printer
Dependents: Good_Serial_HelloWorld_Mbed
Fork of mbed by
BusOut.h
00001 /* mbed Microcontroller Library - BusOut 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_BUSOUT_H 00006 #define MBED_BUSOUT_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 #include "DigitalOut.h" 00013 00014 namespace mbed { 00015 00016 /* Class: BusOut 00017 * A digital output bus, used for setting the state of a collection of pins 00018 */ 00019 class BusOut : public Base { 00020 00021 public: 00022 00023 /* Group: Configuration Methods */ 00024 00025 /* Constructor: BusOut 00026 * Create an BusOut, connected to the specified pins 00027 * 00028 * Variables: 00029 * p<n> - DigitalOut pin to connect to bus bit <n> (p5-p30, NC) 00030 * 00031 * Note: 00032 * It is only required to specify as many pin variables as is required 00033 * for the bus; the rest will default to NC (not connected) 00034 */ 00035 BusOut(PinName p0, PinName p1 = NC, PinName p2 = NC, PinName p3 = NC, 00036 PinName p4 = NC, PinName p5 = NC, PinName p6 = NC, PinName p7 = NC, 00037 PinName p8 = NC, PinName p9 = NC, PinName p10 = NC, PinName p11 = NC, 00038 PinName p12 = NC, PinName p13 = NC, PinName p14 = NC, PinName p15 = NC, 00039 const char *name = NULL); 00040 00041 BusOut(PinName pins[16], const char *name = NULL); 00042 00043 virtual ~BusOut(); 00044 00045 /* Group: Access Methods */ 00046 00047 /* Function: write 00048 * Write the value to the output bus 00049 * 00050 * Variables: 00051 * value - An integer specifying a bit to write for every corresponding DigitalOut pin 00052 */ 00053 void write(int value); 00054 00055 00056 /* Function: read 00057 * Read the value currently output on the bus 00058 * 00059 * Variables: 00060 * returns - An integer with each bit corresponding to associated DigitalOut pin setting 00061 */ 00062 int read(); 00063 00064 #ifdef MBED_OPERATORS 00065 /* Group: Access Method Shorthand */ 00066 00067 /* Function: operator= 00068 * A shorthand for <write> 00069 */ 00070 BusOut& operator= (int v); 00071 BusOut& operator= (BusOut& rhs); 00072 00073 /* Function: operator int() 00074 * A shorthand for <read> 00075 */ 00076 operator int(); 00077 #endif 00078 00079 #ifdef MBED_RPC 00080 virtual const struct rpc_method *get_rpc_methods(); 00081 static struct rpc_class *get_rpc_class(); 00082 #endif 00083 00084 protected: 00085 00086 DigitalOut* _pin[16]; 00087 00088 #ifdef MBED_RPC 00089 static void construct(const char *arguments, char *res); 00090 #endif 00091 00092 }; 00093 00094 } // namespace mbed 00095 00096 #endif 00097
Generated on Wed Jul 13 2022 17:44:10 by
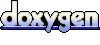