
brw1
Embed:
(wiki syntax)
Show/hide line numbers
GraphicsDisplay.h
00001 /* mbed GraphicsDisplay Display Library Base Class 00002 * Copyright (c) 2007-2009 sford 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 * 00005 * A library for providing a common base class for Graphics displays 00006 * To port a new display, derive from this class and implement 00007 * the constructor (setup the display), pixel (put a pixel 00008 * at a location), width and height functions. Everything else 00009 * (locate, printf, putc, cls, window, putp, fill, blit, blitbit) 00010 * will come for free. You can also provide a specialised implementation 00011 * of window and putp to speed up the results 00012 */ 00013 00014 #ifndef MBED_GRAPHICSDISPLAY_H 00015 #define MBED_GRAPHICSDISPLAY_H 00016 #include "Bitmap.h" 00017 #include "TextDisplay.h" 00018 00019 // GraphicsDisplay has one "soft font" which is in a different format 00020 // then the primary font rendering api - see set_font(...). This is 00021 // therefore deprecated, but preserved for a time for backward 00022 // compatibility. 00023 // #define LOCALFONT 00024 00025 00026 /// The GraphicsDisplay class 00027 /// 00028 /// This graphics display class supports both graphics and text operations. 00029 /// Typically, a subclass is derived from this which has localizations to 00030 /// adapt to a specific hardware platform (e.g. a display controller chip), 00031 /// that overrides methods in here to either add more capability or perhaps 00032 /// to improve performance, by leveraging specific hardware capabilities. 00033 /// 00034 class GraphicsDisplay : public TextDisplay 00035 { 00036 public: 00037 /// The constructor 00038 GraphicsDisplay(const char* name); 00039 00040 /// Draw a pixel in the specified color. 00041 /// 00042 /// @note this method must be supported in the derived class. 00043 /// 00044 /// @param[in] x is the horizontal offset to this pixel. 00045 /// @param[in] y is the vertical offset to this pixel. 00046 /// @param[in] color defines the color for the pixel. 00047 /// @returns success/failure code. @see RetCode_t. 00048 /// 00049 virtual RetCode_t pixel(loc_t x, loc_t y, color_t color) = 0; 00050 00051 /// Write a stream of pixels to the display. 00052 /// 00053 /// @note this method must be supported in the derived class. 00054 /// 00055 /// @param[in] p is a pointer to a color_t array to write. 00056 /// @param[in] count is the number of pixels to write. 00057 /// @param[in] x is the horizontal position on the display. 00058 /// @param[in] y is the vertical position on the display. 00059 /// @returns success/failure code. @see RetCode_t. 00060 /// 00061 virtual RetCode_t pixelStream(color_t * p, uint32_t count, loc_t x, loc_t y) = 0; 00062 00063 /// Get a pixel from the display. 00064 /// 00065 /// @note this method must be supported in the derived class. 00066 /// 00067 /// @param[in] x is the horizontal offset to this pixel. 00068 /// @param[in] y is the vertical offset to this pixel. 00069 /// @returns the pixel. see @color_t 00070 /// 00071 virtual color_t getPixel(loc_t x, loc_t y) = 0; 00072 00073 /// Get a stream of pixels from the display. 00074 /// 00075 /// @note this method must be supported in the derived class. 00076 /// 00077 /// @param[out] p is a pointer to a color_t array to accept the stream. 00078 /// @param[in] count is the number of pixels to read. 00079 /// @param[in] x is the horizontal offset to this pixel. 00080 /// @param[in] y is the vertical offset to this pixel. 00081 /// @returns success/failure code. @see RetCode_t. 00082 /// 00083 virtual RetCode_t getPixelStream(color_t * p, uint32_t count, loc_t x, loc_t y) = 0; 00084 00085 /// get the screen width in pixels 00086 /// 00087 /// @note this method must be supported in the derived class. 00088 /// 00089 /// @returns screen width in pixels. 00090 /// 00091 virtual uint16_t width() = 0; 00092 00093 /// get the screen height in pixels 00094 /// 00095 /// @note this method must be supported in the derived class. 00096 /// 00097 /// @returns screen height in pixels. 00098 /// 00099 virtual uint16_t height() = 0; 00100 00101 /// Prepare the controller to write binary data to the screen by positioning 00102 /// the memory cursor. 00103 /// 00104 /// @note this method must be supported in the derived class. 00105 /// 00106 /// @param[in] x is the horizontal position in pixels (from the left edge) 00107 /// @param[in] y is the vertical position in pixels (from the top edge) 00108 /// @returns success/failure code. @see RetCode_t. 00109 /// 00110 virtual RetCode_t SetGraphicsCursor(loc_t x, loc_t y) = 0; 00111 00112 /// Prepare the controller to read binary data from the screen by positioning 00113 /// the memory read cursor. 