I am in my way
Dependencies: ublox-at-cellular-interface ublox-cellular-base C12832 LM75B ublox-cellular-base-n2xx ublox-at-cellular-interface-n2xx
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 u-blox 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 /* This example program for the u-blox C030 board. 00019 * The UbloxATCellularInterface or OnboardCellularInterface uses it 00020 * to make a simple HTTP connection to a STC IOT server. 00021 * Progress may be monitored with a serial terminal running at 9600 baud or at https://ksu.aep1.iot.stcs.com.sa 00022 * The LED on the C030 board will turn green when this program is 00023 * operating correctly, pulse blue when a sockets operation is completed 00024 * and turn red if there is a failure. 00025 00026 * Modified by: Muhammad Faheem Khan (Digital Solutions, STCS) 00027 */ 00028 00029 #include "mbed.h" 00030 #include "UbloxATCellularInterface.h" 00031 #include "UbloxATCellularInterfaceN2xx.h" 00032 #include "LM75B.h" // Temperature Sensor Library. Import more libraries from https://os.mbed.com/components/mbed-Application-Shield/ 00033 #include "C12832.h" // LCD Library. Import more libraries from https://os.mbed.com/components/mbed-Application-Shield/ 00034 00035 // Sensor Pin and Object Activation 00036 C12832 lcd(D11, D13, D12, D7, D10); 00037 LM75B sensor(D14,D15); // 1st Temperature sensor 00038 //AnalogIn pot2 (A1); // 2nd Potentiometer sensor 00039 AnalogIn moisture(A0); 00040 #define INTERFACE_CLASS UbloxATCellularInterface //This interface is used to initiate modem commands for sending data to sever 00041 00042 // The credentials of the SIM in the board. If PIN checking is enabled 00043 // for your SIM card you must set this to the required PIN. 00044 #define PIN "0000" 00045 #define APN "jtm2m" //APN remains the same for all the kits 00046 #define USERNAME NULL 00047 #define PASSWORD NULL 00048 #define TCP_SERVER "integration.campus.stcs.io" //STC IoT parsing server address 00049 00050 // LEDs 00051 DigitalOut ledRed(LED1, 1); 00052 DigitalOut ledGreen(LED2, 1); 00053 DigitalOut ledBlue(LED3, 1); 00054 00055 // The user button 00056 volatile bool buttonPressed = false; 00057 00058 00059 float map(float in, float inMin, float inMax, float outMin, float outMax) { 00060 // check it's within the range 00061 if (inMin<inMax) { 00062 if (in <= inMin) 00063 return outMin; 00064 if (in >= inMax) 00065 return outMax; 00066 } else { // cope with input range being backwards. 00067 if (in >= inMin) 00068 return outMin; 00069 if (in <= inMax) 00070 return outMax; 00071 } 00072 // calculate how far into the range we are 00073 float scale = (in-inMin)/(inMax-inMin); 00074 // calculate the output. 00075 return outMin + scale*(outMax-outMin); 00076 } 00077 00078 static void good() { 00079 ledGreen = 0; 00080 ledBlue = 1; 00081 ledRed = 1; 00082 } 00083 00084 static void bad() { 00085 ledRed = 0; 00086 ledGreen = 1; 00087 ledBlue = 1; 00088 } 00089 00090 static void event() { 00091 ledBlue = 0; 00092 ledRed = 1; 00093 ledGreen = 1; 00094 } 00095 00096 static void pulseEvent() { 00097 event(); 00098 wait_ms(500); 00099 good(); 00100 } 00101 00102 static void ledOff() { 00103 ledBlue = 1; 00104 ledRed = 1; 00105 ledGreen = 1; 00106 } 00107 static void cbButton() 00108 { 00109 buttonPressed = true; 00110 pulseEvent(); 00111 } 00112 00113 int main() 00114 { 00115 INTERFACE_CLASS *interface = new INTERFACE_CLASS(); 00116 // If you need to debug the cellular interface, comment out the 00117 // instantiation above and uncomment the one below. 