
This is a demonstration of using the tft display of the Cypress PSoC 6 WiFi-BT Pioneer kit. The demo displays the status of the capsense buttons, slider and user button on the tft display. Makes use of emwin, capsense and PinDetect libraries.
main.cpp
00001 #include "mbed.h" 00002 #include "GUI.h" 00003 #include "cy8ckit_028_tft.h" 00004 #define Cy_SysLib_Delay wait_ms 00005 #include "cy_pdl.h" 00006 #include "cycfg_capsense.h" 00007 #include "cycfg.h" 00008 #include "PinDetect.h" 00009 00010 DigitalOut ledGreen(LED_GREEN); 00011 DigitalIn sw2(SWITCH2, PullUp); 00012 DigitalOut ledRed(LED_RED); 00013 DigitalOut ledBlue(LED_BLUE); 00014 DigitalOut ledBlue2(P13_4); 00015 DigitalOut ledGreen2(P13_3); 00016 DigitalOut ledRed2(P13_2); 00017 00018 PinDetect pb2(P0_4); 00019 00020 /* Macros for switch press status */ 00021 #define BTN_PRESSED (0u) 00022 #define BTN_RELEASED (1u) 00023 00024 /*************************************************************************** 00025 * Global constants 00026 ***************************************************************************/ 00027 #define SLIDER_NUM_TOUCH (1u) /* Number of touches on the slider */ 00028 #define LED_OFF (1u) 00029 #define LED_ON (0u) 00030 #define CAPSENSE_SCAN_PERIOD_MS (20u) /* milliseconds */ 00031 00032 00033 /*************************************** 00034 * Function Prototypes 00035 **************************************/ 00036 void RunCapSenseScan(void); 00037 void InitTunerCommunication(void); 00038 void ProcessTouchStatus(void); 00039 void EZI2C_InterruptHandler(void); 00040 void CapSense_InterruptHandler(void); 00041 void CapSenseEndOfScanCallback(cy_stc_active_scan_sns_t * ptrActiveScan); 00042 void InitCapSenseClock(void); 00043 00044 00045 /******************************************************************************* 00046 * Interrupt configuration 00047 *******************************************************************************/ 00048 const cy_stc_sysint_t CapSense_ISR_cfg = 00049 { 00050 .intrSrc = CYBSP_CSD_IRQ, 00051 .intrPriority = 4u 00052 }; 00053 00054 const cy_stc_sysint_t EZI2C_ISR_cfg = { 00055 .intrSrc = CYBSP_CSD_COMM_IRQ, 00056 .intrPriority = 3u 00057 }; 00058 00059 00060 /******************************************************************************* 00061 * Global variables 00062 *******************************************************************************/ 00063 //DigitalOut ledRed(LED_RED); 00064 Semaphore capsense_sem; 00065 EventQueue queue; 00066 cy_stc_scb_ezi2c_context_t EZI2C_context; 00067 uint32_t prevBtn0Status = 0u; 00068 uint32_t prevBtn1Status = 0u; 00069 uint32_t prevSliderPos = 0u; 00070 bool sw2Pressed = false; 00071 bool prevSw2Pressed = false; 00072 00073 00074 /* External global references */ 00075 //extern GUI_CONST_STORAGE GUI_BITMAP bmCypressLogo_1bpp; 00076 00077 extern GUI_CONST_STORAGE GUI_BITMAP bmExampleImage; 00078 extern GUI_CONST_STORAGE GUI_BITMAP bmCypressLogo; 00079 00080 // Callback routine is interrupt activated by a debounced pb1 hit 00081 void pb2_hit_callback (void) { 00082 // printf("Count is %d\n", ++countit); 00083 sw2Pressed = true; 00084 } 00085 // Callback routine is interrupt activated by a debounced pb1 hit 00086 void pb2_released_callback (void) { 00087 // printf("Count is %d\n", ++countit); 00088 sw2Pressed = false; 00089 } 00090 /******************************************************************************* 00091 * Function Name: bool IsBtnClicked 00092 ******************************************************************************** 00093 * 00094 * Summary: This non-blocking function implements SW2 button click check. 