00114 /// 00115 /// @param[in] x is the horizontal position in pixels (from the left edge) 00116 /// @param[in] y is the vertical position in pixels (from the top edge) 00117 /// @returns success/failure code. @see RetCode_t. 00118 /// 00119 virtual RetCode_t SetGraphicsCursorRead(loc_t x, loc_t y) = 0; 00120 00121 /// Draw a filled rectangle in the specified color 00122 /// 00123 /// @note As a side effect, this changes the current 00124 /// foreground color for subsequent operations. 00125 /// 00126 /// @note this method must be supported in the derived class. 00127 /// 00128 /// @param[in] x1 is the horizontal start of the line. 00129 /// @param[in] y1 is the vertical start of the line. 00130 /// @param[in] x2 is the horizontal end of the line. 00131 /// @param[in] y2 is the vertical end of the line. 00132 /// @param[in] color defines the foreground color. 00133 /// @param[in] fillit is optional to NOFILL the rectangle. default is FILL. 00134 /// @returns success/failure code. @see RetCode_t. 00135 /// 00136 virtual RetCode_t fillrect(loc_t x1, loc_t y1, loc_t x2, loc_t y2, 00137 color_t color, fill_t fillit = FILL) = 0; 00138 00139 00140 virtual RetCode_t WriteCommand(unsigned char command, unsigned int data = 0xFFFF) = 0; 00141 virtual RetCode_t WriteData(unsigned char data) = 0; 00142 00143 /// Set the window, which controls where items are written to the screen. 00144 /// 00145 /// When something hits the window width, it wraps back to the left side 00146 /// and down a row. If the initial write is outside the window, it will 00147 /// be captured into the window when it crosses a boundary. 00148 /// 00149 /// @param[in] x is the left edge in pixels. 00150 /// @param[in] y is the top edge in pixels. 00151 /// @param[in] w is the window width in pixels. 00152 /// @param[in] h is the window height in pixels. 00153 /// @returns success/failure code. @see RetCode_t. 00154 /// 00155 virtual RetCode_t window(loc_t x, loc_t y, dim_t w, dim_t h); 00156 00157 /// Clear the screen. 00158 /// 00159 /// The behavior is to clear the whole screen. 00160 /// 00161 /// @param[in] layers is ignored, but supports maintaining the same 00162 /// API for the graphics layer. 00163 /// @returns success/failure code. @see RetCode_t. 00164 /// 00165 virtual RetCode_t cls(uint16_t layers = 0); 00166 00167 /// method to set the window region to the full screen. 00168 /// 00169 /// This restores the 'window' to the full screen, so that 00170 /// other operations (@see cls) would clear the whole screen. 00171 /// 00172 /// @returns success/failure code. @see RetCode_t. 00173 /// 00174 virtual RetCode_t WindowMax(void); 00175 00176 /// method to put a single color pixel to the screen. 00177 /// 00178 /// This method may be called as many times as necessary after 00179 /// @see _StartGraphicsStream() is called, and it should be followed 00180 /// by _EndGraphicsStream. 00181 /// 00182 /// @param[in] pixel is a color value to be put on the screen. 00183 /// @returns success/failure code. @see RetCode_t. 00184 /// 00185 virtual RetCode_t _putp(color_t pixel); 00186 00187 /// method to fill a region. 00188 /// 00189 /// This method fills a region with the specified color. 00190 /// 00191 /// @param[in] x is the left-edge of the region. 00192 /// @param[in] y is the top-edge of the region. 00193 /// @param[in] w specifies the width of the region. 00194 /// @param[in] h specifies the height of the region. 00195 /// @returns success/failure code. @see RetCode_t. 00196 /// 00197 virtual RetCode_t fill(int x, int y, int w, int h, color_t color); 00198 00199 00200 virtual RetCode_t blit(int x, int y, int w, int h, const int * color); 00201 00202 /// This method transfers one character from the external font data 00203 /// to the screen. 00204 /// 00205 /// @note the font data is in a special format as generate by 00206 /// the mikroe font creator. \\ 00207 /// See http://www.mikroe.com/glcd-font-creator/ 00208 /// 00209 /// @param[in] x is the horizontal pixel coordinate 00210 /// @param[in] y is the vertical pixel coordinate 00211 /// @param[in] fontTable is the base of the table which has the metrics 00212 /// @param[in] fontChar is the start of that record in the table for the char (e.g. 'A' - 'Z') 00213 /// @returns how far the cursor should advance to the right in pixels 00214 /// 00215 virtual int fontblit(int x, int y, const unsigned char * fontTable, const unsigned char * fontChar); 00216 00217 /// This method returns the color value from a palette. 