00118 // For the N2xx interface, change xxx to MBED_CONF_UBLOX_CELL_BAUD_RATE, 00119 // while for the non-N2xx interface change it to MBED_CONF_UBLOX_CELL_N2XX_BAUD_RATE. 00120 // INTERFACE_CLASS *interface = new INTERFACE_CLASS(MDMTXD, MDMRXD, 00121 // xxx, 00122 // true); 00123 #ifndef TARGET_UBLOX_C030_N211 00124 TCPSocket sockTcp; // for 1st sensor 00125 TCPSocket sockTcp1; // for 2nd Sensor 00126 #endif 00127 00128 SocketAddress tcpServer; 00129 char deviceID[20] = "357520073946010"; 00130 char buf[1024]; // this buffer is used to send POST data to platform 00131 char temp[50]; // this buffer will be used to store sensor values 00132 char soil[50]; // this buffer will be used to store 2nd sensor values 00133 int x; 00134 00135 #ifdef TARGET_UBLOX_C027 00136 // No user button on C027 00137 InterruptIn userButton(NC); 00138 #else 00139 InterruptIn userButton(SW0); 00140 #endif 00141 00142 // Attach a function to the user button 00143 userButton.rise(&cbButton); 00144 00145 good(); 00146 printf("Starting up, please wait up to 180 seconds for network registration to complete...\n"); 00147 interface->set_credentials(APN, USERNAME, PASSWORD); 00148 for (x = 0; interface->connect(PIN) != 0; x++) { 00149 if (x > 0) { 00150 bad(); 00151 printf("Retrying (have you checked that an antenna is plugged in and your APN is correct?)...\n"); 00152 } 00153 } 00154 pulseEvent(); 00155 00156 printf("Getting the IP address of \"integration.campus.stcs.io\"...\n"); 00157 if (interface->gethostbyname(TCP_SERVER, &tcpServer) == 0) { 00158 pulseEvent(); 00159 00160 tcpServer.set_port(1880); 00161 printf("\"integration.campus.stcs.io\" address: %s on port %d.\n", tcpServer.get_ip_address(), tcpServer.get_port()); 00162 00163 printf("Performing socket operations in a loop (until the user button is pressed on C030)...\n"); 00164 while (!buttonPressed) { 00165 00166 #ifndef TARGET_UBLOX_C030_N211 00167 lcd.cls(); 00168 lcd.locate(0,3); 00169 lcd.printf("Connecting"); 00170 00171 // TCP Sockets 00172 printf("=== TCP ===\n"); 00173 printf("Opening a TCP socket...\n"); 00174 if (sockTcp.open(interface) == 0 && sockTcp1.open(interface) == 0 ) { 00175 pulseEvent(); 00176 printf("TCP socket open.\n"); 00177 sockTcp.set_timeout(10000); 00178 printf("Connecting socket to %s on port %d...\n", tcpServer.get_ip_address(), tcpServer.get_port()); 00179 if (sockTcp.connect(tcpServer) == 0 && sockTcp1.connect(tcpServer) == 0) { 00180 pulseEvent(); 00181 00182 00183 lcd.cls(); 00184 lcd.locate(0,3); 00185 lcd.printf("Connected"); 00186 wait(2.0); 00187 printf("Connected, sending HTTP GET request to %s over socket...