CppUTest is a C /C++ based unit xUnit test framework for unit testing and for test-driving your code.
Embed:
(wiki syntax)
Show/hide line numbers
TestResult.h
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 /////////////////////////////////////////////////////////////////////////////// 00029 // 00030 // A TestResult is a collection of the history of some test runs. Right now 00031 // it just collects failures. Really it just prints the failures. 00032 // 00033 00034 #ifndef D_TestResult_h 00035 #define D_TestResult_h 00036 00037 class TestFailure; 00038 class TestOutput; 00039 class UtestShell; 00040 00041 class TestResult 00042 { 00043 public: 00044 TestResult(TestOutput&); 00045 DEFAULT_COPY_CONSTRUCTOR(TestResult) 00046 virtual ~TestResult(); 00047 00048 virtual void testsStarted(); 00049 virtual void testsEnded(); 00050 virtual void currentGroupStarted(UtestShell* test); 00051 virtual void currentGroupEnded(UtestShell* test); 00052 virtual void currentTestStarted(UtestShell* test); 00053 virtual void currentTestEnded(UtestShell* test); 00054 00055 virtual void countTest(); 00056 virtual void countRun(); 00057 virtual void countCheck(); 00058 virtual void countFilteredOut(); 00059 virtual void countIgnored(); 00060 virtual void addFailure(const TestFailure& failure); 00061 virtual void print(const char* text); 00062 virtual void setProgressIndicator(const char*); 00063 00064 int getTestCount() const 00065 { 00066 return testCount_; 00067 } 00068 int getRunCount() const 00069 { 00070 return runCount_; 00071 } 00072 int getCheckCount() const 00073 { 00074 return checkCount_; 00075 } 00076 int getFilteredOutCount() const 00077 { 00078 return filteredOutCount_; 00079 } 00080 int getIgnoredCount() const 00081 { 00082 return ignoredCount_; 00083 } 00084 int getFailureCount() const 00085 { 00086 return failureCount_; 00087 } 00088 00089 long getTotalExecutionTime() const; 00090 void setTotalExecutionTime(long exTime); 00091 00092 long getCurrentTestTotalExecutionTime() const; 00093 long getCurrentGroupTotalExecutionTime() const; 00094 private: 00095 00096 TestOutput& output_; 00097 int testCount_; 00098 int runCount_; 00099 int checkCount_; 00100 int failureCount_; 00101 int filteredOutCount_; 00102 int ignoredCount_; 00103 long totalExecutionTime_; 00104 long timeStarted_; 00105 long currentTestTimeStarted_; 00106 long currentTestTotalExecutionTime_; 00107 long currentGroupTimeStarted_; 00108 long currentGroupTotalExecutionTime_; 00109 }; 00110 00111 #endif
Generated on Tue Jul 12 2022 18:58:09 by
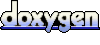