printer
Dependents: Good_Serial_HelloWorld_Mbed
Fork of mbed by
PortIn.h
00001 /* mbed Microcontroller Library - PortInOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_PORTIN_H 00006 #define MBED_PORTIN_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_PORTIN 00011 00012 #include "PortNames.h" 00013 #include "PinNames.h" 00014 00015 namespace mbed { 00016 00017 /* Class: PortIn 00018 * A multiple pin digital input 00019 * 00020 * Example: 00021 * > // Switch on an LED if any of mbed pins 21-26 is high 00022 * > 00023 * > #include "mbed.h" 00024 * > 00025 * > PortIn p(Port2, 0x0000003F); // p21-p26 00026 * > DigitalOut ind(LED4); 00027 * > 00028 * > int main() { 00029 * > while(1) { 00030 * > int pins = p.read(); 00031 * > if(pins) { 00032 * > ind = 1; 00033 * > } else { 00034 * > ind = 0; 00035 * > } 00036 * > } 00037 * > } 00038 */ 00039 class PortIn { 00040 public: 00041 00042 /* Constructor: PortIn 00043 * Create an PortIn, connected to the specified port 00044 * 00045 * Variables: 00046 * port - Port to connect to (Port0-Port5) 00047 * mask - A bitmask to identify which bits in the port should be included (0 - ignore) 00048 */ 00049 PortIn(PortName port, int mask = 0xFFFFFFFF); 00050 00051 /* Function: read 00052 * Read the value currently output on the port 00053 * 00054 * Variables: 00055 * returns - An integer with each bit corresponding to associated port pin setting 00056 */ 00057 int read(); 00058 00059 /* Function: mode 00060 * Set the input pin mode 00061 * 00062 * Variables: 00063 * mode - PullUp, PullDown, PullNone, OpenDrain 00064 */ 00065 void mode(PinMode mode); 00066 00067 /* Function: operator int() 00068 * A shorthand for <read> 00069 */ 00070 operator int() { 00071 return read(); 00072 } 00073 00074 private: 00075 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00076 LPC_GPIO_TypeDef *_gpio; 00077 #endif 00078 PortName _port; 00079 uint32_t _mask; 00080 }; 00081 00082 } // namespace mbed 00083 00084 #endif 00085 00086 #endif
Generated on Wed Jul 13 2022 17:44:10 by
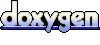