if fftSize is not valid value */ 00131 status = ARM_MATH_ARGUMENT_ERROR; 00132 break; 00133 } 00134 00135 return (status); 00136 } 00137 00138 /** 00139 * @} end of ComplexFFT group 00140 */ 00141
Generated on Tue Jul 12 2022 16:46:23 by
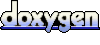