CppUTest is a C /C++ based unit xUnit test framework for unit testing and for test-driving your code.
Embed:
(wiki syntax)
Show/hide line numbers
TestHarness_c.cpp
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #include "CppUTest/TestHarness.h" 00029 #include "CppUTest/MemoryLeakDetector.h" 00030 #include "CppUTest/TestMemoryAllocator.h" 00031 #include "CppUTest/PlatformSpecificFunctions.h" 00032 #include "CppUTest/TestHarness_c.h" 00033 00034 extern "C" 00035 { 00036 00037 00038 void CHECK_EQUAL_C_INT_LOCATION(int expected, int actual, const char* fileName, int lineNumber) 00039 { 00040 UtestShell::getCurrent()->assertLongsEqual((long)expected, (long)actual, fileName, lineNumber, TestTerminatorWithoutExceptions()); 00041 } 00042 00043 void CHECK_EQUAL_C_REAL_LOCATION(double expected, double actual, double threshold, const char* fileName, int lineNumber) 00044 { 00045 UtestShell::getCurrent()->assertDoublesEqual(expected, actual, threshold, fileName, lineNumber, TestTerminatorWithoutExceptions()); 00046 } 00047 00048 void CHECK_EQUAL_C_CHAR_LOCATION(char expected, char actual, const char* fileName, int lineNumber) 00049 { 00050 UtestShell::getCurrent()->assertEquals(((expected) != (actual)), StringFrom(expected).asCharString(), StringFrom(actual).asCharString(), fileName, lineNumber, TestTerminatorWithoutExceptions()); 00051 } 00052 00053 void CHECK_EQUAL_C_STRING_LOCATION(const char* expected, const char* actual, const char* fileName, int lineNumber) 00054 { 00055 UtestShell::getCurrent()->assertCstrEqual(expected, actual, fileName, lineNumber, TestTerminatorWithoutExceptions()); 00056 } 00057 00058 void FAIL_TEXT_C_LOCATION(const char* text, const char* fileName, int lineNumber) 00059 { 00060 UtestShell::getCurrent()->fail(text, fileName, lineNumber, TestTerminatorWithoutExceptions()); 00061 } 00062 00063 void FAIL_C_LOCATION(const char* fileName, int lineNumber) 00064 { 00065 UtestShell::getCurrent()->fail("", fileName, lineNumber, TestTerminatorWithoutExceptions()); 00066 } 00067 00068 void CHECK_C_LOCATION(int condition, const char* conditionString, const char* fileName, int lineNumber) 00069 { 00070 UtestShell::getCurrent()->assertTrue(condition != 0, "CHECK_C", conditionString, fileName, lineNumber, TestTerminatorWithoutExceptions()); 00071 } 00072 00073 enum { NO_COUNTDOWN = -1, OUT_OF_MEMORRY = 0 }; 00074 static int malloc_out_of_memory_counter = NO_COUNTDOWN; 00075 static int malloc_count = 0; 00076 00077 void cpputest_malloc_count_reset(void) 00078 { 00079 malloc_count = 0; 00080 } 00081 00082 int cpputest_malloc_get_count() 00083 { 00084 return malloc_count; 00085 } 00086 00087 void cpputest_malloc_set_out_of_memory() 00088 { 00089 setCurrentMallocAllocator(NullUnknownAllocator::defaultAllocator()); 00090 } 00091 00092 void cpputest_malloc_set_not_out_of_memory() 00093 { 00094 malloc_out_of_memory_counter = NO_COUNTDOWN; 00095 setCurrentMallocAllocatorToDefault(); 00096 } 00097 00098 void cpputest_malloc_set_out_of_memory_countdown(int count) 00099 { 00100 malloc_out_of_memory_counter = count; 00101 if (malloc_out_of_memory_counter == OUT_OF_MEMORRY) 00102 cpputest_malloc_set_out_of_memory(); 00103 } 00104 00105 void* cpputest_malloc(size_t size) 00106 { 00107 return cpputest_malloc_location(size, "<unknown>", 0); 00108 } 00109 00110 void* cpputest_calloc(size_t num, size_t size) 00111 { 00112 return cpputest_calloc_location(num, size, "<unknown>", 0); 00113 } 00114 00115 void* cpputest_realloc(void* ptr, size_t size) 00116 { 00117 return cpputest_realloc_location(ptr, size, "<unknown>", 0); 00118 } 00119 00120 void cpputest_free(void* buffer) 00121 { 00122 cpputest_free_location(buffer, "<unknown>", 0); 00123 } 00124 00125 static void countdown() 00126 { 00127 if (malloc_out_of_memory_counter <= NO_COUNTDOWN) 00128 return; 00129 00130 if (malloc_out_of_memory_counter == OUT_OF_MEMORRY) 00131 return; 00132 00133 malloc_out_of_memory_counter--; 00134 00135 if (malloc_out_of_memory_counter == OUT_OF_MEMORRY) 00136 cpputest_malloc_set_out_of_memory(); 00137 } 00138 00139 void* cpputest_malloc_location(size_t size, const char* file, int line) 00140 { 00141 countdown(); 00142 malloc_count++; 00143 return cpputest_malloc_location_with_leak_detection(size, file, line); 00144 } 00145 00146 void* cpputest_calloc_location(size_t num, size_t size, const char* file, int line) 00147 { 00148 void* mem = cpputest_malloc_location(num * size, file, line); 00149 if (mem) 00150 PlatformSpecificMemset(mem, 0, num*size); 00151 return mem; 00152 } 00153 00154 void* cpputest_realloc_location(void* memory, size_t size, const char* file, int line) 00155 { 00156 return cpputest_realloc_location_with_leak_detection(memory, size, file, line); 00157 } 00158 00159 void cpputest_free_location(void* buffer, const char* file, int line) 00160 { 00161 cpputest_free_location_with_leak_detection(buffer, file, line); 00162 } 00163 00164 }
Generated on Tue Jul 12 2022 18:58:09 by
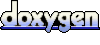