\n", TCP_SERVER); 00188 00189 00190 ////////////////// SENDING 1st SENSOR VALUE ///////////////////// 00191 00192 lcd.cls(); 00193 lcd.locate(0,3); 00194 00195 00196 snprintf(temp,sizeof temp,"Temperature=%.1f", sensor.temp()); //Sensor Key "Temperature" and Value is Sensor Data 00197 00198 lcd.printf("%s", temp); // Display Temperature value on LCD 00199 00200 00201 snprintf(buf, sizeof buf, "%s%s%s%s%s%s", "POST /ksu/?deviceID=",deviceID,"&",temp,"&unit=C"," HTTP/1.0\r\n\r\n"); 00202 00203 printf("Output string is %s",buf); 00204 00205 // Note: since this is a short string we can send it in one go as it will 00206 // fit within the default buffer sizes. Normally you should call sock.send() 00207 // in a loop until your entire buffer has been sent. 00208 if (sockTcp.send((void *) buf, strlen(buf)) == (int) strlen(buf)) { 00209 pulseEvent(); 00210 printf("Socket send completed, waiting for response...\n"); 00211 x = sockTcp.recv(buf, sizeof (buf)); 00212 if (x > 0) { 00213 pulseEvent(); 00214 printf("Received %d byte response from server on TCP socket:\n" 00215 "----------------------------------------------------\n%.*s" 00216 "----------------------------------------------------\n", 00217 x, x, buf); 00218 } 00219 } 00220 00221 00222 00223 00224 00225 00226 /////////////////////////////////// Sending 2nd Sensor Value ////////////////////// 00227 00228 00229 float final_2 = moisture.read() * 3300; 00230 uint16_t meas = (moisture.read_u16()); 00231 float final_1 = (float) 3300 / 65535 * (float) meas; 00232 float newval = map(final_1,660,770,100,0); 00233 00234 00235 lcd.cls(); 00236 lcd.locate(0,3); 00237 00238 snprintf(soil,sizeof soil,"Moisture=%.1f", (float)map(final_1,660,770,100,0)); //Sensor Key "Moisture" and Value is Sensor Data 00239 00240 lcd.printf("%s", soil); // Display Moisture value on LCD 00241 wait(2.0); 00242 00243 00244 snprintf(buf, sizeof buf, "%s%s%s%s%s%s", "POST /ksu/?deviceID=",deviceID,"&",soil,"&unit=%"," HTTP/1.0\r\n\r\n"); 00245 00246 printf("Output string is %s",buf); 00247 00248 // Note: since this is a short string we can send it in one go as it will 00249 // fit within the default buffer sizes. Normally you should call sock.send() 00250 // in a loop until your entire buffer has been sent. 00251 if (sockTcp1.send((void *) buf, strlen(buf)) == (int) strlen(buf)) { 00252 pulseEvent(); 00253 printf("Socket send completed, waiting for response...\n"); 00254 x = sockTcp1.recv(buf, sizeof (buf)); 00255 if (x > 0) { 00256 pulseEvent(); 00257 printf("Received %d byte response from server on TCP socket:\n" 00258 "----------------------------------------------------\n%.*s" 00259 "----------------------------------------------------\n", 00260 x, x, buf); 00261 } 00262 } 00263 00264 } 00265 lcd.cls(); 00266 lcd.locate(0,3); 00267 lcd.printf("Resend after 10s"); 00268 wait(1.0); 00269 printf("Closing socket...\n"); 00270 sockTcp.close(); 00271 sockTcp1.close(); 00272 pulseEvent(); 00273 printf("Socket closed.\n"); 00274 } 00275 #endif 00276 wait_ms(10000); //Loop delay in milliseconds. Keep it more than 10 seconds to save data usage on cellular 00277 #ifndef TARGET_UBLOX_C027 00278 printf("[Checking if user button has been pressed]\n"); 00279 #endif 00280 } 00281 00282 pulseEvent(); 00283 printf("User button was pressed, stopping...\n"); 00284 interface->disconnect(); 00285 ledOff(); 00286 printf("Stopped.\n"); 00287 } 00288 else { 00289 bad(); 00290 printf("Unable to get IP address of \"integraton.campus.stcs.io\".\n"); 00291 } 00292 } 00293 00294 00295 // End Of File
Generated on Wed Aug 3 2022 16:13:39 by
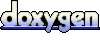