00095 * 00096 * Parameters: 00097 * None 00098 * 00099 * Return: 00100 * Status of the SW2 button: 00101 * true when button was pressed and then released and 00102 * false in other cases 00103 * 00104 *******************************************************************************/ 00105 bool IsBtnClicked(void) 00106 { 00107 int currBtnState; 00108 static int prevBtnState = BTN_RELEASED; 00109 00110 bool result = false; 00111 00112 currBtnState = sw2; 00113 00114 if((prevBtnState == BTN_RELEASED) && (currBtnState == BTN_PRESSED)) 00115 { 00116 result = true; 00117 } 00118 00119 prevBtnState = currBtnState; 00120 00121 wait_ms(5); 00122 00123 return result; 00124 } 00125 00126 /***************************************************************************** 00127 * Function Name: RunCapSenseScan() 00128 ****************************************************************************** 00129 * Summary: 00130 * This function starts the scan, and processes the touch status. It is 00131 * periodically called by an event dispatcher. 00132 * 00133 *****************************************************************************/ 00134 void RunCapSenseScan(void) 00135 { 00136 Cy_CapSense_ScanAllWidgets(&cy_capsense_context); 00137 capsense_sem.acquire(); 00138 Cy_CapSense_ProcessAllWidgets(&cy_capsense_context); 00139 Cy_CapSense_RunTuner(&cy_capsense_context); 00140 ProcessTouchStatus(); 00141 } 00142 00143 00144 /******************************************************************************* 00145 * Function Name: InitTunerCommunication 00146 ******************************************************************************** 00147 * 00148 * Summary: 00149 * This function performs the following functions: 00150 * - Initializes SCB block for operation in EZI2C mode 00151 * - Configures EZI2C pins 00152 * - Configures EZI2C clock 00153 * - Sets communication data buffer to CapSense data structure 00154 * 00155 *******************************************************************************/ 00156 void InitTunerCommunication(void) 00157 { 00158 /* Initialize EZI2C pins */ 00159 Cy_GPIO_Pin_Init(CYBSP_EZI2C_SCL_PORT, CYBSP_EZI2C_SCL_PIN, &CYBSP_EZI2C_SCL_config); 00160 Cy_GPIO_Pin_Init(CYBSP_EZI2C_SDA_PORT, CYBSP_EZI2C_SDA_PIN, &CYBSP_EZI2C_SDA_config); 00161 00162 /* Configure the peripheral clock for EZI2C */ 00163 Cy_SysClk_PeriphAssignDivider(PCLK_SCB3_CLOCK, CY_SYSCLK_DIV_8_BIT, 1U); 00164 Cy_SysClk_PeriphDisableDivider(CY_SYSCLK_DIV_8_BIT, 1U); 00165 Cy_SysClk_PeriphSetDivider(CY_SYSCLK_DIV_8_BIT, 1U, 7U); 00166 Cy_SysClk_PeriphEnableDivider(CY_SYSCLK_DIV_8_BIT, 1U); 00167 00168 Cy_SCB_EZI2C_Init(CYBSP_CSD_COMM_HW, &CYBSP_CSD_COMM_config, &EZI2C_context); 00169 00170 /* Initialize and enable EZI2C interrupts */ 00171 Cy_SysInt_Init(&EZI2C_ISR_cfg, &EZI2C_InterruptHandler); 00172 NVIC_EnableIRQ(EZI2C_ISR_cfg.intrSrc); 00173 00174 /* Set up communication data buffer to CapSense data structure to be exposed 00175 * to I2C master at primary slave address request. 00176 */ 00177 Cy_SCB_EZI2C_SetBuffer1(CYBSP_CSD_COMM_HW, (uint8 *)&cy_capsense_tuner, 00178 sizeof(cy_capsense_tuner), sizeof(cy_capsense_tuner), &EZI2C_context); 00179 00180 /* Enable EZI2C block */ 00181 Cy_SCB_EZI2C_Enable(CYBSP_CSD_COMM_HW); 00182 } 00183 00184 00185 /******************************************************************************* 00186 * Function Name: ProcessTouchStatus 00187 ******************************************************************************** 00188 * 00189 * Summary: 00190 * Controls the LED status according to the status of CapSense widgets and 00191 * prints the status to serial terminal. 