printer
Dependents: Good_Serial_HelloWorld_Mbed
Fork of mbed by
Timer.h
00001 /* mbed Microcontroller Library - Timer 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_TIMER_H 00006 #define MBED_TIMER_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 00013 namespace mbed { 00014 00015 /* Class: Timer 00016 * A general purpose timer 00017 * 00018 * Example: 00019 * > // Count the time to toggle a LED 00020 * > 00021 * > #include "mbed.h" 00022 * > 00023 * > Timer timer; 00024 * > DigitalOut led(LED1); 00025 * > int begin, end; 00026 * > 00027 * > int main() { 00028 * > timer.start(); 00029 * > begin = timer.read_us(); 00030 * > led = !led; 00031 * > end = timer.read_us(); 00032 * > printf("Toggle the led takes %d us", end - begin); 00033 * > } 00034 */ 00035 class Timer : public Base { 00036 00037 public: 00038 00039 Timer(const char *name = NULL); 00040 00041 /* Function: start 00042 * Start the timer 00043 */ 00044 void start(); 00045 00046 /* Function: stop 00047 * Stop the timer 00048 */ 00049 void stop(); 00050 00051 /* Function: reset 00052 * Reset the timer to 0. 00053 * 00054 * If it was already counting, it will continue 00055 */ 00056 void reset(); 00057 00058 /* Function: read 00059 * Get the time passed in seconds 00060 */ 00061 float read(); 00062 00063 /* Function: read_ms 00064 * Get the time passed in mili-seconds 00065 */ 00066 int read_ms(); 00067 00068 /* Function: read_us 00069 * Get the time passed in micro-seconds 00070 */ 00071 int read_us(); 00072 00073 #ifdef MBED_OPERATORS 00074 operator float(); 00075 #endif 00076 00077 #ifdef MBED_RPC 00078 virtual const struct rpc_method *get_rpc_methods(); 00079 static struct rpc_class *get_rpc_class(); 00080 #endif 00081 00082 protected: 00083 00084 int slicetime(); 00085 int _running; // whether the timer is running 00086 unsigned int _start; // the start time of the latest slice 00087 int _time; // any accumulated time from previous slices 00088 00089 }; 00090 00091 } // namespace mbed 00092 00093 #endif
Generated on Wed Jul 13 2022 17:44:11 by
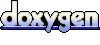