ADT7320 SPI High Resolution MBED Library
Embed:
(wiki syntax)
Show/hide line numbers
ADT7320_SPI.cpp
00001 #include "ADT7320_SPI.h" 00002 00003 //#include "mbed.h" 00004 00005 ADT7320_SPI::ADT7320_SPI(PinName mosi, PinName miso, PinName sclk, PinName cs) : spi_(mosi, miso, sclk),cs_(cs) { 00006 // Chip must be deselected 00007 cs_ = 1; 00008 // Setup the spi for 8 bit data, high steady state clock, 00009 // second edge capture, with a 1MHz clock rate 00010 spi_.format(8,3); 00011 spi_.frequency(1000000); 00012 } 00013 00014 //Read from register from the ADT7320: 00015 unsigned int ADT7320_SPI::readRegister(uint8_t thisRegister, int bytesToRead ) { 00016 uint8_t inByte = 0; // incoming byte from the SPI 00017 unsigned int result = 0; // result to return 00018 // take the chip select low to select the device: 00019 cs_=0; 00020 // send the device the register you want to read: 00021 spi_.write(thisRegister); 00022 // send a value of 0 to read the first byte returned: 00023 result = spi_.write(0xFF); 00024 // decrement the number of bytes left to read: 00025 bytesToRead--; 00026 // if you still have another byte to read: 00027 if (bytesToRead > 0) { 00028 // shift the first byte left, then get the second byte: 00029 result = result << 8; 00030 inByte = spi_.write(0xFF); 00031 // combine the byte you just got with the previous one: 00032 result = result | inByte; 00033 // decrement the number of bytes left to read: 00034 bytesToRead--; 00035 } 00036 // take the chip select high to de-select: 00037 cs_=1; 00038 // return the result: 00039 return(result); 00040 } 00041 00042 float ADT7320_SPI::readTemp(void) 00043 { 00044 //Read the temperature data 00045 unsigned int tempData = readRegister(0x50, 2); // 0x50 is read commad for 0x02 register 00046 tempData = tempData/8;// MSB bit15 and LSB bit4 so received value need to be divide/8 00047 00048 // convert the temperature to celsius and display it: 00049 float realTemp = (float)tempData * 0.0625; 00050 return realTemp; 00051 } 00052
Generated on Wed Jul 13 2022 15:37:33 by
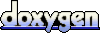