
brw1
Embed:
(wiki syntax)
Show/hide line numbers
Bitmap.h
00001 // 00002 // Windows BMP file definitions. 00003 // 00004 // Adapted from code written by Michael Sweet from Paul Bourke's 00005 // web site: http://paulbourke.net/dataformats/bmp/ 00006 // 00007 00008 #ifndef _BITMAP_H_ 00009 #define _BITMAP_H_ 00010 00011 #include <mbed.h> 00012 00013 // BITMAPFILEHEADER 00014 // BITMAPINFOHEADER 00015 // Optional Palette 00016 // Raw Data 00017 00018 // 00019 // Bitmap file data structures 00020 // 00021 // must align to 2-byte boundaries so it doesn't alter the memory image when 00022 // bytes are read from the file system into this footprint. 00023 #pragma push 00024 #pragma pack(2) 00025 00026 typedef struct /**** BMP file header structure ****/ 00027 { 00028 uint16_t bfType; /* Magic number for file */ 00029 uint32_t bfSize; /* Size of file */ 00030 uint16_t bfReserved1; /* Reserved */ 00031 uint16_t bfReserved2; /* ... */ 00032 uint32_t bfOffBits; /* Offset to bitmap data */ 00033 } BITMAPFILEHEADER; 00034 00035 typedef struct /**** BMP file info structure ****/ 00036 { 00037 uint32_t biSize; /* Size of info header */ 00038 uint32_t biWidth; /* Width of image */ 00039 uint32_t biHeight; /* Height of image */ 00040 uint16_t biPlanes; /* Number of color planes */ 00041 uint16_t biBitCount; /* Number of bits per pixel */ 00042 uint32_t biCompression; /* Type of compression to use */ 00043 uint32_t biSizeImage; /* Size of image data */ 00044 int32_t biXPelsPerMeter; /* X pixels per meter */ 00045 int32_t biYPelsPerMeter; /* Y pixels per meter */ 00046 uint32_t biClrUsed; /* Number of colors used */ 00047 uint32_t biClrImportant; /* Number of important colors */ 00048 } BITMAPINFOHEADER; 00049 #pragma pop 00050 00051 #define BF_TYPE 0x4D42 /* "MB" */ 00052 00053 /* 00054 * Constants for the biCompression field... 00055 */ 00056 00057 # define BI_RGB 0 /* No compression - straight BGR data */ 00058 # define BI_RLE8 1 /* 8-bit run-length compression */ 00059 # define BI_RLE4 2 /* 4-bit run-length compression */ 00060 # define BI_BITFIELDS 3 /* RGB bitmap with RGB masks */ 00061 00062 typedef struct /**** Colormap entry structure ****/ 00063 { 00064 uint8_t rgbBlue; /* Blue value */ 00065 uint8_t rgbGreen; /* Green value */ 00066 uint8_t rgbRed; /* Red value */ 00067 uint8_t rgbReserved; /* Reserved */ 00068 } RGBQUAD; 00069 00070 //typedef struct /**** Bitmap information structure ****/ 00071 // { 00072 // BITMAPINFOHEADER bmiHeader; /* Image header */ 00073 // RGBQUAD bmiColors[256]; /* Image colormap */ 00074 // } BITMAPINFO; 00075 00076 00077 #pragma push 00078 #pragma pack(2) 00079 00080 typedef struct /**** ICO file header structure ****/ 00081 { 00082 uint16_t Reserved_zero; // Always zero 00083 uint16_t icType; // 1 for .ico, 2 for .cur 00084 uint16_t icImageCount; // number of images in the file 00085 } ICOFILEHEADER; 00086 00087 typedef struct /**** ICO file Directory Entry structure (1 or more) ****/ 00088 { 00089 uint8_t biWidth; /* Width of image */ 00090 uint8_t biHeight; /* Height of image */ 00091 uint8_t biClrUsed; /* Number of colors used */ 00092 uint8_t Reserved_zero; 00093 uint16_t biPlanes; /* Number of color planes (ICO should be 0 or 1, CUR horz hotspot */ 00094 uint16_t biBitCount; /* Number of bits per pixel (ICO bits per pixel, CUR vert hotspot */ 00095 uint32_t biSizeImage; /* Size of image data */ 00096 uint32_t bfOffBits; /* Offset into file for the bitmap data */ 00097 } ICODIRENTRY; 00098 #pragma pop 00099 00100 #define IC_TYPE 0x0001 /* 1 = ICO (icon), 2 = CUR (cursor) */ 00101 00102 #endif // _BITMAP_H_
Generated on Fri Jul 15 2022 05:44:41 by
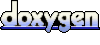