
STM32F7 Ethernet interface for nucleo STM32F767
UDP
User Datagram Protocol module
.
More...
Functions | |
err_t | udp_send (struct udp_pcb *pcb, struct pbuf *p) |
Send data using UDP. | |
err_t | udp_send_chksum (struct udp_pcb *pcb, struct pbuf *p, u8_t have_chksum, u16_t chksum) |
Same as udp_send() but with checksum. | |
err_t | udp_sendto (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port) |
Send data to a specified address using UDP. | |
err_t | udp_sendto_chksum (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, u8_t have_chksum, u16_t chksum) |
Same as udp_sendto(), but with checksum. | |
err_t | udp_sendto_if (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif) |
Send data to a specified address using UDP. | |
err_t | udp_sendto_if_src (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif, const ip_addr_t *src_ip) |
Same as udp_sendto_if, but with source address. | |
err_t | udp_bind (struct udp_pcb *pcb, const ip_addr_t *ipaddr, u16_t port) |
Bind an UDP PCB. | |
err_t | udp_connect (struct udp_pcb *pcb, const ip_addr_t *ipaddr, u16_t port) |
Connect an UDP PCB. | |
void | udp_disconnect (struct udp_pcb *pcb) |
Disconnect a UDP PCB. | |
void | udp_recv (struct udp_pcb *pcb, udp_recv_fn recv, void *recv_arg) |
Set a receive callback for a UDP PCB. | |
void | udp_remove (struct udp_pcb *pcb) |
Remove an UDP PCB. | |
struct udp_pcb * | udp_new (void) |
Create a UDP PCB. | |
struct udp_pcb * | udp_new_ip_type (u8_t type) |
Create a UDP PCB for specific IP type. |
Detailed Description
User Datagram Protocol module
.
- See also:
- raw_api and Netconn API
Function Documentation
Bind an UDP PCB.
- Parameters:
-
pcb UDP PCB to be bound with a local address ipaddr and port. ipaddr local IP address to bind with. Use IP4_ADDR_ANY to bind to all local interfaces. port local UDP port to bind with. Use 0 to automatically bind to a random port between UDP_LOCAL_PORT_RANGE_START and UDP_LOCAL_PORT_RANGE_END.
ipaddr & port are expected to be in the same byte order as in the pcb.
- Returns:
- lwIP error code.
- ERR_OK. Successful. No error occurred.
- ERR_USE. The specified ipaddr and port are already bound to by another UDP PCB.
- See also:
- udp_disconnect()
Definition at line 882 of file lwip_udp.c.
Connect an UDP PCB.
This will associate the UDP PCB with the remote address.
- Parameters:
-
pcb UDP PCB to be connected with remote address ipaddr and port. ipaddr remote IP address to connect with. port remote UDP port to connect with.
- Returns:
- lwIP error code
ipaddr & port are expected to be in the same byte order as in the pcb.
The udp pcb is bound to a random local port if not already bound.
- See also:
- udp_disconnect()
Definition at line 981 of file lwip_udp.c.
void udp_disconnect | ( | struct udp_pcb * | pcb ) |
Disconnect a UDP PCB.
- Parameters:
-
pcb the udp pcb to disconnect.
Definition at line 1025 of file lwip_udp.c.
struct udp_pcb* udp_new | ( | void | ) | [read] |
Create a UDP PCB.
- Returns:
- The UDP PCB which was created. NULL if the PCB data structure could not be allocated.
- See also:
- udp_remove()
Definition at line 1103 of file lwip_udp.c.
struct udp_pcb* udp_new_ip_type | ( | u8_t | type ) | [read] |
Create a UDP PCB for specific IP type.
- Parameters:
-
type IP address type, see lwip_ip_addr_type definitions. If you want to listen to IPv4 and IPv6 (dual-stack) packets, supply IPADDR_TYPE_ANY as argument and bind to IP_ANY_TYPE.
- Returns:
- The UDP PCB which was created. NULL if the PCB data structure could not be allocated.