00218 /// 00219 /// This method accepts a pointer to a Bitmap color palette, which 00220 /// is a table in memory composed of RGB Quad values (r, g, b, 0), 00221 /// and an index into that table. It then extracts the color information 00222 /// and downsamples it to a color_t value which it returns. 00223 /// 00224 /// @note This method probably has very little value outside of 00225 /// the internal methods for reading BMP files. 00226 /// 00227 /// @param[in] colorPaletteArray is the handle to the color palette array to use. 00228 /// @param[in] index is the index into the color palette. 00229 /// @returns the color in color_t format. 00230 /// 00231 color_t RGBQuadToRGB16(RGBQUAD * colorPaletteArray, uint16_t index); 00232 00233 /// This method converts a 16-bit color value into a 24-bit RGB Quad. 00234 /// 00235 /// @param[in] c is the 16-bit color. @see color_t. 00236 /// @returns an RGBQUAD value. @see RGBQUAD 00237 /// 00238 RGBQUAD RGB16ToRGBQuad(color_t c); 00239 00240 /// This method attempts to render a specified graphics image file at 00241 /// the specified screen location. 00242 /// 00243 /// This supports several variants of the following file types: 00244 /// \li Bitmap file format, 00245 /// \li Icon file format. 00246 /// 00247 /// @note The specified image width and height, when adjusted for the 00248 /// x and y origin, must fit on the screen, or the image will not 00249 /// be shown (it does not clip the image). 00250 /// 00251 /// @note The file extension is tested, and if it ends in a supported 00252 /// format, the appropriate handler is called to render that image. 00253 /// 00254 /// @param[in] x is the horizontal pixel coordinate 00255 /// @param[in] y is the vertical pixel coordinate 00256 /// @param[in] FileName refers to the fully qualified path and file on 00257 /// a mounted file system. 00258 /// @returns success or error code. 00259 /// 00260 RetCode_t RenderImageFile(loc_t x, loc_t y, const char *FileName); 00261 00262 /// This method reads a disk file that is in bitmap format and 00263 /// puts it on the screen. 00264 /// 00265 /// Supported formats: 00266 /// \li 4-bit color format (16 colors) 00267 /// \li 8-bit color format (256 colors) 00268 /// \li 16-bit color format (65k colors) 00269 /// \li compression: no. 00270 /// 00271 /// @note This is a slow operation, typically due to the use of 00272 /// the file system, and partially because bmp files 00273 /// are stored from the bottom up, and the memory is written 00274 /// from the top down; as a result, it constantly 'seeks' 00275 /// on the file system for the next row of information. 00276 /// 00277 /// As a performance test, a sample picture was timed. A family picture 00278 /// was converted to Bitmap format; shrunk to 352 x 272 pixels and save 00279 /// in 8-bit color format. The resulting file size was 94.5 KByte. 00280 /// The SPI port interface was set to 20 MHz. 00281 /// The original bitmap rendering software was purely in software, 00282 /// pushing 1 pixel at a time to the write function, which did use SPI 00283 /// hardware (not pin wiggling) to transfer commands and data to the 00284 /// display. Then, the driver was improved to leverage the capability 00285 /// of the derived display driver. As a final check, instead of the 00286 /// [known slow] local file system, a randomly chosen USB stick was 00287 /// used. The performance results are impressive (but depend on the 00288 /// listed factors). 00289 /// 00290 /// \li 34 seconds, LocalFileSystem, Software Rendering 00291 /// \li 9 seconds, LocalFileSystem, Hardware Rending for RA8875 00292 /// \li 3 seconds, MSCFileSystem, Hardware Rendering for RA8875 00293 /// 00294 /// @param[in] x is the horizontal pixel coordinate 00295 /// @param[in] y is the vertical pixel coordinate 00296 /// @param[in] Name_BMP is the filename on the mounted file system. 00297 /// @returns success or error code. 00298 /// 00299 RetCode_t RenderBitmapFile(loc_t x, loc_t y, const char *Name_BMP); 00300 00301 00302 /// This method reads a disk file that is in ico format and 00303 /// puts it on the screen. 00304 /// 00305 /// Reading the disk is slow, but a typical icon file is small 00306 /// so it should be ok. 00307 /// 00308 /// @note An Icon file can have more than one icon in it. This 00309 /// implementation only processes the first image in the file. 00310 /// 00311 /// @param[in] x is the horizontal pixel coordinate 00312 /// @param[in] y is the vertical pixel coordinate 00313 /// @param[in] Name_ICO is the filename on the mounted file system. 