
STM32F7 Ethernet interface for nucleo STM32F767
DNS - host name to IP address resolver. More...
Go to the source code of this file.
Functions | |
static void | dns_recv (void *arg, struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *addr, u16_t port) |
Receive input function for DNS response packets arriving for the dns UDP pcb. | |
static void | dns_check_entries (void) |
Call dns_check_entry for each entry in dns_table - check all entries. | |
static void | dns_call_found (u8_t idx, ip_addr_t *addr) |
dns_call_found() - call the found callback and check if there are duplicate entries for the given hostname. | |
void | dns_init (void) |
Initialize the resolver: set up the UDP pcb and configure the default server (if DNS_SERVER_ADDRESS is set). | |
void | dns_setserver (u8_t numdns, const ip_addr_t *dnsserver) |
Initialize one of the DNS servers. | |
const ip_addr_t * | dns_getserver (u8_t numdns) |
Obtain one of the currently configured DNS server. | |
void | dns_tmr (void) |
The DNS resolver client timer - handle retries and timeouts and should be called every DNS_TMR_INTERVAL milliseconds (every second by default). | |
size_t | dns_local_iterate (dns_found_callback iterator_fn, void *iterator_arg) |
Iterate the local host-list for a hostname. | |
err_t | dns_local_lookup (const char *hostname, ip_addr_t *addr, u8_t dns_addrtype) |
Scans the local host-list for a hostname. | |
int | dns_local_removehost (const char *hostname, const ip_addr_t *addr) |
Remove all entries from the local host-list for a specific hostname and/or IP address. | |
err_t | dns_local_addhost (const char *hostname, const ip_addr_t *addr) |
Add a hostname/IP address pair to the local host-list. | |
static err_t | dns_lookup (const char *name, ip_addr_t *addr LWIP_DNS_ADDRTYPE_ARG(u8_t dns_addrtype)) |
Look up a hostname in the array of known hostnames. | |
static u16_t | dns_compare_name (const char *query, struct pbuf *p, u16_t start_offset) |
Compare the "dotted" name "query" with the encoded name "response" to make sure an answer from the DNS server matches the current dns_table entry (otherwise, answers might arrive late for hostname not on the list any more). | |
static u16_t | dns_skip_name (struct pbuf *p, u16_t query_idx) |
Walk through a compact encoded DNS name and return the end of the name. | |
static err_t | dns_send (u8_t idx) |
Send a DNS query packet. | |
static u8_t | dns_alloc_pcb (void) |
dns_alloc_pcb() - allocates a new pcb (or reuses an existing one) to be used for sending a request | |
static void | dns_check_entry (u8_t i) |
dns_check_entry() - see if entry has not yet been queried and, if so, sends out a query. | |
static void | dns_correct_response (u8_t idx, u32_t ttl) |
Save TTL and call dns_call_found for correct response. | |
static err_t | dns_enqueue (const char *name, size_t hostnamelen, dns_found_callback found, void *callback_arg LWIP_DNS_ADDRTYPE_ARG(u8_t dns_addrtype) LWIP_DNS_ISMDNS_ARG(u8_t is_mdns)) |
Queues a new hostname to resolve and sends out a DNS query for that hostname. | |
err_t | dns_gethostbyname (const char *hostname, ip_addr_t *addr, dns_found_callback found, void *callback_arg) |
Resolve a hostname (string) into an IP address. | |
err_t | dns_gethostbyname_addrtype (const char *hostname, ip_addr_t *addr, dns_found_callback found, void *callback_arg, u8_t dns_addrtype) |
Like dns_gethostbyname, but returned address type can be controlled: | |
Variables | |
static struct local_hostlist_entry * | local_hostlist_dynamic |
Local host-list. |
Detailed Description
DNS - host name to IP address resolver.
Definition in file lwip_dns.c.
Function Documentation
static u8_t dns_alloc_pcb | ( | void | ) | [static] |
dns_alloc_pcb() - allocates a new pcb (or reuses an existing one) to be used for sending a request
- Returns:
- an index into dns_pcbs
Definition at line 881 of file lwip_dns.c.
static void dns_call_found | ( | u8_t | idx, |
ip_addr_t * | addr | ||
) | [static] |
dns_call_found() - call the found callback and check if there are duplicate entries for the given hostname.
