Extended MaximInterface
Dependents: mbed_DS28EC20_GPIO
I2CMaster Class Reference
I2C master interface. More...
#include <I2CMaster.hpp>
Inherited by DS9400, I2CMasterDecorator, and I2CMaster.
Public Types | |
enum | ErrorValue { NackError = 1 } |
Public Member Functions | |
virtual error_code | start (uint_least8_t address)=0 |
Send start condition and address on the bus. | |
virtual error_code | stop ()=0 |
Send stop condition on the bus. | |
virtual error_code | writeByte (uint_least8_t data)=0 |
Write data byte to the bus. | |
virtual MaximInterface_EXPORT error_code | writeBlock (span< const uint_least8_t > data) |
Write data block to the bus. | |
error_code | writePacket (uint_least8_t address, span< const uint_least8_t > data, bool sendStop=true) |
Perform a complete write transaction on the bus with optional stop condition. | |
virtual error_code | readByte (AckStatus status, uint_least8_t &data)=0 |
Read data byte from the bus. | |
virtual MaximInterface_EXPORT error_code | readBlock (AckStatus status, span< uint_least8_t > data) |
Read data block from the bus. | |
error_code | readPacket (uint_least8_t address, span< uint_least8_t > data, bool sendStop=true) |
Perform a complete read transaction on the bus with optional stop condition. |
Detailed Description
I2C master interface.
Definition at line 44 of file Links/I2CMaster.hpp.
Member Enumeration Documentation
enum ErrorValue |
Definition at line 46 of file Links/I2CMaster.hpp.
Member Function Documentation
error_code readBlock | ( | AckStatus | status, |
span< uint_least8_t > | data | ||
) | [virtual] |
Read data block from the bus.
- Parameters:
-
status Determines whether an ACK or NACK is sent after reading. [out] data Data read from the bus if successful.
Definition at line 63 of file Links/I2CMaster.cpp.
virtual error_code readByte | ( | AckStatus | status, |
uint_least8_t & | data | ||
) | [pure virtual] |
Read data byte from the bus.
- Parameters:
-
status Determines whether an ACK or NACK is sent after reading. [out] data Data read from the bus if successful.
Implemented in I2CMaster.
error_code readPacket | ( | uint_least8_t | address, |
span< uint_least8_t > | data, | ||
bool | sendStop = true |
||
) |
Perform a complete read transaction on the bus with optional stop condition.
- Parameters:
-
address Address in 8-bit format. [out] data Data read from the bus if successful. sendStop True to send a stop condition or false to set up a repeated start.
Definition at line 98 of file Links/I2CMaster.hpp.
virtual error_code start | ( | uint_least8_t | address ) | [pure virtual] |
Send start condition and address on the bus.
- Parameters:
-
address Address with R/W bit.
Implemented in I2CMaster.
virtual error_code stop | ( | ) | [pure virtual] |
Send stop condition on the bus.
Implemented in I2CMaster.
error_code writeBlock | ( | span< const uint_least8_t > | data ) | [virtual] |
Write data block to the bus.
Definition at line 38 of file Links/I2CMaster.cpp.
virtual error_code writeByte | ( | uint_least8_t | data ) | [pure virtual] |
Write data byte to the bus.
Implemented in I2CMaster.
error_code writePacket | ( | uint_least8_t | address, |
span< const uint_least8_t > | data, | ||
bool | sendStop = true |
||
) |
Perform a complete write transaction on the bus with optional stop condition.
- Parameters:
-
address Address in 8-bit format. data Data to write to the bus. sendStop True to send a stop condition or false to set up a repeated start.
Definition at line 75 of file Links/I2CMaster.hpp.
Generated on Tue Jul 12 2022 23:29:45 by
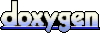