printer
Dependents: Good_Serial_HelloWorld_Mbed
Fork of mbed by
AnalogOut.h
00001 /* mbed Microcontroller Library - AnalogOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_ANALOGOUT_H 00006 #define MBED_ANALOGOUT_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_ANALOGOUT 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /* Class: AnalogOut 00020 * An analog output, used for setting the voltage on a pin 00021 * 00022 * Example: 00023 * > // Make a sawtooth output 00024 * > 00025 * > #include "mbed.h" 00026 * > 00027 * > AnalogOut tri(p18); 00028 * > int main() { 00029 * > while(1) { 00030 * > tri = tri + 0.01; 00031 * > wait_us(1); 00032 * > if(tri == 1) { 00033 * > tri = 0; 00034 * > } 00035 * > } 00036 * > } 00037 */ 00038 class AnalogOut : public Base { 00039 00040 public: 00041 00042 /* Constructor: AnalogOut 00043 * Create an AnalogOut connected to the specified pin 00044 * 00045 * Variables: 00046 * pin - AnalogOut pin to connect to (18) 00047 */ 00048 AnalogOut(PinName pin, const char *name = NULL); 00049 00050 /* Function: write 00051 * Set the output voltage, specified as a percentage (float) 00052 * 00053 * Variables: 00054 * percent - A floating-point value representing the output voltage, 00055 * specified as a percentage. The value should lie between 00056 * 0.0f (representing 0v / 0%) and 1.0f (representing 3.3v / 100%). 00057 * Values outside this range will be saturated to 0.0f or 1.0f. 00058 */ 00059 void write(float value); 00060 00061 /* Function: write_u16 00062 * Set the output voltage, represented as an unsigned short in the range [0x0, 0xFFFF] 00063 * 00064 * Variables: 00065 * value - 16-bit unsigned short representing the output voltage, 00066 * normalised to a 16-bit value (0x0000 = 0v, 0xFFFF = 3.3v) 00067 */ 00068 void write_u16(unsigned short value); 00069 00070 /* Function: read 00071 * Return the current output voltage setting, measured as a percentage (float) 00072 * 00073 * Variables: 00074 * returns - A floating-point value representing the current voltage being output on the pin, 00075 * measured as a percentage. The returned value will lie between 00076 * 0.0f (representing 0v / 0%) and 1.0f (representing 3.3v / 100%). 00077 * 00078 * Note: 00079 * This value may not match exactly the value set by a previous <write>. 00080 */ 00081 float read(); 00082 00083 00084 #ifdef MBED_OPERATORS 00085 /* Function: operator= 00086 * An operator shorthand for <write()> 00087 */ 00088 AnalogOut& operator= (float percent); 00089 AnalogOut& operator= (AnalogOut& rhs); 00090 00091 /* Function: operator float() 00092 * An operator shorthand for <read()> 00093 */ 00094 operator float(); 00095 #endif 00096 00097 #ifdef MBED_RPC 00098 virtual const struct rpc_method *get_rpc_methods(); 00099 static struct rpc_class *get_rpc_class(); 00100 #endif 00101 00102 protected: 00103 00104 DACName _dac; 00105 00106 }; 00107 00108 } // namespace mbed 00109 00110 #endif 00111 00112 #endif
Generated on Wed Jul 13 2022 17:44:10 by
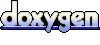