
brw1
Embed:
(wiki syntax)
Show/hide line numbers
TextDisplay.h
00001 /* mbed TextDisplay Library Base Class 00002 * Copyright (c) 2007-2009 sford 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 * 00005 * A common base class for Text displays 00006 * To port a new display, derive from this class and implement 00007 * the constructor (setup the display), character (put a character 00008 * at a location), rows and columns (number of rows/cols) functions. 00009 * Everything else (locate, printf, putc, cls) will come for free 00010 * 00011 * The model is the display will wrap at the right and bottom, so you can 00012 * keep writing and will always get valid characters. The location is 00013 * maintained internally to the class to make this easy 00014 */ 00015 00016 #ifndef MBED_TEXTDISPLAY_H 00017 #define MBED_TEXTDISPLAY_H 00018 00019 #include "mbed.h" 00020 00021 #include "DisplayDefs.h" 00022 00023 /// A text display class that supports character based 00024 /// presentation. 00025 /// 00026 class TextDisplay : public Stream 00027 { 00028 public: 00029 00030 // functions needing implementation in derived implementation class 00031 /// Create a TextDisplay interface 00032 /// 00033 /// @param name The name used in the path to access the display through 00034 /// the stdio stream. 00035 /// 00036 TextDisplay(const char *name = NULL); 00037 00038 /// output a character at the given position 00039 /// 00040 /// @note this method may be overridden in a derived class. 00041 /// 00042 /// @param[in] x position in pixels 00043 /// @param[in] y position in pixels 00044 /// @param[in] c the character to be written to the TextDisplay 00045 /// @returns number of pixels to advance the cursor which could be the cell width 00046 /// for non-proportional characters, or the actual character width for 00047 /// proportional characters. 00048 /// 00049 virtual int character(int x, int y, int c) = 0; 00050 00051 /// return number of rows on TextDisplay 00052 /// 00053 /// @note this method may be overridden in a derived class. 00054 /// 00055 /// @returns number of text rows for the display for the currently 00056 /// active font. 00057 /// 00058 virtual int rows() = 0; 00059 00060 /// return number if columns on TextDisplay 00061 /// 00062 /// @note this method may be overridden in a derived class. 00063 /// 00064 /// @returns number of text rows for the display for the currently 00065 /// active font. 00066 /// 00067 virtual int columns() = 0; 00068 00069 // functions that come for free, but can be overwritten 00070 00071 /// redirect output from a stream (stoud, sterr) to display 00072 /// 00073 /// @note this method may be overridden in a derived class. 00074 /// 00075 /// @param[in] stream that shall be redirected to the TextDisplay 00076 /// @returns true if the claim succeeded. 00077 /// 00078 virtual bool claim (FILE *stream); 00079 00080 /// clear screen 00081 /// 00082 /// @note this method may be overridden in a derived class. 00083 /// 00084 /// @param[in] layers is ignored, but supports maintaining the same 00085 /// API for the graphics layer. 00086 /// @returns error code. 00087 /// 00088 virtual RetCode_t cls(uint16_t layers = 0) = 0; 00089 00090 /// locate the cursor at a character position. 00091 /// 00092 /// Based on the currently active font, locate the cursor on screen. 00093 /// 00094 /// @note this method may be overridden in a derived class. 00095 /// 00096 /// @param[in] column is the horizontal offset from the left side. 00097 /// @param[in] row is the vertical offset from the top. 00098 /// @returns error code. 00099 /// 00100 virtual RetCode_t locate(textloc_t column, textloc_t row) = 0; 00101 00102 /// set the foreground color 00103 /// 00104 /// @note this method may be overridden in a derived class. 00105 /// 00106 /// @param[in] color is color to use for foreground drawing. 00107 /// @returns error code. 00108 /// 00109 virtual RetCode_t foreground(color_t color) = 0; 00110 00111 /// set the background color 00112 /// 00113 /// @note this method may be overridden in a derived class. 00114 /// 00115 /// @param[in] color is color to use for background drawing. 00116 /// @returns error code. 00117 /// 00118 virtual RetCode_t background(color_t color) = 0; 00119 // putc (from Stream) 00120 // printf (from Stream) 00121 00122 protected: 00123 virtual int _putc(int value); 00124 virtual int _getc(); 00125 00126 // character location 00127 uint16_t _column; 00128 uint16_t _row; 00129 00130 // colors 00131 color_t _foreground; 00132 color_t _background; 00133 char *_path; 00134 }; 00135 00136 #endif
Generated on Fri Jul 15 2022 05:44:41 by
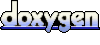