
brw1
Embed:
(wiki syntax)
Show/hide line numbers
DisplayDefs.h
00001 #ifndef DISPLAYDEFS_H 00002 #define DISPLAYDEFS_H 00003 00004 #define RGB(r,g,b) ( ((r<<8)&0xF800) | ((g<<3)&0x07E0) | (b>>3) ) 00005 00006 typedef uint16_t color_t; 00007 00008 /// return values from functions. Use this number, or use the 00009 /// lookup function to get a text string. @see GetErrorMessage. 00010 /// 00011 typedef enum 00012 { 00013 noerror, ///< no errors, command completed successfully 00014 bad_parameter, ///< one or more parameters are invalid 00015 file_not_found, ///< specified file could not be found 00016 not_bmp_format, ///< file is not a .bmp file 00017 not_ico_format, ///< file is not a .ico file 00018 not_supported_format, ///< file format is not yet supported 00019 image_too_big, ///< image is too large for the screen 00020 not_enough_ram, ///< could not allocate ram for scanline 00021 LastErrCode, // Private marker. 00022 } RetCode_t; 00023 00024 /// type that manages locations, which is typically an x or y pixel location, 00025 /// which can range from -N to +N (even if the screen is 0 to +n). @see textloc_t. 00026 typedef int16_t loc_t; 00027 00028 /// type that manages text locations, which are row or column values in 00029 /// units of character, not pixel. @see loc_t. 00030 typedef uint16_t textloc_t; 00031 00032 /// type that manages dimensions of width or height, which range from 0 to N. 00033 typedef uint16_t dim_t; 00034 00035 /// type that manages x,y pairs 00036 typedef struct 00037 { 00038 loc_t x; ///< x value in the point 00039 loc_t y; ///< y value in the point 00040 } point_t; 00041 00042 /// type that manages rectangles, which are pairs of points. It is recommended 00043 /// that p1 contains the top-left point and p2 contains the bottom-right point, 00044 /// even though eventually this should not matter. 00045 typedef struct 00046 { 00047 point_t p1; ///< p1 defines one point on the rectangle 00048 point_t p2; ///< p2 defines the opposite point on the rectangle 00049 } rect_t; 00050 00051 typedef struct 00052 { 00053 int32_t An, Bn, Cn, Dn, En, Fn, Divider; 00054 } tpMatrix_t; 00055 00056 /// color type definition to let the compiler help keep us honest. 00057 /// 00058 /// colors can be defined with the RGB(r,g,b) macro, and there 00059 /// are a number of predefined colors: 00060 /// - Black, Blue, Green, Cyan, 00061 /// - Red, Magenta, Brown, Gray, 00062 /// - Charcoal, BrightBlue, BrightGreen, BrightCyan, 00063 /// - Orange, Pink, Yellow, White 00064 /// 00065 typedef uint16_t color_t; 00066 00067 /// background fill info for drawing Text, Rectangles, RoundedRectanges, Circles, Ellipses and Triangles. 00068 typedef enum 00069 { 00070 NOFILL, ///< do not fill the object with the background color 00071 FILL ///< fill the object space with the background color 00072 } fill_t; 00073 00074 #endif // DISPLAYDEFS_H
Generated on Fri Jul 15 2022 05:44:41 by
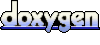