- See also:
- udp_remove()
Definition at line 1135 of file lwip_udp.c.
void udp_recv | ( | struct udp_pcb * | pcb, |
udp_recv_fn | recv, | ||
void * | recv_arg | ||
) |
Set a receive callback for a UDP PCB.
This callback will be called when receiving a datagram for the pcb.
- Parameters:
-
pcb the pcb for which to set the recv callback recv function pointer of the callback function recv_arg additional argument to pass to the callback function
Definition at line 1053 of file lwip_udp.c.
void udp_remove | ( | struct udp_pcb * | pcb ) |
Remove an UDP PCB.
- Parameters:
-
pcb UDP PCB to be removed. The PCB is removed from the list of UDP PCB's and the data structure is freed from memory.
- See also:
- udp_new()
Definition at line 1070 of file lwip_udp.c.
Send data using UDP.
- Parameters:
-
pcb UDP PCB used to send the data. p chain of pbuf's to be sent.
The datagram will be sent to the current remote_ip & remote_port stored in pcb. If the pcb is not bound to a port, it will automatically be bound to a random port.
- Returns:
- lwIP error code.
- ERR_OK. Successful. No error occurred.
- ERR_MEM. Out of memory.
- ERR_RTE. Could not find route to destination address.
- ERR_VAL. No PCB or PCB is dual-stack
- More errors could be returned by lower protocol layers.
- See also:
- udp_disconnect() udp_sendto()
Definition at line 455 of file lwip_udp.c.
Same as udp_send() but with checksum.
Definition at line 470 of file lwip_udp.c.
err_t udp_sendto | ( | struct udp_pcb * | pcb, |
struct pbuf * | p, | ||
const ip_addr_t * | dst_ip, | ||
u16_t | dst_port | ||
) |
Send data to a specified address using UDP.
- Parameters:
-
pcb UDP PCB used to send the data. p chain of pbuf's to be sent. dst_ip Destination IP address. dst_port Destination UDP port.
dst_ip & dst_port are expected to be in the same byte order as in the pcb.
If the PCB already has a remote address association, it will be restored after the data is sent.
- Returns:
- lwIP error code (
- See also:
- udp_send for possible error codes)
- udp_disconnect() udp_send()
Definition at line 502 of file lwip_udp.c.
err_t udp_sendto_chksum | ( | struct udp_pcb * | pcb, |
struct pbuf * | p, | ||
const ip_addr_t * | dst_ip, | ||
u16_t | dst_port, | ||
u8_t | have_chksum, | ||
u16_t | chksum | ||
) |
Same as udp_sendto(), but with checksum.
Definition at line 512 of file lwip_udp.c.
err_t udp_sendto_if | ( | struct udp_pcb * | pcb, |
struct pbuf * | p, | ||
const ip_addr_t * | dst_ip, | ||
u16_t | dst_port, | ||
struct netif * | netif | ||
) |
Send data to a specified address using UDP.
The netif used for sending can be specified.
This function exists mainly for DHCP, to be able to send UDP packets on a netif that is still down.
- Parameters:
-
pcb UDP PCB used to send the data. p chain of pbuf's to be sent. dst_ip Destination IP address. dst_port Destination UDP port. netif the netif used for sending.
dst_ip & dst_port are expected to be in the same byte order as in the pcb.
- Returns:
- lwIP error code (
- See also:
- udp_send for possible error codes)
- udp_disconnect() udp_send()
Definition at line 592 of file lwip_udp.c.
err_t udp_sendto_if_src | ( | struct udp_pcb * | pcb, |
struct pbuf * | p, | ||
const ip_addr_t * | dst_ip, | ||
u16_t | dst_port, | ||
struct netif * | netif, | ||
const ip_addr_t * | src_ip | ||
) |
Same as udp_sendto_if, but with source address.
Definition at line 661 of file lwip_udp.c.
Generated on Tue Jul 12 2022 14:49:33 by
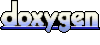