00192 * 00193 *******************************************************************************/ 00194 void ProcessTouchStatus(void) 00195 { 00196 uint32_t currSliderPos; 00197 uint32_t currBtn0Status = Cy_CapSense_IsSensorActive(CY_CAPSENSE_BUTTON0_WDGT_ID, CY_CAPSENSE_BUTTON0_SNS0_ID, &cy_capsense_context); 00198 uint32_t currBtn1Status = Cy_CapSense_IsSensorActive(CY_CAPSENSE_BUTTON1_WDGT_ID, CY_CAPSENSE_BUTTON1_SNS0_ID, &cy_capsense_context); 00199 cy_stc_capsense_touch_t *sldrTouch = Cy_CapSense_GetTouchInfo(CY_CAPSENSE_LINEARSLIDER0_WDGT_ID, &cy_capsense_context); 00200 char outputString[80]; 00201 if(currBtn0Status != prevBtn0Status) 00202 { 00203 printf("Button_0 status: %u\r\n", currBtn0Status); 00204 sprintf(outputString," Button_0 status: %u ", currBtn0Status); 00205 GUI_SetTextAlign(GUI_TA_HCENTER); 00206 GUI_DispStringAt(outputString, 160, 60); 00207 prevBtn0Status = currBtn0Status; 00208 } 00209 00210 if(currBtn1Status != prevBtn1Status) 00211 { 00212 printf("Button_1 status: %u\r\n", currBtn1Status); 00213 sprintf(outputString," Button_1 status: %u ", currBtn1Status); 00214 GUI_SetTextAlign(GUI_TA_HCENTER); 00215 GUI_DispStringAt(outputString, 160, 80); 00216 prevBtn1Status = currBtn1Status; 00217 } 00218 if(sw2Pressed != prevSw2Pressed) { 00219 sprintf(outputString," Button_2 status: %u ", sw2Pressed); 00220 GUI_SetTextAlign(GUI_TA_HCENTER); 00221 GUI_DispStringAt(outputString, 160, 100); 00222 prevSw2Pressed = sw2Pressed; 00223 } 00224 if (sldrTouch->numPosition == SLIDER_NUM_TOUCH) 00225 { 00226 currSliderPos = sldrTouch->ptrPosition->x; 00227 00228 if(currSliderPos != prevSliderPos) 00229 { 00230 printf("Slider position: %u\r\n", currSliderPos); 00231 sprintf(outputString," Slider position: %u ", currSliderPos); 00232 GUI_SetTextAlign(GUI_TA_HCENTER); 00233 GUI_DispStringAt(outputString, 160, 120); 00234 prevSliderPos = currSliderPos; 00235 } 00236 } 00237 00238 ledRed = (sw2Pressed || currBtn0Status || currBtn1Status || (sldrTouch->numPosition == SLIDER_NUM_TOUCH)) ? LED_ON : LED_OFF; 00239 } 00240 00241 00242 /******************************************************************************* 00243 * Function Name: EZI2C_InterruptHandler 00244 ******************************************************************************** 00245 * Summary: 00246 * Wrapper function for handling interrupts from EZI2C block. 00247 * 00248 *******************************************************************************/ 00249 void EZI2C_InterruptHandler(void) 00250 { 00251 Cy_SCB_EZI2C_Interrupt(CYBSP_CSD_COMM_HW, &EZI2C_context); 00252 } 00253 00254 /***************************************************************************** 00255 * Function Name: CapSense_InterruptHandler() 00256 ****************************************************************************** 00257 * Summary: 00258 * Wrapper function for handling interrupts from CSD block. 00259 * 00260 *****************************************************************************/ 00261 void CapSense_InterruptHandler(void) 00262 { 00263 Cy_CapSense_InterruptHandler(CYBSP_CSD_HW, &cy_capsense_context); 00264 } 00265 00266 00267 /***************************************************************************** 00268 * Function Name: CapSenseEndOfScanCallback() 00269 ****************************************************************************** 00270 * Summary: 00271 * This function releases a semaphore to indicate end of a CapSense scan. 00272 * 00273 * Parameters: 00274 * cy_stc_active_scan_sns_t* : pointer to active sensor details. 00275 * 00276 *****************************************************************************/ 00277 void CapSenseEndOfScanCallback(cy_stc_active_scan_sns_t * ptrActiveScan) 00278 { 00279 capsense_sem.release(); 00280 } 00281 00282 00283 /***************************************************************************** 00284 * Function Name: InitCapSenseClock() 00285 ****************************************************************************** 00286 * Summary: 00287 * This function configures the peripheral clock for CapSense. 