00314 /// @returns success or error code. 00315 /// 00316 RetCode_t RenderIconFile(loc_t x, loc_t y, const char *Name_ICO); 00317 00318 00319 /// prints one character at the specified coordinates. 00320 /// 00321 /// This will print the character at the specified pixel coordinates. 00322 /// 00323 /// @param[in] x is the horizontal offset in pixels. 00324 /// @param[in] y is the vertical offset in pixels. 00325 /// @param[in] value is the character to print. 00326 /// @returns number of pixels to index to the right if a character was printed, 0 otherwise. 00327 /// 00328 virtual int character(int x, int y, int value); 00329 00330 /// get the number of colums based on the currently active font 00331 /// 00332 /// @returns number of columns. 00333 /// 00334 virtual int columns(void); 00335 00336 /// get the number of rows based on the currently active font 00337 /// 00338 /// @returns number of rows. 00339 /// 00340 virtual int rows(void); 00341 00342 /// Select a bitmap font (provided by the user) for all subsequent text 00343 /// rendering. 00344 /// 00345 /// This API permits selection of a special memory mapped font, which 00346 /// enables the presentation of many font sizes and styles, including 00347 /// proportional fonts. 00348 /// 00349 /// @note Tool to create the fonts is accessible from its creator 00350 /// available at http://www.mikroe.com. 00351 /// Hint: Change the data to an array of type char[]. 00352 /// 00353 /// This special font array has a 4-byte header, followed by 00354 /// the data: 00355 /// \li the number of bytes per char 00356 /// \li the vertical size in pixels for each character 00357 /// \li the horizontal size in pixels for each character 00358 /// \li the number of bytes per vertical line (width of the array) 00359 /// \li the subsequent records are the font information. 00360 /// 00361 /// @param[in] font is a pointer to a specially formed font array. 00362 /// NULL, or the omission of this parameter will restore the default 00363 /// font capability, which may use the display controllers hardware 00364 /// font (if available), or no font. 00365 /// @returns error code. 00366 /// 00367 virtual RetCode_t set_font(const unsigned char * font = NULL); 00368 00369 protected: 00370 00371 /// Pure virtual method indicating the start of a graphics stream. 00372 /// 00373 /// This is called prior to a stream of pixel data being sent. 00374 /// This may cause register configuration changes in the derived 00375 /// class in order to prepare the hardware to accept the streaming 00376 /// data. 00377 /// 00378 /// @note this method must be supported in the derived class. 00379 /// 00380 /// @returns error code. 00381 /// 00382 virtual RetCode_t _StartGraphicsStream(void) = 0; 00383 00384 /// Pure virtual method indicating the end of a graphics stream. 00385 /// 00386 /// This is called to conclude a stream of pixel data that was sent. 00387 /// This may cause register configuration changes in the derived 00388 /// class in order to stop the hardware from accept the streaming 00389 /// data. 00390 /// 00391 /// @note this method must be supported in the derived class. 00392 /// 00393 /// @returns error code. 00394 /// 00395 virtual RetCode_t _EndGraphicsStream(void) = 0; 00396 00397 /// Protected method to render an image given a file handle and 00398 /// coordinates. 00399 /// 00400 /// @param[in] x is the horizontal pixel coordinate 00401 /// @param[in] y is the vertical pixel coordinate 00402 /// @param[in] w is the image width restriction, or zero to permit full image width. 00403 /// @param[in] h is the image height restriction, or zero to permit full image height. 00404 /// @param[in] fileOffset is the offset into the file where the image data starts 00405 /// @param[in] Image is the filename stream already opened for the data. 00406 /// @returns success or error code. 00407 /// 00408 RetCode_t _RenderBitmap(loc_t x, loc_t y, uint32_t fileOffset, FILE * Image); 00409 00410 #ifdef LOCALFONT 00411 virtual int blitbit(int x, int y, int w, int h, const char * color); 00412 #endif 00413 00414 const unsigned char * font; ///< reference to an external font somewhere in memory 00415 00416 // pixel location 00417 short _x; 00418 short _y; 00419 00420 // window location 00421 short _x1; 00422 short _x2; 00423 short _y1; 00424 short _y2; 00425 }; 00426 00427 #endif 00428
Generated on Fri Jul 15 2022 05:44:41 by
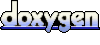