printer
Dependents: Good_Serial_HelloWorld_Mbed
Fork of mbed by
PortInOut.h
00001 /* mbed Microcontroller Library - PortInOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_PORTINOUT_H 00006 #define MBED_PORTINOUT_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_PORTINOUT 00011 00012 #include "PortNames.h" 00013 #include "PinNames.h" 00014 00015 namespace mbed { 00016 00017 /* Class: PortInOut 00018 * A multiple pin digital in/out used to set/read multiple bi-directional pins 00019 */ 00020 class PortInOut { 00021 public: 00022 00023 /* Constructor: PortInOut 00024 * Create an PortInOut, connected to the specified port 00025 * 00026 * Variables: 00027 * port - Port to connect to (Port0-Port5) 00028 * mask - A bitmask to identify which bits in the port should be included (0 - ignore) 00029 */ 00030 PortInOut(PortName port, int mask = 0xFFFFFFFF); 00031 00032 /* Function: write 00033 * Write the value to the output port 00034 * 00035 * Variables: 00036 * value - An integer specifying a bit to write for every corresponding port pin 00037 */ 00038 void write(int value); 00039 00040 /* Function: read 00041 * Read the value currently output on the port 00042 * 00043 * Variables: 00044 * returns - An integer with each bit corresponding to associated port pin setting 00045 */ 00046 int read(); 00047 00048 /* Function: output 00049 * Set as an output 00050 */ 00051 void output(); 00052 00053 /* Function: input 00054 * Set as an input 00055 */ 00056 void input(); 00057 00058 /* Function: mode 00059 * Set the input pin mode 00060 * 00061 * Variables: 00062 * mode - PullUp, PullDown, PullNone, OpenDrain 00063 */ 00064 void mode(PinMode mode); 00065 00066 /* Function: operator= 00067 * A shorthand for <write> 00068 */ 00069 PortInOut& operator= (int value) { 00070 write(value); 00071 return *this; 00072 } 00073 00074 PortInOut& operator= (PortInOut& rhs) { 00075 write(rhs.read()); 00076 return *this; 00077 } 00078 00079 /* Function: operator int() 00080 * A shorthand for <read> 00081 */ 00082 operator int() { 00083 return read(); 00084 } 00085 00086 private: 00087 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00088 LPC_GPIO_TypeDef *_gpio; 00089 #endif 00090 PortName _port; 00091 uint32_t _mask; 00092 }; 00093 00094 } // namespace mbed 00095 00096 #endif 00097 00098 #endif
Generated on Wed Jul 13 2022 17:44:10 by
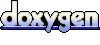