printer
Dependents: Good_Serial_HelloWorld_Mbed
Fork of mbed by
SPI.h
00001 /* mbed Microcontroller Library - SPI 00002 * Copyright (c) 2010-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_SPI_H 00006 #define MBED_SPI_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_SPI 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /* Class: SPI 00020 * A SPI Master, used for communicating with SPI slave devices 00021 * 00022 * The default format is set to 8-bits, mode 0, and a clock frequency of 1MHz 00023 * 00024 * Most SPI devices will also require Chip Select and Reset signals. These 00025 * can be controlled using <DigitalOut> pins 00026 * 00027 * Example: 00028 * > // Send a byte to a SPI slave, and record the response 00029 * > 00030 * > #include "mbed.h" 00031 * > 00032 * > SPI device(p5, p6, p7); // mosi, miso, sclk 00033 * > 00034 * > int main() { 00035 * > int response = device.write(0xFF); 00036 * > } 00037 */ 00038 class SPI : public Base { 00039 00040 public: 00041 00042 /* Constructor: SPI 00043 * Create a SPI master connected to the specified pins 00044 * 00045 * Variables: 00046 * mosi - SPI Master Out, Slave In pin 00047 * miso - SPI Master In, Slave Out pin 00048 * sclk - SPI Clock pin 00049 * name - (optional) A string to identify the object 00050 * 00051 * Pin Options: 00052 * (5, 6, 7) or (11, 12, 13) 00053 * 00054 * mosi or miso can be specfied as NC if not used 00055 */ 00056 SPI(PinName mosi, PinName miso, PinName sclk, const char *name = NULL); 00057 00058 /* Function: format 00059 * Configure the data transmission format 00060 * 00061 * Variables: 00062 * bits - Number of bits per SPI frame (4 - 16) 00063 * mode - Clock polarity and phase mode (0 - 3) 00064 * 00065 * > mode | POL PHA 00066 * > -----+-------- 00067 * > 0 | 0 0 00068 * > 1 | 0 1 00069 * > 2 | 1 0 00070 * > 3 | 1 1 00071 */ 00072 void format(int bits, int mode = 0); 00073 00074 /* Function: frequency 00075 * Set the spi bus clock frequency 00076 * 00077 * Variables: 00078 * hz - SCLK frequency in hz (default = 1MHz) 00079 */ 00080 void frequency(int hz = 1000000); 00081 00082 /* Function: write 00083 * Write to the SPI Slave and return the response 00084 * 00085 * Variables: 00086 * value - Data to be sent to the SPI slave 00087 * returns - Response from the SPI slave 00088 */ 00089 virtual int write(int value); 00090 00091 00092 #ifdef MBED_RPC 00093 virtual const struct rpc_method *get_rpc_methods(); 00094 static struct rpc_class *get_rpc_class(); 00095 #endif 00096 00097 protected: 00098 00099 SPIName _spi; 00100 00101 void aquire(void); 00102 static SPI *_owner; 00103 int _bits; 00104 int _mode; 00105 int _hz; 00106 00107 }; 00108 00109 } // namespace mbed 00110 00111 #endif 00112 00113 #endif
Generated on Wed Jul 13 2022 17:44:10 by
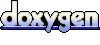