CppUTest is a C /C++ based unit xUnit test framework for unit testing and for test-driving your code.
Embed:
(wiki syntax)
Show/hide line numbers
CommandLineArguments.cpp
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #include "CppUTest/TestHarness.h" 00029 #include "CppUTest/CommandLineArguments.h" 00030 #include "CppUTest/PlatformSpecificFunctions.h" 00031 00032 CommandLineArguments::CommandLineArguments(int ac, const char** av) : 00033 ac_(ac), av_(av), verbose_(false), runTestsAsSeperateProcess_(false), repeat_(1), groupFilter_(""), nameFilter_(""), outputType_(OUTPUT_ECLIPSE), packageName_("") 00034 { 00035 } 00036 00037 CommandLineArguments::~CommandLineArguments() 00038 { 00039 } 00040 00041 bool CommandLineArguments::parse(TestPlugin* plugin) 00042 { 00043 bool correctParameters = true; 00044 for (int i = 1; i < ac_; i++) { 00045 SimpleString argument = av_[i]; 00046 if (argument == "-v") verbose_ = true; 00047 else if (argument == "-p") runTestsAsSeperateProcess_ = true; 00048 else if (argument.startsWith("-r")) SetRepeatCount(ac_, av_, i); 00049 else if (argument.startsWith("-g")) SetGroupFilter(ac_, av_, i); 00050 else if (argument.startsWith("-sg")) SetStrictGroupFilter(ac_, av_, i); 00051 else if (argument.startsWith("-n")) SetNameFilter(ac_, av_, i); 00052 else if (argument.startsWith("-sn")) SetStrictNameFilter(ac_, av_, i); 00053 else if (argument.startsWith("TEST(")) SetTestToRunBasedOnVerboseOutput(ac_, av_, i, "TEST("); 00054 else if (argument.startsWith("IGNORE_TEST(")) SetTestToRunBasedOnVerboseOutput(ac_, av_, i, "IGNORE_TEST("); 00055 else if (argument.startsWith("-o")) correctParameters = SetOutputType(ac_, av_, i); 00056 else if (argument.startsWith("-p")) correctParameters = plugin->parseAllArguments(ac_, av_, i); 00057 else if (argument.startsWith("-k")) SetPackageName(ac_, av_, i); 00058 else correctParameters = false; 00059 00060 if (correctParameters == false) { 00061 return false; 00062 } 00063 } 00064 return true; 00065 } 00066 00067 const char* CommandLineArguments::usage() const 00068 { 00069 return "usage [-v] [-r#] [-g|sg groupName] [-n|sn testName] [-o{normal, junit}] [-k packageName]\n"; 00070 } 00071 00072 bool CommandLineArguments::isVerbose() const 00073 { 00074 return verbose_; 00075 } 00076 00077 bool CommandLineArguments::runTestsInSeperateProcess() const 00078 { 00079 return runTestsAsSeperateProcess_; 00080 } 00081 00082 00083 int CommandLineArguments::getRepeatCount() const 00084 { 00085 return repeat_; 00086 } 00087 00088 TestFilter CommandLineArguments::getGroupFilter() const 00089 { 00090 return groupFilter_; 00091 } 00092 00093 TestFilter CommandLineArguments::getNameFilter() const 00094 { 00095 return nameFilter_; 00096 } 00097 00098 void CommandLineArguments::SetRepeatCount(int ac, const char** av, int& i) 00099 { 00100 repeat_ = 0; 00101 00102 SimpleString repeatParameter(av[i]); 00103 if (repeatParameter.size() > 2) repeat_ = PlatformSpecificAtoI(av[i] + 2); 00104 else if (i + 1 < ac) { 00105 repeat_ = PlatformSpecificAtoI(av[i + 1]); 00106 if (repeat_ != 0) i++; 00107 } 00108 00109 if (0 == repeat_) repeat_ = 2; 00110 00111 } 00112 00113 SimpleString CommandLineArguments::getParameterField(int ac, const char** av, int& i, const SimpleString& parameterName) 00114 { 00115 size_t parameterLength = parameterName.size(); 00116 SimpleString parameter(av[i]); 00117 if (parameter.size() > parameterLength) return av[i] + parameterLength; 00118 else if (i + 1 < ac) return av[++i]; 00119 return ""; 00120 } 00121 00122 void CommandLineArguments::SetGroupFilter(int ac, const char** av, int& i) 00123 { 00124 groupFilter_ = TestFilter(getParameterField(ac, av, i, "-g")); 00125 } 00126 00127 void CommandLineArguments::SetStrictGroupFilter(int ac, const char** av, int& i) 00128 { 00129 groupFilter_ = TestFilter(getParameterField(ac, av, i, "-sg")); 00130 groupFilter_.strictMatching(); 00131 } 00132 00133 void CommandLineArguments::SetNameFilter(int ac, const char** av, int& i) 00134 { 00135 nameFilter_ = getParameterField(ac, av, i, "-n"); 00136 } 00137 00138 void CommandLineArguments::SetStrictNameFilter(int ac, const char** av, int& index) 00139 { 00140 nameFilter_ = getParameterField(ac, av, index, "-sn"); 00141 nameFilter_.strictMatching(); 00142 } 00143 00144 void CommandLineArguments::SetTestToRunBasedOnVerboseOutput(int ac, const char** av, int& index, const char* parameterName) 00145 { 00146 SimpleString wholename = getParameterField(ac, av, index, parameterName); 00147 SimpleString testname = wholename.subStringFromTill(',', ')'); 00148 testname = testname.subString(2, testname.size()); 00149 groupFilter_ = wholename.subStringFromTill(wholename.at(0), ','); 00150 nameFilter_ = testname; 00151 nameFilter_.strictMatching(); 00152 groupFilter_.strictMatching(); 00153 } 00154 00155 void CommandLineArguments::SetPackageName(int ac, const char** av, int& i) 00156 { 00157 SimpleString packageName = getParameterField(ac, av, i, "-k"); 00158 if (packageName.size() == 0) return; 00159 00160 packageName_ = packageName; 00161 } 00162 00163 bool CommandLineArguments::SetOutputType(int ac, const char** av, int& i) 00164 { 00165 SimpleString outputType = getParameterField(ac, av, i, "-o"); 00166 if (outputType.size() == 0) return false; 00167 00168 if (outputType == "normal" || outputType == "eclipse") { 00169 outputType_ = OUTPUT_ECLIPSE; 00170 return true; 00171 } 00172 if (outputType == "junit") { 00173 outputType_ = OUTPUT_JUNIT; 00174 return true; 00175 } 00176 return false; 00177 } 00178 00179 bool CommandLineArguments::isEclipseOutput() const 00180 { 00181 return outputType_ == OUTPUT_ECLIPSE; 00182 } 00183 00184 bool CommandLineArguments::isJUnitOutput() const 00185 { 00186 return outputType_ == OUTPUT_JUNIT; 00187 } 00188 00189 const SimpleString& CommandLineArguments::getPackageName() const 00190 { 00191 return packageName_; 00192 } 00193
Generated on Tue Jul 12 2022 18:58:09 by
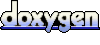