If there are any, their found callback will be called and they will be removed.
- Parameters:
-
idx dns table index of the entry that is resolved or removed addr IP address for the hostname (or NULL on error or memory shortage)
Definition at line 923 of file lwip_dns.c.
static void dns_check_entries | ( | void | ) | [static] |
Call dns_check_entry for each entry in dns_table - check all entries.
Definition at line 1088 of file lwip_dns.c.
static void dns_check_entry | ( | u8_t | i ) | [static] |
dns_check_entry() - see if entry has not yet been queried and, if so, sends out a query.
Check an entry in the dns_table:
- send out query for new entries
- retry old pending entries on timeout (also with different servers)
- remove completed entries from the table if their TTL has expired
- Parameters:
-
i index of the dns_table entry to check
Definition at line 1011 of file lwip_dns.c.
static u16_t dns_compare_name | ( | const char * | query, |
struct pbuf * | p, | ||
u16_t | start_offset | ||
) | [static] |
Compare the "dotted" name "query" with the encoded name "response" to make sure an answer from the DNS server matches the current dns_table entry (otherwise, answers might arrive late for hostname not on the list any more).
- Parameters:
-
query hostname (not encoded) from the dns_table p pbuf containing the encoded hostname in the DNS response start_offset offset into p where the name starts
- Returns:
- 0xFFFF: names differ, other: names equal -> offset behind name
- See also:
- RFC 1035 - 4.1.4. Message compression
Definition at line 651 of file lwip_dns.c.
static void dns_correct_response | ( | u8_t | idx, |
u32_t | ttl | ||
) | [static] |
Save TTL and call dns_call_found for correct response.
Definition at line 1101 of file lwip_dns.c.
static err_t dns_enqueue | ( | const char * | name, |
size_t | hostnamelen, | ||
dns_found_callback | found, | ||
void *callback_arg | LWIP_DNS_ADDRTYPE_ARGu8_t dns_addrtype) LWIP_DNS_ISMDNS_ARG(u8_t is_mdns | ||
) | [static] |
Queues a new hostname to resolve and sends out a DNS query for that hostname.
- Parameters:
-
name the hostname that is to be queried hostnamelen length of the hostname found a callback function to be called on success, failure or timeout callback_arg argument to pass to the callback function
- Returns:
- err_t return code.
Definition at line 1317 of file lwip_dns.c.
void dns_init | ( | void | ) |
Initialize the resolver: set up the UDP pcb and configure the default server (if DNS_SERVER_ADDRESS is set).
Definition at line 318 of file lwip_dns.c.
static void dns_recv | ( | void * | s, |
struct udp_pcb * | pcb, | ||
struct pbuf * | p, | ||
const ip_addr_t * | addr, | ||
u16_t | port | ||
) | [static] |
Receive input function for DNS response packets arriving for the dns UDP pcb.
Definition at line 1133 of file lwip_dns.c.
static err_t dns_send | ( | u8_t | idx ) | [static] |
Send a DNS query packet.
- Parameters:
-
idx the DNS table entry index for which to send a request
- Returns:
- ERR_OK if packet is sent; an err_t indicating the problem otherwise
Definition at line 735 of file lwip_dns.c.
static u16_t dns_skip_name | ( | struct pbuf * | p, |
u16_t | query_idx | ||
) | [static] |
Walk through a compact encoded DNS name and return the end of the name.
- Parameters:
-
p pbuf containing the name query_idx start index into p pointing to encoded DNS name in the DNS server response
- Returns:
- index to end of the name
- See also:
- RFC 1035 - 4.1.4. Message compression
Definition at line 698 of file lwip_dns.c.
void dns_tmr | ( | void | ) |
The DNS resolver client timer - handle retries and timeouts and should be called every DNS_TMR_INTERVAL milliseconds (every second by default).
Definition at line 398 of file lwip_dns.c.
Variable Documentation
struct local_hostlist_entry* local_hostlist_dynamic [static] |
Local host-list.
For hostnames in this list, no external name resolution is performed
Definition at line 264 of file lwip_dns.c.
Generated on Tue Jul 12 2022 14:49:27 by
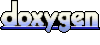