00288 * 00289 *****************************************************************************/ 00290 void InitCapSenseClock(void) 00291 { 00292 Cy_SysClk_PeriphAssignDivider(PCLK_CSD_CLOCK, CYBSP_CSD_CLK_DIV_HW, CYBSP_CSD_CLK_DIV_NUM); 00293 Cy_SysClk_PeriphDisableDivider(CYBSP_CSD_CLK_DIV_HW, CYBSP_CSD_CLK_DIV_NUM); 00294 Cy_SysClk_PeriphSetDivider(CYBSP_CSD_CLK_DIV_HW, CYBSP_CSD_CLK_DIV_NUM, 0u); 00295 Cy_SysClk_PeriphEnableDivider(CYBSP_CSD_CLK_DIV_HW, CYBSP_CSD_CLK_DIV_NUM); 00296 } 00297 00298 /***************************************************************************** 00299 * Function Name: main() 00300 ****************************************************************************** 00301 * Summary: 00302 * Main function that starts a thread for CapSense scan and enters a forever 00303 * wait state. 00304 * 00305 *****************************************************************************/ 00306 int main(void) 00307 { 00308 /* Turn off both red and green LEDs */ 00309 ledGreen = LED_OFF; 00310 ledRed = LED_OFF; 00311 ledBlue = LED_OFF; 00312 ledGreen2 = 0; /* 1 for on 0 for off */ 00313 ledRed2 = 0; 00314 ledBlue2 = 0; 00315 char outputString[80]; 00316 00317 /* Initialize EmWin driver*/ 00318 GUI_Init(); 00319 /* Set font size, foreground and background Colours */ 00320 GUI_SetFont(GUI_FONT_16B_1); 00321 GUI_SetColor(GUI_WHITE); 00322 GUI_SetBkColor(GUI_BLACK); 00323 00324 GUI_Clear(); 00325 GUI_SetTextAlign(GUI_TA_HCENTER); 00326 GUI_DispStringAt("Capsense Demo", 160, 20); 00327 printf("\r\nApplication has started. Touch any CapSense button or slider.\r\n"); 00328 sprintf(outputString,"\r\nApplication has started.\r\n Touch any CapSense button or slider."); 00329 GUI_SetTextAlign(GUI_TA_HCENTER); 00330 GUI_DispStringAt(outputString, 160, 180); 00331 wait(0.10); 00332 /* Configure AMUX bus for CapSense */ 00333 init_cycfg_routing(); 00334 00335 /* Configure PERI clocks for CapSense */ 00336 InitCapSenseClock(); 00337 00338 InitTunerCommunication(); 00339 00340 /* Initialize the CSD HW block to the default state. */ 00341 cy_status status = Cy_CapSense_Init(&cy_capsense_context); 00342 if(CY_RET_SUCCESS != status) 00343 { 00344 printf("CapSense initialization failed. Status code: %u\r\n", status); 00345 sprintf(outputString,"CapSense initialization failed. Status code: %u", status); 00346 GUI_SetTextAlign(GUI_TA_HCENTER); 00347 GUI_DispStringAt(outputString, 160, 40); 00348 wait(osWaitForever); 00349 } 00350 00351 /* Initialize CapSense interrupt */ 00352 Cy_SysInt_Init(&CapSense_ISR_cfg, &CapSense_InterruptHandler); 00353 NVIC_ClearPendingIRQ(CapSense_ISR_cfg.intrSrc); 00354 NVIC_EnableIRQ(CapSense_ISR_cfg.intrSrc); 00355 00356 /* Initialize the CapSense firmware modules. */ 00357 Cy_CapSense_Enable(&cy_capsense_context); 00358 Cy_CapSense_RegisterCallback(CY_CAPSENSE_END_OF_SCAN_E, CapSenseEndOfScanCallback, &cy_capsense_context); 00359 00360 /* Create a thread to run CapSense scan periodically using an event queue 00361 * dispatcher. 00362 */ 00363 Thread thread(osPriorityNormal, OS_STACK_SIZE, NULL, "CapSense Scan Thread"); 00364 thread.start(callback(&queue, &EventQueue::dispatch_forever)); 00365 queue.call_every(CAPSENSE_SCAN_PERIOD_MS, RunCapSenseScan); 00366 00367 /* Initiate scan immediately since the first call of RunCapSenseScan() 00368 * happens CAPSENSE_SCAN_PERIOD_MS after the event queue dispatcher has 00369 * started. 00370 */ 00371 Cy_CapSense_ScanAllWidgets(&cy_capsense_context); 00372 00373 00374 // Use internal pullups for pushbutton 00375 pb2.mode(PullUp); 00376 // pb2.mode(PullUp); 00377 // Delay for initial pullup to take effect 00378 wait(.01); 00379 // Setup Interrupt callback functions for a pb hit 00380 pb2.attach_deasserted(&pb2_hit_callback); 00381 // pb2.attach_deasserted(&pb2_hit_callback); 00382 // Start sampling pb inputs using interrupts 00383 pb2.setSampleFrequency(); 00384 00385 pb2.attach_asserted( &pb2_released_callback ); 00386 wait(osWaitForever); 00387 00388 } 00389
Generated on Thu Jul 28 2022 12:57:44 by
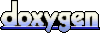